JAVA PLEASE Starting with your solution to the previous task, add the following methods to your ScaleneTriangle class to extend its functionality. A default (or "no-argument" or "no-arg") constructor that constructs a Triangle object using default values. An overloaded constructor that constructs a Triangle object with the given values. A method named getArea() which returns the area of a triangle. This method returns a double. The are is calculated by: √(s * (s - sideA) * (s - sideB) * (s - sideC)) where s = (sideA + sideB + sideC) / 2 Write the ScaleneTriangle class in the answer box below assuming that the superclass is given. For example:
JAVA PLEASE
Starting with your solution to the previous task, add the following methods to your ScaleneTriangle class to extend its functionality.
- A default (or "no-argument" or "no-arg") constructor that constructs a Triangle object using default values.
- An overloaded constructor that constructs a Triangle object with the given values.
- A method named getArea() which returns the area of a triangle. This method returns a double. The are is calculated by: √(s * (s - sideA) * (s - sideB) * (s - sideC)) where s = (sideA + sideB + sideC) / 2
Write the ScaleneTriangle class in the answer box below assuming that the superclass is given.
For example:
abstract class Triangle {
protected double sideA;
protected double sideB;
protected double sideC;
public Triangle() {
sideA = sideB = sideC = 10;
}
public Triangle(double sideA, double sideB, double sideC) {
this.sideA = sideA;
this.sideB = sideB;
this.sideC = sideC;
}
public double getSideA() {
return sideA;
}
public double getSideB() {
return sideB;
}
public double getSideC() {
return sideC;
}
public abstract double getArea();
public double getPerimeter() {
return sideA + sideB + sideC;
}
public String toString() {
return
String.format("%s:(%.2f, %.2f, %.2f)",
getClass().getName(), sideA, sideB, sideC);
}
}


Step by step
Solved in 4 steps with 3 images

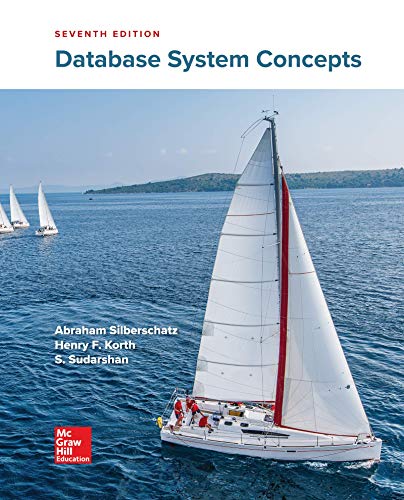
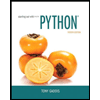
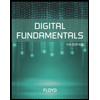
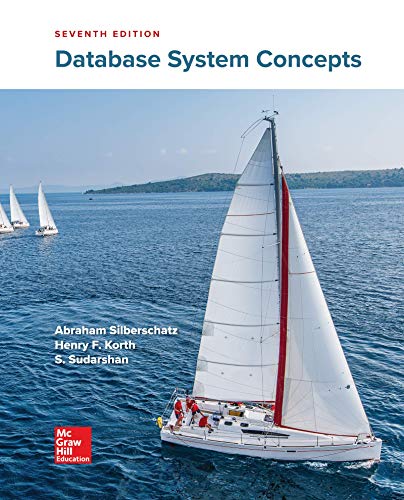
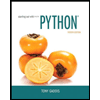
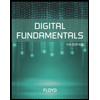
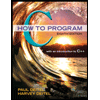
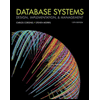
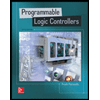