WORD FREQUENCIES Input Standard Input Output Standard Output Data Structure List Problem Description Write a JAVA program that will display all words from a given passage with its frequencies. At the end of output, print the text analysis such as (i) Total words, (ii) Number of Repeated words, (iii) Number of Unique words and (iv) Most used word. Input Input of this program is a passage. A passage consists of N words and symbols. Symbols that will be considered in the passage are full stop (.), comma (,), question mark (?) and exclamation mark (!). The passage will have M unique words, where the M is less than or equal to N. Output Output of the program is M lines, where each line contains a word followed by symbol (, then followed by an integer that represent the word frequency and ends by symbol). Display the analysis of Total words, Number of repeated words, Number of unique words and Most used word as shown in Sample InputOutput. Sample Input-Output Input Output I go to school by bus. The bus is big. The school is also big. I like big school and big bus. I(2) The(2) also(1) and(1) big(4) bus(3) by(1) go(1) is(2) like(1) school(3) to(1) Total words: 22 Number of repeated words: 6 Number of unique words: 12 Most used word: big Solution The algorithm for Words Frequencies: Read a passage For each word in the passage: Search for the same word in the list If not found: Add the word into the list Set and add the word’s frequency to 1 If found: Update the word’s frequency value in list Sort the list Display the list and text analysis Class Data ** You must use class Data to complete the question 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 import public } java.util.Comparator; // Class Data class Data { String word; int freq; public Data (String item){ this.word =item; this.freq=1; } public String getWord() { return word; } public void setWord(String newword) { this.word= newword; } public int getFreq() { return freq; } public void setFreq(int freq2) { this.freq = freq2; } public static Comparator WordComparator = new Comparator() { // Used for sorting in ascending order of word public int compare(Data a, Data b) { String word1 = a.getWord(); String word2 = b.getWord(); return (word1).compareTo(word2); //string1.compareTo(string2) } };
WORD FREQUENCIES |
|
Input |
Standard Input |
Output |
Standard Output |
Data Structure |
List |
Problem Description
Write a JAVA program that will display all words from a given passage with its frequencies. At the end of output, print the text analysis such as (i) Total words, (ii) Number of Repeated words, (iii) Number of Unique words and (iv) Most used word.
Input
Input of this program is a passage. A passage consists of N words and symbols. Symbols that will be considered in the passage are full stop (.), comma (,), question mark (?) and exclamation mark (!).
The passage will have M unique words, where the M is less than or equal to N.
Output
Output of the program is M lines, where each line contains a word followed by symbol (, then followed by an integer that represent the word frequency and ends by symbol). Display the analysis of Total words, Number of repeated words, Number of unique words and Most used word as shown in Sample InputOutput.
Sample Input-Output
Input |
Output |
I go to school by bus. The bus is big. The school is also big. I like big school and big bus.
|
I(2) The(2) also(1) and(1) big(4) bus(3) by(1) go(1) is(2) like(1) school(3) to(1)
|
|
Total words: 22 Number of repeated words: 6 Number of unique words: 12 Most used word: big
|
Solution
The
Read a passage For each word in the passage:
Search for the same word in the list If not found: Add the word into the list Set and add the word’s frequency to 1 If found: Update the word’s frequency value in list |
Sort the list
Display the list and text analysis
Class Data
** You must use class Data to complete the question
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
import public
} |
java.util.Comparator; // Class Data class Data { String word; int freq; public Data (String item){ this.word =item; this.freq=1; } public String getWord() { return word; } public void setWord(String newword) { this.word= newword; } public int getFreq() { return freq; } public void setFreq(int freq2) { this.freq = freq2; } public static Comparator<Data> WordComparator = new Comparator<Data>() { // Used for sorting in ascending order of word public int compare(Data a, Data b) { String word1 = a.getWord(); String word2 = b.getWord();
return (word1).compareTo(word2); //string1.compareTo(string2) } }; |

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

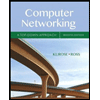
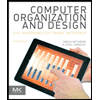
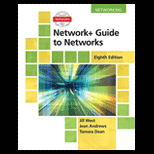
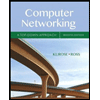
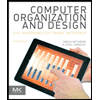
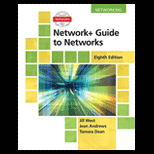
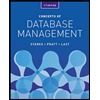
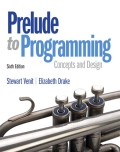
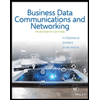