Integer armchairCount is read from input as the number of input values that follow. The node headArmchair is created with the string "color". Use scnr.next() to read armchairCount strings. Insert a ArmchairNode for each string at the end of the linked list. Ex: If the input is 2 rose scarlet, then the output is: color rose scarlet import java.util.Scanner; public class ArmchairLinkedList { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); ArmchairNode headArmchair = null; ArmchairNode currArmchair = null; ArmchairNode lastArmchair = null; int armchairCount; String inputValue; int i; armchairCount = scnr.nextInt(); headArmchair = new ArmchairNode("color"); lastArmchair = headArmchair; currArmchair = headArmchair; while (currArmchair != null) { currArmchair.printNodeData(); currArmchair = currArmchair.getNext(); } } } class ArmchairNode { private String colorVal; private ArmchairNode nextNodeRef; public ArmchairNode(String colorInit) { this.colorVal = colorInit; this.nextNodeRef = null; } public void insertAfter(ArmchairNode nodeLoc) { ArmchairNode tmpNext = null; tmpNext = this.nextNodeRef; this.nextNodeRef = nodeLoc; nodeLoc.nextNodeRef = tmpNext; } public ArmchairNode getNext() { return this.nextNodeRef; } public void printNodeData() { System.out.println(this.colorVal); } }
Integer armchairCount is read from input as the number of input values that follow. The node headArmchair is created with the string "color". Use scnr.next() to read armchairCount strings. Insert a ArmchairNode for each string at the end of the linked list.
Ex: If the input is 2 rose scarlet, then the output is:
color rose scarlet
import java.util.Scanner;
public class ArmchairLinkedList {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
ArmchairNode headArmchair = null;
ArmchairNode currArmchair = null;
ArmchairNode lastArmchair = null;
int armchairCount;
String inputValue;
int i;
armchairCount = scnr.nextInt();
headArmchair = new ArmchairNode("color");
lastArmchair = headArmchair;
currArmchair = headArmchair;
while (currArmchair != null) {
currArmchair.printNodeData();
currArmchair = currArmchair.getNext();
}
}
}
class ArmchairNode {
private String colorVal;
private ArmchairNode nextNodeRef;
public ArmchairNode(String colorInit) {
this.colorVal = colorInit;
this.nextNodeRef = null;
}
public void insertAfter(ArmchairNode nodeLoc) {
ArmchairNode tmpNext = null;
tmpNext = this.nextNodeRef;
this.nextNodeRef = nodeLoc;
nodeLoc.nextNodeRef = tmpNext;
}
public ArmchairNode getNext() {
return this.nextNodeRef;
}
public void printNodeData() {
System.out.println(this.colorVal);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

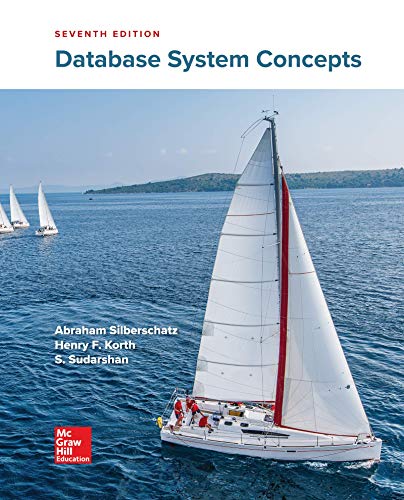
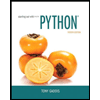
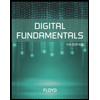
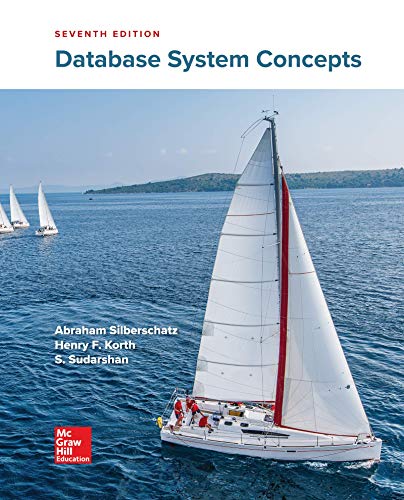
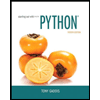
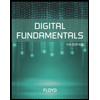
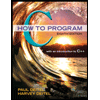
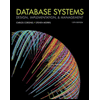
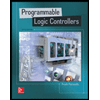