Java programming 1. Please find the maximum element in a Binary Search Tree? using java.util.Scanner;
Java
1. Please find the maximum element in a Binary Search Tree? using java.util.Scanner;

A Java program that finds the maximum element in the Binary Search Tree (BST) is as follows,
File name: “BST.java”
//Import the necessary package
import java.util.*;
//Create a class
class BST
{
//Create a binary node "Node"
static class Node
{
int data;
Node left;
Node right;
};
// Define the method to create a new node
static Node newNode(int data)
{
Node node = new Node();
node.data = data;
node.left = null;
node.right = null;
return (node);
}
// Define a method to insert a new node
static Node insert(Node node1, int key)
{
//Check whether the tree is empty
if (node1 == null)
//Return a new node
return (newNode(key));
else
{
//Otherwise down the tree recursively
if (key <= node1.data)
node1.left = insert(node1.left, key);
else
node1.right = insert(node1.right, key);
//Return the node pointer
return node1;
}
}
// Define a method to find the node with maximum value
static int maxValue(Node node)
{
//Loop down to search the rightmost leaf
Node current_node = node;
while (current_node.right != null)
current_node = current_node.right;
return (current_node.data);
}
// Define the main() method
public static void main(String[] args)
{
//create Scanner class
Scanner in=new Scanner(System.in);
Node aRoot = null;
System.out.print("Enter the elements to be inserted in BST:");
//Assign the value returned from the method insert()
aRoot = insert(aRoot, in.nextInt());
insert(aRoot, in.nextInt());
insert(aRoot, in.nextInt());
insert(aRoot, in.nextInt());
insert(aRoot, in.nextInt());
insert(aRoot, in.nextInt());
//Print the output
System.out.println("The maximum element in BST is: " + maxValue(aRoot));
}
}
Screenshot #1:
Screenshot #2:
Screenshot #3:
Screenshot #4:
Step by step
Solved in 3 steps with 5 images

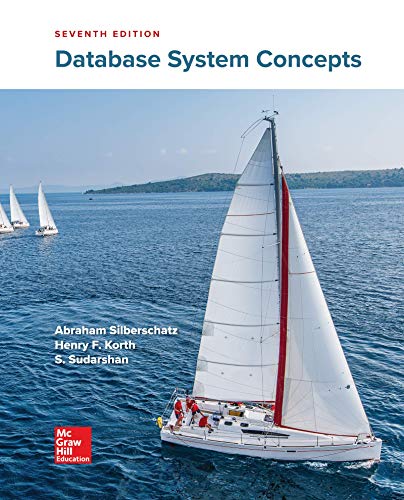
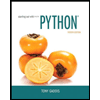
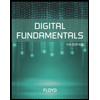
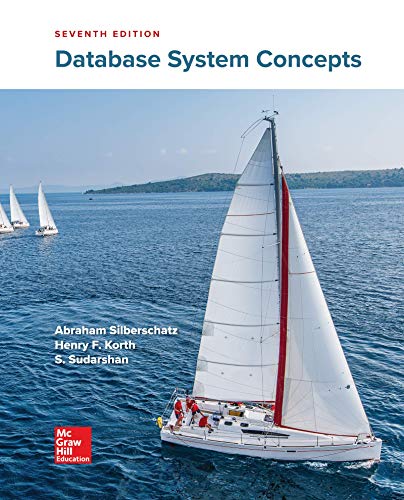
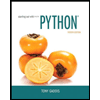
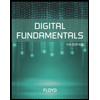
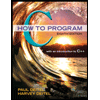
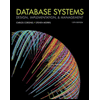
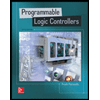