Java Program ASAP ************This program must work in hypergrade and pass all the test cases.********** Remove the extra space \n from the program and dont take out quit from the program because it requires a space for it as shown in the screenshot. The text files are located in Hypergrade. For test case 1 first display Please enter a string to convert to Morse code:\n then you press Enter it should print out \n. Then for test case 2 it should display Please enter a string to convert to Morse code:\n then you type abc it should print out .- -... -.-. \n For test case 3 first display Please enter a string to convert to Morse code:\n then you type This is a sample string 1234.ENTER it should print put - .... .. ... .. ... .- ... .- -- .--. .-.. . ... - .-. .. -. --. .---- ..--- ...-- ....- .-.-.- \n. This program down below does not pass the test cases as shown in the screenshot I have provided the correct test case as a screenshot too. Please modify it or create a new program so it paases the test cases. Thank you! For test case 1 it wants only Please enter a string to convert to Morse code:\n ENTER \n For test case 2 it wants only Please enter a string to convert to Morse code:\n abcENTER .- -... -.-. \n For test case 3 it wants only Please enter a string to convert to Morse code:\n This is a sample string 1234.ENTER - .... .. ... .. ... .- ... .- -- .--. .-.. . ... - .-. .. -. --. .---- ..--- ...-- ....- .-.-.- \n import java.util.HashMap; import java.util.Scanner; public class MorseEncoder { private static final HashMap codeMappings = new HashMap<>(); public static void main(String[] args) { initializeMappings(); Scanner textScanner = new Scanner(System.in); System.out.print("Please enter a string to convert to Morse code:\n"); String textForEncoding = textScanner.nextLine(); if ("ENTER".equals(textForEncoding)) { System.out.println(); return; } String encodedOutput = encodeText(textForEncoding); System.out.println(encodedOutput); } private static void initializeMappings() { codeMappings.put('A', ".-"); codeMappings.put('B', "-..."); codeMappings.put('C', "-.-."); codeMappings.put('D', "-.."); codeMappings.put('E', "."); codeMappings.put('F', "..-."); codeMappings.put('G', "--."); codeMappings.put('H', "...."); codeMappings.put('I', ".."); codeMappings.put('J', ".---"); codeMappings.put('K', "-.-"); codeMappings.put('L', ".-.."); codeMappings.put('M', "--"); codeMappings.put('N', "-."); codeMappings.put('O', "---"); codeMappings.put('P', ".--."); codeMappings.put('Q', "--.-"); codeMappings.put('R', ".-."); codeMappings.put('S', "..."); codeMappings.put('T', "-"); codeMappings.put('U', "..-"); codeMappings.put('V', "...-"); codeMappings.put('W', ".--"); codeMappings.put('X', "-..-"); codeMappings.put('Y', "-.--"); codeMappings.put('Z', "--.."); } private static String encodeText(String textForEncoding) { StringBuilder encodedStringBuilder = new StringBuilder(); boolean lastCharWasSpace = false; for (char individualChar : textForEncoding.toCharArray()) { if (individualChar == ' ') { if (!lastCharWasSpace) { encodedStringBuilder.append(" "); lastCharWasSpace = true; } continue; } lastCharWasSpace = false; String morseSymbol = codeMappings.get(Character.toUpperCase(individualChar)); if (morseSymbol != null) { encodedStringBuilder.append(morseSymbol).append(' '); } } return encodedStringBuilder.toString().trim(); } } morse.txt 0 ----- 1 .---- 2 ..--- 3 ...-- 4 ....- 5 ..... 6 -.... 7 --... 8 ---.. 9 ----. , --..-- . .-.-.- ? ..--.. A .- B -... C -.-. D -.. E . F ..-. G --. H .... I .. J .--- K -.- L .-.. M -- N -. O --- P .--. Q --.- R .-. S ... T - U ..- V ...- W .-- X -..- Y -.-- Z --..
ENTER
\n
abcENTER
.- -... -.-. \n
This is a sample string 1234.ENTER
- .... .. ... .. ... .- ... .- -- .--. .-.. . ... - .-. .. -. --. .---- ..--- ...-- ....- .-.-.- \n
import java.util.HashMap;
import java.util.Scanner;
public class MorseEncoder {
private static final HashMap<Character, String> codeMappings = new HashMap<>();
public static void main(String[] args) {
initializeMappings();
Scanner textScanner = new Scanner(System.in);
System.out.print("Please enter a string to convert to Morse code:\n");
String textForEncoding = textScanner.nextLine();
if ("ENTER".equals(textForEncoding)) {
System.out.println();
return;
}
String encodedOutput = encodeText(textForEncoding);
System.out.println(encodedOutput);
}
private static void initializeMappings() {
codeMappings.put('A', ".-");
codeMappings.put('B', "-...");
codeMappings.put('C', "-.-.");
codeMappings.put('D', "-..");
codeMappings.put('E', ".");
codeMappings.put('F', "..-.");
codeMappings.put('G', "--.");
codeMappings.put('H', "....");
codeMappings.put('I', "..");
codeMappings.put('J', ".---");
codeMappings.put('K', "-.-");
codeMappings.put('L', ".-..");
codeMappings.put('M', "--");
codeMappings.put('N', "-.");
codeMappings.put('O', "---");
codeMappings.put('P', ".--.");
codeMappings.put('Q', "--.-");
codeMappings.put('R', ".-.");
codeMappings.put('S', "...");
codeMappings.put('T', "-");
codeMappings.put('U', "..-");
codeMappings.put('V', "...-");
codeMappings.put('W', ".--");
codeMappings.put('X', "-..-");
codeMappings.put('Y', "-.--");
codeMappings.put('Z', "--..");
}
private static String encodeText(String textForEncoding) {
StringBuilder encodedStringBuilder = new StringBuilder();
boolean lastCharWasSpace = false;
for (char individualChar : textForEncoding.toCharArray()) {
if (individualChar == ' ') {
if (!lastCharWasSpace) {
encodedStringBuilder.append(" ");
lastCharWasSpace = true;
}
continue;
}
lastCharWasSpace = false;
String morseSymbol = codeMappings.get(Character.toUpperCase(individualChar));
if (morseSymbol != null) {
encodedStringBuilder.append(morseSymbol).append(' ');
}
}
return encodedStringBuilder.toString().trim();
}
}
0 -----
1 .----
2 ..---
3 ...--
4 ....-
5 .....
6 -....
7 --...
8 ---..
9 ----.
, --..--
. .-.-.-
? ..--..
A .-
B -...
C -.-.
D -..
E .
F ..-.
G --.
H ....
I ..
J .---
K -.-
L .-..
M --
N -.
O ---
P .--.
Q --.-
R .-.
S ...
T -
U ..-
V ...-
W .--
X -..-
Y -.--
Z --..



Step by step
Solved in 4 steps with 3 images

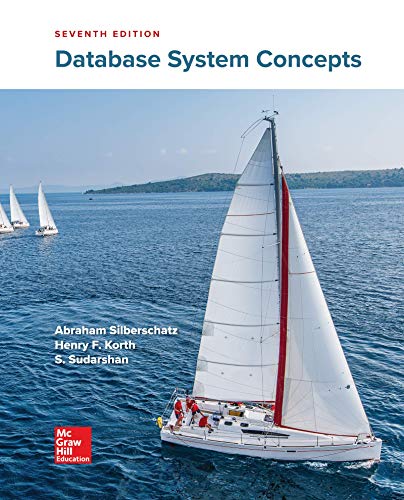
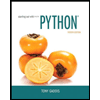
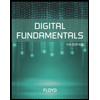
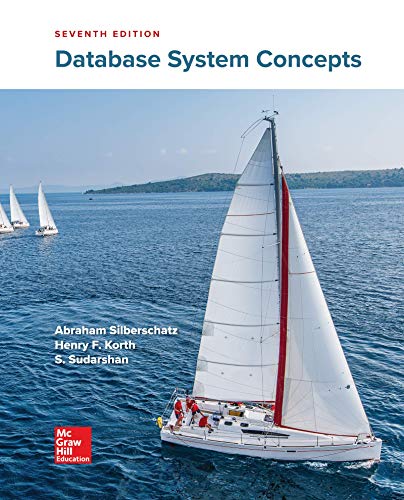
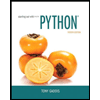
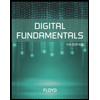
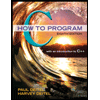
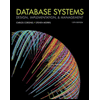
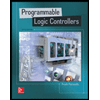