Information is present in the screenshot and below. Based on that need help in solving the code for this problem in python. The time complexity has to be as less as possible (nlogn or n at best, no n^2). Apply divide-and-conquer algorithm in the problem. Make sure all test cases return expected outputs by providing output screenshots. Provide screenshots Hint: Apply bisection method/modules Output Format Output contains a line with two space-separated integers W_a and W_b. - W_a is the maximum matchups won by Hamiltonia - W_b is the maximum matchups won by Burrgadia. Sample Input 0 3 5 5 4 4 0 2 4 1 0 Sample Output 0 3 0 Sample Input 1 5 4 8 3 3 4 8 5 1 8 3 Sample Output 1 2 2 Sample Input 2 7 8 10 2 8 12 1 9 12 6 0 13 1 9 8 5 1 Sample Output 2 7 0 The actual code """ Solves a test case Parameters: a : int - number of leaders in Hamiltonia b : int - number of leaders in Burrgadia s_i : array-like - rap proficiencies of Hamiltonia's leaders r_j : array-like - rap proficiencies of Burrgadia's leaders Returns: win_a : int - number of wins from Hamiltonia win_b : int - number of wins from Burrgadia """ def solve(a,b,s_i,r_j): # TODO a,b = list(map(int,input().strip().split(" "))) s_i = [int(input()) for i in range(a)] r_j = [int(input()) for i in range(b)] win_a, win_b = solve(a,b,s_i,r_j) print(f"{win_a} {win_b}")
Information is present in the screenshot and below. Based on that need help in solving the code for this problem in python. The time complexity has to be as less as possible (nlogn or n at best, no n^2). Apply divide-and-conquer
Output Format
Output contains a line with two space-separated integers W_a and W_b.
- W_a is the maximum matchups won by Hamiltonia
- W_b is the maximum matchups won by Burrgadia.
Sample Input 0
3 5
5
4
4
0
2
4
1
0
Sample Output 0
3 0
Sample Input 1
5 4
8
3
3
4
8
5
1
8
3
Sample Output 1
2 2
Sample Input 2
7 8
10
2
8
12
1
9
12
6
0
13
1
9
8
5
1
Sample Output 2
7 0
The actual code
""" Parameters: Returns: s_i = [int(input()) for i in range(a)] win_a, win_b = solve(a,b,s_i,r_j) print(f"{win_a} {win_b}") |


Step by step
Solved in 3 steps

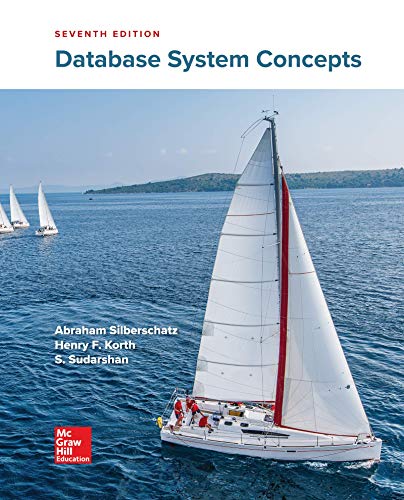
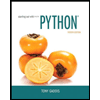
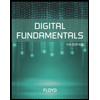
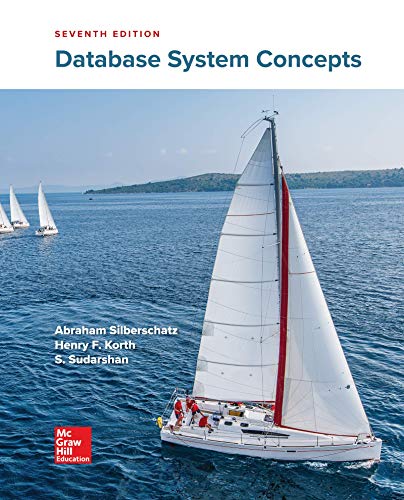
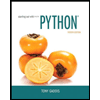
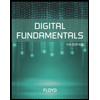
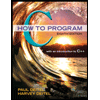
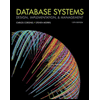
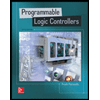