(Java) DO PART 2! PART 2 IS BASED OFF PART 1!!! PART 1: Open up a new Java project called Activity6 and create a class called Person Inside of the class, define three attributes that a person has as variables of the class Name Age Gender Next, define two actions that can be performed as methods of the class: The method is named greeting It takes no parameters It prints out the message "Hi, my name is " + name + "!" It returns nothing The method is named addYear It takes in no parameters It adds one year to the person's age It returns nothing Then, create a new Java file called PersonTest.java. Copy and paste the below test code into PersonTest.java: /** * @author * CIS 36B, Activity 6.1 */ public class PersonTest { public static void main(String[] args) { Person wen = new Person(); wen.name = "Wen"; wen.age = 19; wen.gender = "male"; wen.greeting(); wen.addYear(); System.out.println("I just turned " + wen.age + " years old."); } } Now, run the program and verify that you get the following output: Hi, my name is Wen! I just turned 20 years old. Part 2: Open up your Person class from the last activity. Add a second method to this class. Here are the specifications for this method: The method is named greeting It takes in one String parameter for another person's name It prints out the message "Hi, " + otherName + "!" It returns nothing. Add a final method to this class. The method is named printPerson It prints a Person's name, age and gender to the console, in the format Name: Age: Gender: It returns nothing Copy and paste the new test file below into PersonTest.java (you can erase the old contents of the file) Add the missing 4 lines of code. Then run your code to verify you have the correct output (shown below): /** * @author * CIS 36B, Activity 6.2 */ import java.util.Scanner; import java.util.ArrayList; public class PersonTest { public static void main(String[] args) { Scanner input = new Scanner(System.in); Person wen = new Person(); wen.name = "Wen"; wen.age = 19; wen.gender = "male"; wen.greeting(); System.out.print("\nWhat's your name: "); String name = input.nextLine(); //call greeting method passing in name parameter System.out.print("\nHow old are you: "); int age = input.nextInt(); System.out.print("What is your gender: "); String gender = input.next(); //make a new Person here and assign that Person the name, age and gender read from console ArrayList pair = new ArrayList(2); pair.add(wen); //add second person to ArrayList here System.out.println("\nPair Programming Partners: "); for (int i = 0; i < pair.size(); i++) { //add a line of code to call printPerson on each person in ArrayList } } } Now, run the program and verify that you get the new output (given the below input): Hi, my name is Wen! What's your name: Maria Hi, Maria! How old are you: 20 What is your gender: female Pair Programming Partners: Name: Wen Age: 19 Gender: male Name: Maria Age: 20 Gender: female When you are getting the correct output, submit your Person.java *and* PersonTest.java
(Java) DO PART 2! PART 2 IS BASED OFF PART 1!!! PART 1: Open up a new Java project called Activity6 and create a class called Person Inside of the class, define three attributes that a person has as variables of the class Name Age Gender Next, define two actions that can be performed as methods of the class: The method is named greeting It takes no parameters It prints out the message "Hi, my name is " + name + "!" It returns nothing The method is named addYear It takes in no parameters It adds one year to the person's age It returns nothing Then, create a new Java file called PersonTest.java. Copy and paste the below test code into PersonTest.java: /** * @author * CIS 36B, Activity 6.1 */ public class PersonTest { public static void main(String[] args) { Person wen = new Person(); wen.name = "Wen"; wen.age = 19; wen.gender = "male"; wen.greeting(); wen.addYear(); System.out.println("I just turned " + wen.age + " years old."); } } Now, run the program and verify that you get the following output: Hi, my name is Wen! I just turned 20 years old. Part 2: Open up your Person class from the last activity. Add a second method to this class. Here are the specifications for this method: The method is named greeting It takes in one String parameter for another person's name It prints out the message "Hi, " + otherName + "!" It returns nothing. Add a final method to this class. The method is named printPerson It prints a Person's name, age and gender to the console, in the format Name: Age: Gender: It returns nothing Copy and paste the new test file below into PersonTest.java (you can erase the old contents of the file) Add the missing 4 lines of code. Then run your code to verify you have the correct output (shown below): /** * @author * CIS 36B, Activity 6.2 */ import java.util.Scanner; import java.util.ArrayList; public class PersonTest { public static void main(String[] args) { Scanner input = new Scanner(System.in); Person wen = new Person(); wen.name = "Wen"; wen.age = 19; wen.gender = "male"; wen.greeting(); System.out.print("\nWhat's your name: "); String name = input.nextLine(); //call greeting method passing in name parameter System.out.print("\nHow old are you: "); int age = input.nextInt(); System.out.print("What is your gender: "); String gender = input.next(); //make a new Person here and assign that Person the name, age and gender read from console ArrayList pair = new ArrayList(2); pair.add(wen); //add second person to ArrayList here System.out.println("\nPair Programming Partners: "); for (int i = 0; i < pair.size(); i++) { //add a line of code to call printPerson on each person in ArrayList } } } Now, run the program and verify that you get the new output (given the below input): Hi, my name is Wen! What's your name: Maria Hi, Maria! How old are you: 20 What is your gender: female Pair Programming Partners: Name: Wen Age: 19 Gender: male Name: Maria Age: 20 Gender: female When you are getting the correct output, submit your Person.java *and* PersonTest.java
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
(Java)
DO PART 2! PART 2 IS BASED OFF PART 1!!!
PART 1:
- Open up a new Java project called Activity6 and create a class called Person
- Inside of the class, define three attributes that a person has as variables of the class
- Name
- Age
- Gender
- Next, define two actions that can be performed as methods of the class:
- The method is named greeting
- It takes no parameters
- It prints out the message "Hi, my name is " + name + "!"
- It returns nothing
- The method is named addYear
- It takes in no parameters
- It adds one year to the person's age
- It returns nothing
- Then, create a new Java file called PersonTest.java.
- Copy and paste the below test code into PersonTest.java:
/**
* @author
* CIS 36B, Activity 6.1
*/
public class PersonTest {
public static void main(String[] args) {
Person wen = new Person();
wen.name = "Wen";
wen.age = 19;
wen.gender = "male";
wen.greeting();
wen.addYear();
System.out.println("I just turned " + wen.age + " years old.");
}
}
- Now, run the program and verify that you get the following output:
Hi, my name is Wen!
I just turned 20 years old.
Part 2:
- Open up your Person class from the last activity.
- Add a second method to this class. Here are the specifications for this method:
- The method is named greeting
- It takes in one String parameter for another person's name
- It prints out the message "Hi, " + otherName + "!"
- It returns nothing.
- Add a final method to this class.
- The method is named printPerson
- It prints a Person's name, age and gender to the console, in the format
Name: <name>
Age: <age>
Gender: <gender>
- It returns nothing
- Copy and paste the new test file below into PersonTest.java (you can erase the old contents of the file)
- Add the missing 4 lines of code.
- Then run your code to verify you have the correct output (shown below):
* @author
* CIS 36B, Activity 6.2
*/
import java.util.Scanner;
import java.util.ArrayList;
public class PersonTest {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
Person wen = new Person();
wen.name = "Wen";
wen.age = 19;
wen.gender = "male";
wen.greeting();
System.out.print("\nWhat's your name: ");
String name = input.nextLine();
//call greeting method passing in name parameter
System.out.print("\nHow old are you: ");
int age = input.nextInt();
System.out.print("What is your gender: ");
String gender = input.next();
//make a new Person here and assign that Person the name, age and gender read from console
ArrayList<Person> pair = new ArrayList<Person>(2);
pair.add(wen);
//add second person to ArrayList here
System.out.println("\nPair
for (int i = 0; i < pair.size(); i++) {
//add a line of code to call printPerson on each person in ArrayList
}
}
}
- Now, run the program and verify that you get the new output (given the below input):
What's your name: Maria
Hi, Maria!
How old are you: 20
What is your gender: female
Pair Programming Partners:
Name: Wen
Age: 19
Gender: male
Name: Maria
Age: 20
Gender: female
- When you are getting the correct output, submit your Person.java *and* PersonTest.java
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
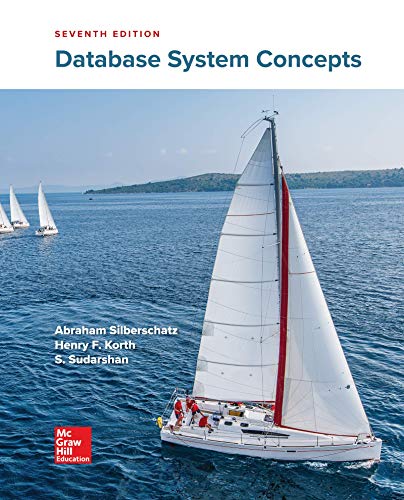
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
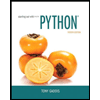
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
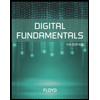
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
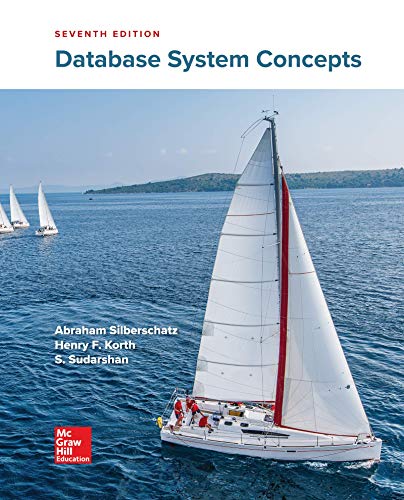
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
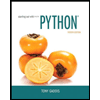
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
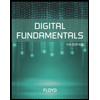
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
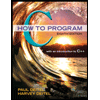
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
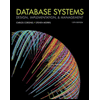
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
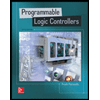
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education