JAVA - How do I make this a random pull of participants instead of just a participant +1? I want the purchaser to be the same as it was input in the array, but then want the gift to be random. If that isn't possible, making both random would work as well, but either way the giver and receiver can't be the same person.
JAVA - How do I make this a random pull of participants instead of just a participant +1?
I want the purchaser to be the same as it was input in the array, but then want the gift to be random. If that isn't possible, making both random would work as well, but either way the giver and receiver can't be the same person.
The output should be something like this:
a will purchase a gift for d (age: 2)
b will purchase a gift for c (age: 3)
c will purchase a gift for a (age: 4)
d will purchase a gift for b (age: 2)
import java.util.Random;
public class ExchangeResults
{
public static void main(String[] args)
{
UserInput userinput = new UserInput();
Participant[] participants = userinput.inputParticipants();
System.out.println("Exchange Results");
//PRINT MATCHES
shuffleParticipants(participants);
}
//MAKE RANDOM MATCHES
public static void shuffleParticipants(Participant[] participants)
{
for (int i = 0; i < participants.length-1 ; i++)
{
Participant p1 = participants[i];
Participant p2 = participants[i+1];
System.out.printf("%s will purchase a gift for %s (age: %d)\n",p1.getName(),p2.getName(),p2.getAge());
}
Participant pl = participants[participants.length-1];
Participant p0 = participants[0];
System.out.printf("%s will purchase a gift for %s (age: %d)\n", pl.getName(), p0.getName(), p0.getAge());
}
}

Step by step
Solved in 2 steps

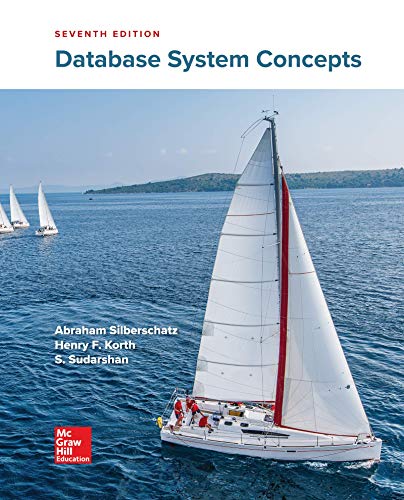
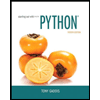
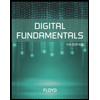
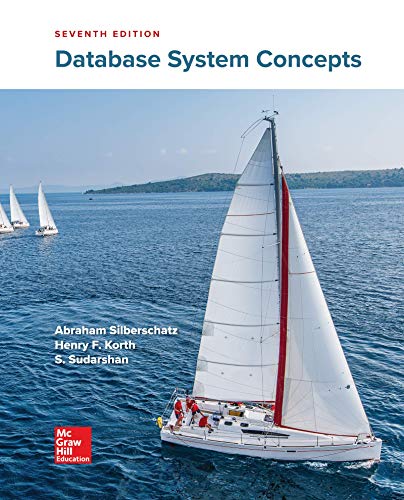
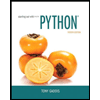
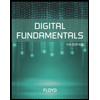
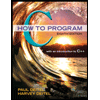
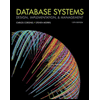
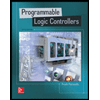