Introduction This particular program implements a game called "Checkers" that is played in real life. For this assignment, you should try to make your program look as nice as possible using the provided code fragment. Problem statement: Write a C++ program that plays the game of Checkers. The game is simple and you can view the instruction video online: https://www.youtube.com/watch?y=m0drB0cx8pQ You can also find a simulation if you want to see the game in action. One specific online simulation is at: http://www.csgnetwork.com/checkers.html Set Up: • Two players, one uses "x's, the other uses "o's. • Checkers may be played on an 8x8, 10x10 or 12x12 square board. • Command line arguments will be used to indicate the size of the board, for example: . /checkers 8 • No matter the size of the board, pieces are placed on the dark squares of the board, leaving the middle two rows blank. For example: How to Play (Game rules): • Players cannot move an opponent's piece. • Turns alternate between players until the game is won. • The player who runs out of pieces first or cannot make a valid move loses the game. • Pieces may only move diagonally into unoccupied squares and only by one square if they are not capturing a piece. • piece may capture opponent's pieces by jumping over them diagonally. So long as there are successive pieces to be captured, the piece may continue moving. The line the piece travels does not have to be straight along one diagonal, it may change directions so long as it continues to progress the board and capture pieces. When a piece has reached the King's Row (furthest row from it's originating side) then it is "kinged" by placing a second token on top of it (You may use "X's or "O's or any other distinguishable symbols to indicate "kinged" pieces). This piece is now permitted to move backwards on the board and allowed to capture backward.
Introduction This particular program implements a game called "Checkers" that is played in real life. For this assignment, you should try to make your program look as nice as possible using the provided code fragment. Problem statement: Write a C++ program that plays the game of Checkers. The game is simple and you can view the instruction video online: https://www.youtube.com/watch?y=m0drB0cx8pQ You can also find a simulation if you want to see the game in action. One specific online simulation is at: http://www.csgnetwork.com/checkers.html Set Up: • Two players, one uses "x's, the other uses "o's. • Checkers may be played on an 8x8, 10x10 or 12x12 square board. • Command line arguments will be used to indicate the size of the board, for example: . /checkers 8 • No matter the size of the board, pieces are placed on the dark squares of the board, leaving the middle two rows blank. For example: How to Play (Game rules): • Players cannot move an opponent's piece. • Turns alternate between players until the game is won. • The player who runs out of pieces first or cannot make a valid move loses the game. • Pieces may only move diagonally into unoccupied squares and only by one square if they are not capturing a piece. • piece may capture opponent's pieces by jumping over them diagonally. So long as there are successive pieces to be captured, the piece may continue moving. The line the piece travels does not have to be straight along one diagonal, it may change directions so long as it continues to progress the board and capture pieces. When a piece has reached the King's Row (furthest row from it's originating side) then it is "kinged" by placing a second token on top of it (You may use "X's or "O's or any other distinguishable symbols to indicate "kinged" pieces). This piece is now permitted to move backwards on the board and allowed to capture backward.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Question
.
You can also find a simulation if you want to see the game in action. One specific online simulation is at: [Checkers Simulation](http://www.csgnetwork.com/checkers.html)
### Set Up
- Two players, one uses "x's", the other uses "o's".
- Checkers may be played on an 8x8, 10x10, or 12x12 square board.
- Command line arguments will be used to indicate the size of the board, for example: `./checkers 8`
- No matter the size of the board, pieces are placed on the dark squares of the board, leaving the middle two rows blank. For example:
- **8x8 Board Setup**:

- **10x10 Board Setup**:

- **12x12 Board Setup**:

### How to Play (Game rules)
- Players cannot move an opponent’s piece.
- Turns alternate between players until the game is won.
- The player who runs out of pieces first or cannot make a valid move loses the game.
- Pieces may only move diagonally into unoccupied squares and only by one square if they are not capturing a piece.
- A piece may capture an opponent’s pieces by jumping over them diagonally. As long as there are successive pieces to be captured, the piece may continue moving. The line the piece travels does not have to be straight along one diagonal, it may change directions so long as it continues to progress the board and capture pieces.
- When a piece has reached the King’s Row (furthest row from its original side) then it is “kinged” by placing a second token on top](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8759b09c-a99c-4616-917a-1eb84cf5071d%2Fa4616e1b-0eb0-4271-8307-bf054da6b603%2Fw8npyr1_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Introduction
This particular program implements a game called "Checkers" that is played in real life. For this assignment, you should try to make your program look as nice as possible using the provided code fragment.
### Problem statement
Write a C++ program that plays the game of Checkers. The game is simple and you can view the instruction video online: [Checkers Instruction Video](https://www.youtube.com/watch?v=m0dR0cx8pQ).
You can also find a simulation if you want to see the game in action. One specific online simulation is at: [Checkers Simulation](http://www.csgnetwork.com/checkers.html)
### Set Up
- Two players, one uses "x's", the other uses "o's".
- Checkers may be played on an 8x8, 10x10, or 12x12 square board.
- Command line arguments will be used to indicate the size of the board, for example: `./checkers 8`
- No matter the size of the board, pieces are placed on the dark squares of the board, leaving the middle two rows blank. For example:
- **8x8 Board Setup**:

- **10x10 Board Setup**:

- **12x12 Board Setup**:

### How to Play (Game rules)
- Players cannot move an opponent’s piece.
- Turns alternate between players until the game is won.
- The player who runs out of pieces first or cannot make a valid move loses the game.
- Pieces may only move diagonally into unoccupied squares and only by one square if they are not capturing a piece.
- A piece may capture an opponent’s pieces by jumping over them diagonally. As long as there are successive pieces to be captured, the piece may continue moving. The line the piece travels does not have to be straight along one diagonal, it may change directions so long as it continues to progress the board and capture pieces.
- When a piece has reached the King’s Row (furthest row from its original side) then it is “kinged” by placing a second token on top
![### Implementation Requirements for the Board Game
1. **Board Size Initialization and Error Handling:**
- Establish the size of the board via command line arguments. Ensure to handle errors for too many and too few arguments, as well as incorrect input sizes. If an invalid size is provided, the program should display a message indicating the problem and recover by asking for the size during runtime.
2. **Dynamic Memory Allocation:**
- Create the board using two-dimensional, dynamic memory allocation.
3. **Board Colorization:**
- The board must be correctly colored black and white using the provided code snippet as a base. Adjust this code as needed to provide the best user interface for your program:
```cpp
for (int i=0; i<rows; i++)
{
for (int j=0; j<cols; j++)
{
if (i % 2 == 0 && j % 2 == 0)
cout << "\033[30;47m " << board[i][j] << " ";
else if (i % 2 == 1 && j % 2 == 1)
cout << "\033[30;47m " << board[i][j] << " ";
else
cout << "\033[0m " << board[i][j] << " ";
cout << "\033[0m";
}
cout << endl;
}
```
4. **Game Setup and Play:**
- The game must be correctly set up and played as described in the "Set Up" and "How to Play" sections of the project documentation.
5. **Path of Travel:**
- The path of travel should be received as a single, C-style string. It must be parsed and handled to move the pieces correctly. The string should list square coordinates. The choice of delimiter and coordinate system is up to the programmer but should be clearly communicated to the user.
6. **Board Printing:**
- Print the board after each piece move, ensuring to include the defined coordinate system. This is crucial, particularly for pieces that may move multiple squares in one turn.
7. **Handling Captured Pieces:**
- Remove captured pieces from the board after each move.
8. **Turn Completion:**
- After a turn is completed, display the total number of captured pieces for each player.
9. **Kinging Pieces:**](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8759b09c-a99c-4616-917a-1eb84cf5071d%2Fa4616e1b-0eb0-4271-8307-bf054da6b603%2Fpg4eid_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Implementation Requirements for the Board Game
1. **Board Size Initialization and Error Handling:**
- Establish the size of the board via command line arguments. Ensure to handle errors for too many and too few arguments, as well as incorrect input sizes. If an invalid size is provided, the program should display a message indicating the problem and recover by asking for the size during runtime.
2. **Dynamic Memory Allocation:**
- Create the board using two-dimensional, dynamic memory allocation.
3. **Board Colorization:**
- The board must be correctly colored black and white using the provided code snippet as a base. Adjust this code as needed to provide the best user interface for your program:
```cpp
for (int i=0; i<rows; i++)
{
for (int j=0; j<cols; j++)
{
if (i % 2 == 0 && j % 2 == 0)
cout << "\033[30;47m " << board[i][j] << " ";
else if (i % 2 == 1 && j % 2 == 1)
cout << "\033[30;47m " << board[i][j] << " ";
else
cout << "\033[0m " << board[i][j] << " ";
cout << "\033[0m";
}
cout << endl;
}
```
4. **Game Setup and Play:**
- The game must be correctly set up and played as described in the "Set Up" and "How to Play" sections of the project documentation.
5. **Path of Travel:**
- The path of travel should be received as a single, C-style string. It must be parsed and handled to move the pieces correctly. The string should list square coordinates. The choice of delimiter and coordinate system is up to the programmer but should be clearly communicated to the user.
6. **Board Printing:**
- Print the board after each piece move, ensuring to include the defined coordinate system. This is crucial, particularly for pieces that may move multiple squares in one turn.
7. **Handling Captured Pieces:**
- Remove captured pieces from the board after each move.
8. **Turn Completion:**
- After a turn is completed, display the total number of captured pieces for each player.
9. **Kinging Pieces:**
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
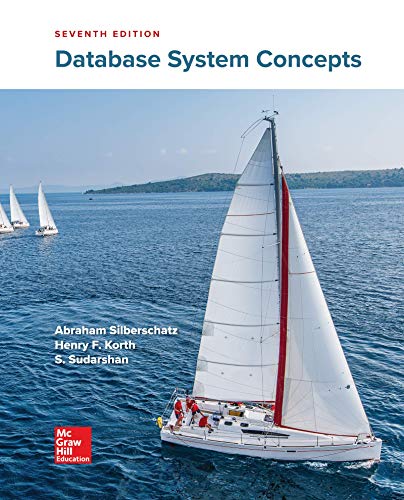
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
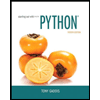
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
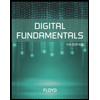
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
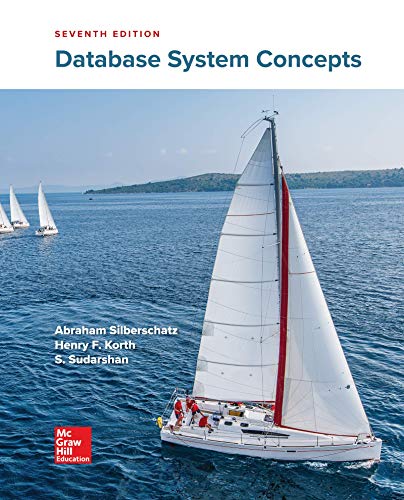
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
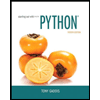
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
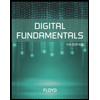
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
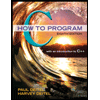
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
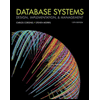
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
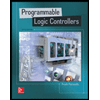
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education