#include // size_t for sizes and indexes using namespace std; ///////||/ WRITE YOUR FUNCTION BELOW THIS LINE //// //////// int appendEvens(const int al[], int a2[], size_t sizel, size_t& size2) { int result; // Add your code here return result; }
#include // size_t for sizes and indexes using namespace std; ///////||/ WRITE YOUR FUNCTION BELOW THIS LINE //// //////// int appendEvens(const int al[], int a2[], size_t sizel, size_t& size2) { int result; // Add your code here return result; }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
C++
Can someone help me with this problem? I have been trying it but it still doesn't work.
Thank you
![```cpp
#include <cstddef> // size_t for sizes and indexes
using namespace std;
// WRITE YOUR FUNCTION BELOW THIS LINE
int appendEvens(const int a1[], int a2[], size_t size1, size_t& size2)
{
int result;
// Add your code here
return result;
}
```
### Testers
**Running Tester.cpp**
- Test results:
```
fail fail fail fail fail fail fail fail fail fail fail fail pass pass fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail
```
### Testing Append Evens
**Details:**
- **Function Call:** `appendEvens([21, 38, 32, 49, 12, 35, 45, 52], [], sizeA, sizeB)`
- **Returned Value:** `0`
- **Expected Value:** `4`
**Array Contents:**
- **Returned:** `[]`
- **Expected:** `[38, 32, 12, 52]`
- **Size:**
- **Returned:** `0`
- **Expected:** `4`
This test aims to verify the function `appendEvens`, which should append even numbers from the first array `a1[]` to the second array `a2[]`. The test expected the even numbers `[38, 32, 12, 52]` but returned an empty array, indicating that the implementation needs to be corrected. The size returned by the function also does not match the expected size of the appended array.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2f0d11ad-d1e5-4d2b-a52c-9bd1292a7fad%2Fb928c87e-22af-44f3-b1b7-93c99f9715c3%2F0wbbzeh_processed.png&w=3840&q=75)
Transcribed Image Text:```cpp
#include <cstddef> // size_t for sizes and indexes
using namespace std;
// WRITE YOUR FUNCTION BELOW THIS LINE
int appendEvens(const int a1[], int a2[], size_t size1, size_t& size2)
{
int result;
// Add your code here
return result;
}
```
### Testers
**Running Tester.cpp**
- Test results:
```
fail fail fail fail fail fail fail fail fail fail fail fail pass pass fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail fail
```
### Testing Append Evens
**Details:**
- **Function Call:** `appendEvens([21, 38, 32, 49, 12, 35, 45, 52], [], sizeA, sizeB)`
- **Returned Value:** `0`
- **Expected Value:** `4`
**Array Contents:**
- **Returned:** `[]`
- **Expected:** `[38, 32, 12, 52]`
- **Size:**
- **Returned:** `0`
- **Expected:** `4`
This test aims to verify the function `appendEvens`, which should append even numbers from the first array `a1[]` to the second array `a2[]`. The test expected the even numbers `[38, 32, 12, 52]` but returned an empty array, indicating that the implementation needs to be corrected. The size returned by the function also does not match the expected size of the appended array.
![## Function: `appendEvens()`
### Description
The `appendEvens()` function takes two arrays as input and appends all of the even elements from the first array to the second array. It is assumed that the second array has enough space to accommodate the additional elements.
### Parameters
The function accepts four arguments:
- **array 1**: This array should not be modified.
- **array 2**: This array may be modified as even elements will be appended to it.
- **size 1**: This is the size of the first array and it is not modified.
- **size 2**: This is the size of the second array, and it is modified as elements are appended.
### Return Value
The function returns the number of even elements appended. If the first array is empty, it returns `-1`.
### Example Usage
```c
const int MAX = 100;
int a1[MAX] = {1, 2, 3};
int a2[MAX] = {7, 9};
size_t size2 = 2;
int appended = appendEvens(a1, a2, 3, size2); // 1 appended
// After: size2->3, a2->[7, 9, 2]
```
### Explanation of Example
- **Input:**
- `a1` is the first array with elements `{1, 2, 3}`.
- `a2` is the second array with elements `{7, 9}`.
- The size of the second array `size2` is `2` initially.
- **Process:**
- The function finds the even number `2` in `a1` and appends it to `a2`.
- **Output:**
- The size `size2` is updated to `3`.
- The second array `a2` is now `[7, 9, 2]`.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2f0d11ad-d1e5-4d2b-a52c-9bd1292a7fad%2Fb928c87e-22af-44f3-b1b7-93c99f9715c3%2Fxj1by9_processed.png&w=3840&q=75)
Transcribed Image Text:## Function: `appendEvens()`
### Description
The `appendEvens()` function takes two arrays as input and appends all of the even elements from the first array to the second array. It is assumed that the second array has enough space to accommodate the additional elements.
### Parameters
The function accepts four arguments:
- **array 1**: This array should not be modified.
- **array 2**: This array may be modified as even elements will be appended to it.
- **size 1**: This is the size of the first array and it is not modified.
- **size 2**: This is the size of the second array, and it is modified as elements are appended.
### Return Value
The function returns the number of even elements appended. If the first array is empty, it returns `-1`.
### Example Usage
```c
const int MAX = 100;
int a1[MAX] = {1, 2, 3};
int a2[MAX] = {7, 9};
size_t size2 = 2;
int appended = appendEvens(a1, a2, 3, size2); // 1 appended
// After: size2->3, a2->[7, 9, 2]
```
### Explanation of Example
- **Input:**
- `a1` is the first array with elements `{1, 2, 3}`.
- `a2` is the second array with elements `{7, 9}`.
- The size of the second array `size2` is `2` initially.
- **Process:**
- The function finds the even number `2` in `a1` and appends it to `a2`.
- **Output:**
- The size `size2` is updated to `3`.
- The second array `a2` is now `[7, 9, 2]`.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 1 images

Recommended textbooks for you
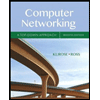
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
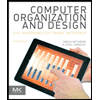
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
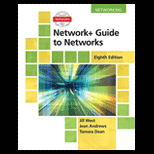
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
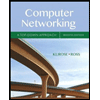
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
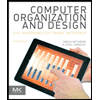
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
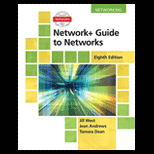
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
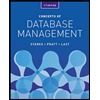
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
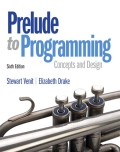
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
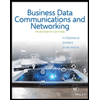
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY