Introduction In this lab you will find several exercises to strengthen your understanding of recursion and C++ arrays. Turn in all your work (code, spreadsheets, graphs, and short answers) into Blackboard. Part 1: Recursion A. Write a recursive function named factorial that computes the factorial for a given int. B. Write a recursive function named fibonacci that computes the value of the nth Fibonacci sequence: [1, 2, 3, 5, ...] C. Write a function named towers that counts the number of moves in Towers of Hanoi given the number of rings n. Part 2: Unit Testing Write test functions for the three functions in part 1. Name your test functions with a "test" prefix; for example, testRecursive Contains. Use the minimal test equivalence class to ensure a minimal yet complete test. Use c-style asserts to verify and validate values or print to the console for manual verification. Part 3: Time Complexity Review Big-O notation in the link provided'. In this exercise we'll explore the time complexity of the recursive Towers of Hanoi solution. Below you'll see a Big-O complexity chart we'll use later containing common ways algorithm operations can scale with user input. The towers function you created outputs the number of moves required for the number of rings given. Use this function to gather several input and output sample points. Enter these points into a spreadsheet program such as Google Sheets or Microsoft Excel. Once you have a dozen or sample points, create a plot like in the Big-O complexity chart.
Introduction In this lab you will find several exercises to strengthen your understanding of recursion and C++ arrays. Turn in all your work (code, spreadsheets, graphs, and short answers) into Blackboard. Part 1: Recursion A. Write a recursive function named factorial that computes the factorial for a given int. B. Write a recursive function named fibonacci that computes the value of the nth Fibonacci sequence: [1, 2, 3, 5, ...] C. Write a function named towers that counts the number of moves in Towers of Hanoi given the number of rings n. Part 2: Unit Testing Write test functions for the three functions in part 1. Name your test functions with a "test" prefix; for example, testRecursive Contains. Use the minimal test equivalence class to ensure a minimal yet complete test. Use c-style asserts to verify and validate values or print to the console for manual verification. Part 3: Time Complexity Review Big-O notation in the link provided'. In this exercise we'll explore the time complexity of the recursive Towers of Hanoi solution. Below you'll see a Big-O complexity chart we'll use later containing common ways algorithm operations can scale with user input. The towers function you created outputs the number of moves required for the number of rings given. Use this function to gather several input and output sample points. Enter these points into a spreadsheet program such as Google Sheets or Microsoft Excel. Once you have a dozen or sample points, create a plot like in the Big-O complexity chart.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![Introduction
In this lab you will find several exercises to strengthen your understanding of recursion and
C++ arrays. Turn in all your work (code, spreadsheets, graphs, and short answers) into
Blackboard.
Part 1: Recursion
A. Write a recursive function named factorial that computes the factorial for a given
int.
B. Write a recursive function named fibonacci that computes the value of the nth
Fibonacci sequence: [1, 2, 3, 5, ...]
C. Write a function named towers that counts the number of moves in Towers of Hanoi
given the number of rings n.
Part 2: Unit Testing
Write test functions for the three functions in part 1. Name your test functions with
a "test" prefix; for example, testRecursive Contains. Use the minimal test equivalence
class to ensure a minimal yet complete test. Use c-style asserts to verify and validate
values or print to the console for manual verification.
Part 3: Time Complexity
Review Big-O notation in the link provided'. In this exercise we'll explore the time
complexity of the recursive Towers of Hanoi solution. Below you'll see a Big-O complexity
chart we'll use later containing common ways algorithm operations can scale with user
input.
The towers function you created outputs the number of moves required for the
number of rings given. Use this function to gather several input and output sample points.
Enter these points into a spreadsheet program such as Google Sheets or Microsoft Excel.
Once you have a dozen or sample points, create a plot like in the Big-O complexity chart.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc97a1019-e6b4-4b8d-9bc8-da000b2e5be1%2Ff025d6ea-d800-4af2-b6e4-f5bfae86fc4c%2Ftc10t7_processed.png&w=3840&q=75)
Transcribed Image Text:Introduction
In this lab you will find several exercises to strengthen your understanding of recursion and
C++ arrays. Turn in all your work (code, spreadsheets, graphs, and short answers) into
Blackboard.
Part 1: Recursion
A. Write a recursive function named factorial that computes the factorial for a given
int.
B. Write a recursive function named fibonacci that computes the value of the nth
Fibonacci sequence: [1, 2, 3, 5, ...]
C. Write a function named towers that counts the number of moves in Towers of Hanoi
given the number of rings n.
Part 2: Unit Testing
Write test functions for the three functions in part 1. Name your test functions with
a "test" prefix; for example, testRecursive Contains. Use the minimal test equivalence
class to ensure a minimal yet complete test. Use c-style asserts to verify and validate
values or print to the console for manual verification.
Part 3: Time Complexity
Review Big-O notation in the link provided'. In this exercise we'll explore the time
complexity of the recursive Towers of Hanoi solution. Below you'll see a Big-O complexity
chart we'll use later containing common ways algorithm operations can scale with user
input.
The towers function you created outputs the number of moves required for the
number of rings given. Use this function to gather several input and output sample points.
Enter these points into a spreadsheet program such as Google Sheets or Microsoft Excel.
Once you have a dozen or sample points, create a plot like in the Big-O complexity chart.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
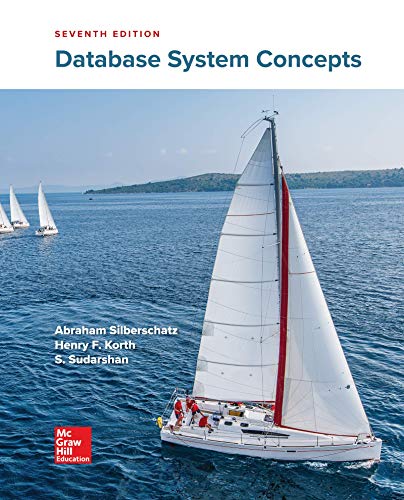
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
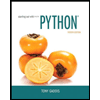
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
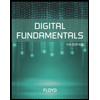
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
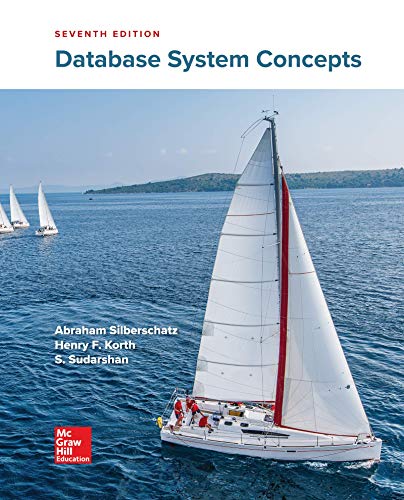
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
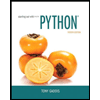
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
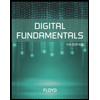
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
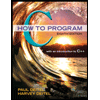
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
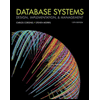
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
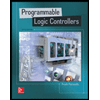
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education