JAVA PROGRAM Introduction The goal of this project is to develop a nontrivial computer program in the Java language by applying elementary programming language concepts learned so far. Specifically, the concepts to be used for this project include simple input/output, selection, iteration (loops), and possibly one-dimensional arrays (although the use of arrays is not required for completing this project). Developing a Java program for printing a calendar Statement of Problem: Develop a Java program to print a calendar for the year that the user will provide as an input to the program. Use the Eclipse IDE (on a Windows or Mac machine). Your program must fulfill the following requirements: Your program should prompt and receive as input an integer value representing the year for which the user wishes to have a calendar printed. This integer must be in the range from 1780 through 2040. In case the user input a year that is outside this range, your program must print out a message such as "Invalid year. Please input an integer in the range 1780 through 2040." The program must do this repeatedly if necessary until the user provides an acceptable input. Each month of the year must be printed out with proper labels for the weekdays and the dates aligned properly under the appropriate labels. For your reference, please see the sample output below for the month of May 2019: Your program must print out all 12 months of the year specified. Each month must be printed directly below the previous month. The deliverable for this task is a plain text document named MyCalendar.java that contains the source code of your program. This document must be submitted on Canvas. Java Program for Printing a Calendar: Analysis and Design Creating a working program is an iterative process. You may have to repeatedly make changes in your source code for removing syntax errors, to begin with. Then you may need to make changes that will address any logical errors that you detect based on the results you obtain. Thus, I would recommend developing your code incrementally. Incremental development would mean writing a few lines of code at a time to complete a simple task that forms part of your final solution and running the program to make sure that those lines of code work. This way, if at any time you add something new an error pops up, you will be able to focus your attention on the latest addition in your code. In summary, you will be dividing the given problem into sub-problems first and solve the sub-problems one by one. This requires carrying out a careful analysis of the problem, followed by an appropriate design formulation for your solution. For this project, the following hints will help in the analysis of the problem so that you are able to appreciate the resulting design and build your code. Another way to look at this is that the analysis will provide an idea of how you may split your task of creating the program into several sub-tasks. Writing code that will help complete each of these sub-tasks in order will amount to incrementally developing the required program. From the sample month shown, we need the following information about each month in order to print it properly. The day of the week on which the first day of the month falls; and The number of days in the month. The number of days in each month is a known quantity, independent of the year, except for February. February has either 28 days or 29 days, depending on whether the given year is a leap year or not Suppose the year user input is 2016. Then, we need to first figure out on which day of the week did January 1, 2016 fall. Using a formula which we will provide later, we can figure out that January 1, 2016 was a Friday. The above information, coupled with the fact that the month of January has 31 days, will help us figure out that February 1, 2016 was a Monday. Since 2016 is a leap year, the month of February had 29 days. This leads us to the correct conclusion that March 1, 2016 was a Tuesday. The above discussion and the statement of the problem at the beginning have already helped us understand that the following sub-problems need to be handled in the Java code: Prompt the user and receive an input integer representing the year. If the year entered is not in the range from 1780 through 2040, print out a message requesting the user to enter a year in the permissible range. Repeat this until the user inputs an acceptable year. Figure out the day of the week for January 1 of
JAVA PROGRAM
Introduction
The goal of this project is to develop a nontrivial computer program in the Java language by applying elementary programming language concepts learned so far. Specifically, the concepts to be used for this project include simple input/output, selection, iteration (loops), and possibly one-dimensional arrays (although the use of arrays is not required for completing this project).
Developing a Java program for printing a calendar
Statement of Problem: Develop a Java program to print a calendar for the year that the user will provide as an input to the program. Use the Eclipse IDE (on a Windows or Mac machine). Your program must fulfill the following requirements:
- Your program should prompt and receive as input an integer value representing the year for which the user wishes to have a calendar printed. This integer must be in the range from 1780 through 2040.
- In case the user input a year that is outside this range, your program must print out a message such as "Invalid year. Please input an integer in the range 1780 through 2040." The program must do this repeatedly if necessary until the user provides an acceptable input.
- Each month of the year must be printed out with proper labels for the weekdays and the dates aligned properly under the appropriate labels. For your reference, please see the sample output below for the month of May 2019:
- Your program must print out all 12 months of the year specified. Each month must be printed directly below the previous month.
- The deliverable for this task is a plain text document named MyCalendar.java that contains the source code of your program. This document must be submitted on Canvas.
Java Program for Printing a Calendar: Analysis and Design
Creating a working program is an iterative process. You may have to repeatedly make changes in your source code for removing syntax errors, to begin with. Then you may need to make changes that will address any logical errors that you detect based on the results you obtain. Thus, I would recommend developing your code incrementally. Incremental development would mean writing a few lines of code at a time to complete a simple task that forms part of your final solution and running the program to make sure that those lines of code work. This way, if at any time you add something new an error pops up, you will be able to focus your attention on the latest addition in your code.
In summary, you will be dividing the given problem into sub-problems first and solve the sub-problems one by one. This requires carrying out a careful analysis of the problem, followed by an appropriate design formulation for your solution.
For this project, the following hints will help in the analysis of the problem so that you are able to appreciate the resulting design and build your code. Another way to look at this is that the analysis will provide an idea of how you may split your task of creating the program into several sub-tasks. Writing code that will help complete each of these sub-tasks in order will amount to incrementally developing the required program.
- From the sample month shown, we need the following information about each month in order to print it properly.
- The day of the week on which the first day of the month falls; and
- The number of days in the month.
- The number of days in each month is a known quantity, independent of the year, except for February. February has either 28 days or 29 days, depending on whether the given year is a leap year or not
- Suppose the year user input is 2016. Then, we need to first figure out on which day of the week did January 1, 2016 fall. Using a formula which we will provide later, we can figure out that January 1, 2016 was a Friday.
- The above information, coupled with the fact that the month of January has 31 days, will help us figure out that February 1, 2016 was a Monday. Since 2016 is a leap year, the month of February had 29 days. This leads us to the correct conclusion that March 1, 2016 was a Tuesday.
- The above discussion and the statement of the problem at the beginning have already helped us understand that the following sub-problems need to be handled in the Java code:
- Prompt the user and receive an input integer representing the year. If the year entered is not in the range from 1780 through 2040, print out a message requesting the user to enter a year in the permissible range. Repeat this until the user inputs an acceptable year.
- Figure out the day of the week for January 1 of

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

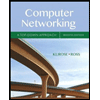
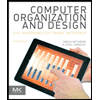
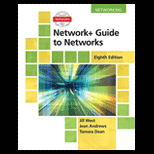
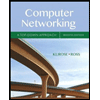
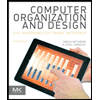
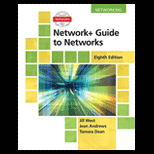
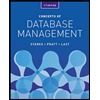
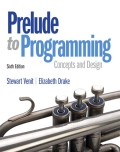
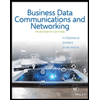