Instructions Write a function is_valid_month(date_list) that takes as a parameter a list of strings in the [MM, DD, YYYY] format and returns True if the provided month number is a possible month in the U.S. (i.e., an integer between 1 and 12 inclusive). Write a function is_valid_day (date_list) that takes as a parameter a list of strings in the [MM, DD, YYYY] format and returns True if the provided day is a possible day for the given month. You can use the provided dictionary. Note that you should call is_valid_month() within this function to help you validate the month. Write a function is_valid_year (date_list) that takes as a parameter a list of strings in the [MM, DD, YYYY] format and returns True if the provided year is a possible year: a positive integer. For the purposes of this lab, ensure that the year is also greater than 1000. Test Your Code #test incorrect types assert is_valid_month([12, 31, 2021]) == False assert is_valid_day ([12, 31, 2021]) == False assert is_valid_year ([12, 31, 2021]) == False Make sure that the input is of the correct type assert is_valid_month(["01", "01", "1970"]) == True assert is_valid_month(["12", "31", "2021"]) == True assert is_valid_day (["02", "03", "2000"]) == True assert is_valid_day (["12", "31", "2021"])== True assert is_valid_year (["10", "15", "2022"]) == True assert is_valid_year (["12", "31", "2021"]) == True Now, test the edge cases of the values: assert is_valid_month(["21", "01", "1970"]) == False assert is_valid_month(["-2", "31", "2021"]) == False assert is_valid_month(["March", "31", "2021"]) == False assert is_valid_day (["02", "33", "2000"]) == False assert is_valid_day (["02", "31", "2021"]) == False assert is_valid_day (["02", "1st", "2021"]) False assert is_valid_day (["14", "1st", "2021"]) == False assert is_valid_year (["10", "15", "22"]) == False assert is_valid_year (["12", "31", "-21"]) == False == Hints • Use the type () function from Section 2.1 and review the note in Section 4.3 to see the syntax for checking the type of a variable. • Refer to LAB 6.19 to review how to use the .isdigit() string function, which returns True if all characters in are the numbers 0-9.
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
please Use PYTHON
![```plaintext
Instructions
Write a function is_valid_month(date_list) that takes as a parameter a list of strings in the [MM, DD, YYYY] format and returns True if the provided month number is a possible month in the U.S. (i.e., an integer between 1 and 12 inclusive).
Write a function is_valid_day(date_list) that takes as a parameter a list of strings in the [MM, DD, YYYY] format and returns True if the provided day is a possible day for the given month. You can use the provided dictionary. Note that you should call is_valid_month() within this function to help you validate the month.
Write a function is_valid_year(date_list) that takes as a parameter a list of strings in the [MM, DD, YYYY] format and returns True if the provided year is a possible year: a positive integer. For the purposes of this lab, ensure that the year is also greater than 1000.
Test Your Code
# test incorrect types
assert is_valid_month([12, 31, 2021]) == False
assert is_valid_day([12, 31, 2021]) == False
assert is_valid_year([12, 31, 2021]) == False
Make sure that the input is of the correct type
assert is_valid_month(["01", "01", "1970"]) == True
assert is_valid_month(["12", "31", "2021"]) == True
assert is_valid_day(["02", "30", "2000"]) == False
assert is_valid_day(["12", "31", "2021"]) == True
assert is_valid_year(["10", "15", "2022"]) == True
assert is_valid_year(["12", "31", "2021"]) == True
Now, test the edge cases of the values:
assert is_valid_month(["21", "01", "1970"]) == False
assert is_valid_month(["-2", "31", "2021"]) == False
assert is_valid_month(["March", "31", "2021"]) == False
assert is_valid_day(["02", "33", "2000"]) == False
assert is_valid_day(["02", "12", "31"]) == False
assert is_valid_day(["02", "1st", "2021"]) == False
assert is_valid_day(["14", "31", "2021"]) == False](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fccac55ee-732b-4607-a38e-f5bd0a8aacb2%2Ffe334002-3c84-44e9-a27e-8ed49278f640%2Fdz1xweq_processed.png&w=3840&q=75)

Step by step
Solved in 4 steps with 3 images

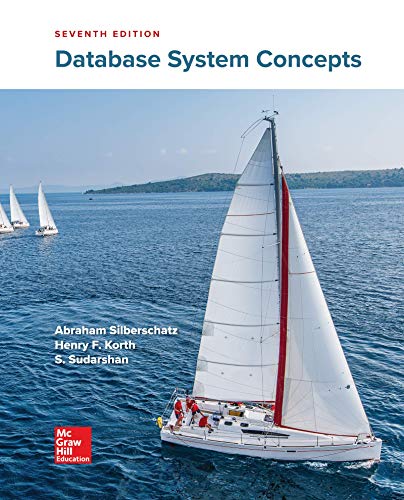
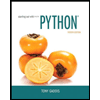
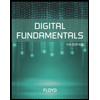
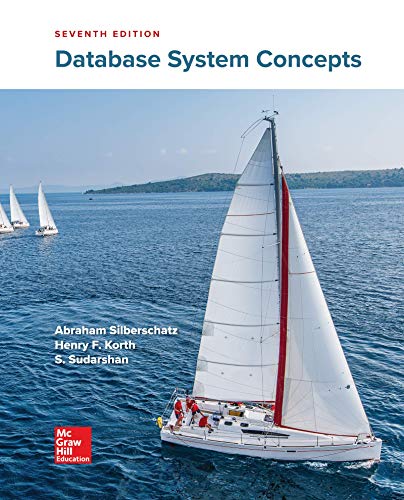
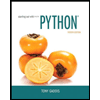
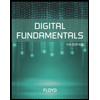
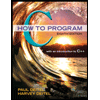
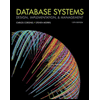
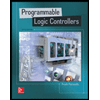