In this lab, we will be building an application that uses an interactive menu. Let's say our high-level menu has the following options: L : List A : Add U : Update D : Delete S : Save R : Restore Q : Quit These key-option mappings will be stored in a dictionary in the main program. print_main_menu() function Write the print_main_menu() that accepts a dictionary of keys-options like the one shown above and prints the menu options stored in that dictionary in an easy-to-read format. Below is an example of the result of calling print_main_menu() (notice the question it asks at the top - it is part of the function output):
- use a dictionary to store menu items
- use a function to print formatted menu options
- use a while loop to create an interactive
program - check the user input using if branches
- check that an option is correct (verify that a dictionary key exists)
- use break to interrupt the program execution
Introduction
In this lab, we will be building an application that uses an interactive menu.
Let's say our high-level menu has the following options:
L : List A : Add U : Update D : Delete S : Save R : Restore Q : Quit
These key-option mappings will be stored in a dictionary in the main program.
print_main_menu() function
Write the print_main_menu() that accepts a dictionary of keys-options like the one shown above and prints the menu options stored in that dictionary in an easy-to-read format. Below is an example of the result of calling print_main_menu() (notice the question it asks at the top - it is part of the function output):
Example
Given the menu with the following options as mentioned above, the call to print_main_menu(main_menu) will output:
========================== What would you like to do? L - List A - Add U - Update D - Delete S - Save the data R - Restore data from file Q - Quit this program ==========================
Program flow
The expected program flow is:
- The main program starts with a menu of options given above
- Loop indefinitely (while the user didn't choose to exit):
- Print the menu to the user
- Get the user's choice from input()
- Check if the user's choice is a valid option in the menu (is it one of the dictionary keys?).
- If the input is a valid option, print the option that user selected
- If not, simply continue from the top of the loop
- If the user entered 'Q', break the while loop
Instructions
-
Fix TODO 1: Add the options from the instructions to the_menu dictionary inside the main program.
-
Fix TODO 2: Implement the "Quit" option, breaking from the while loop if the user input is an uppercase OR lowercase "Q".
-
Fix TODO 3: Check whether a provided option is a valid menu option.
Each time a valid menu option is provided, the program "echoes" it back to the user as follows:
print(f"You selected option {opt} to > {the_menu[opt]}.")
Hints
- Make sure you do not hard-code the menu options in your functions - the options need to be retrieved from the dictionary provided as a parameter to the function.
enter solution here:
![=zyBooks
+5.21 LABLECTURE-The Tao Toe
Students:
This content contreded by your instructor, and
and is not
zyBooks content Direct questions or consems about
this content to your instructor if you have
technical issues with the zylab suberiam
use the Trouble with lab button at the bottom of the
5.22 LAB CHECKPOINT: In
CSWB Learning Goals
use a dictionary to store menu items
use a function to print formatted menu
options
use a while loop to create an interactive
program
check the user input using if branches
check that an option is correct (verify that a
dictionary key exista)
use break to interrupt the program execution
Introduction
in this lab, we will be building an application that
uses an interactive menu
Let's say our high-level menu has the following
options
1: 1st
A: Add
U: Update
D Delete
Pelate
8: Save
R: Restore
Q: Quit
These key-option mappings will be stored in a
dictionary in the main program.
print_main_menu() function
Write the print nain nenu() that accepts a
dictionary of keys-options like the one shown above
and prints the menu options stored in that
dictionary in an easy-to-read format. Below is an
example of the result of calling
print nain nenu() (notice the question it asks
at the top-it is part of the function output:
Example
Given the menu with the following options as
mentioned above, the call to
print nain nenu(main menu) will output:
What would you like to do?
1 List
A Add
U
Update
D Delete
..
8- Save the data
Dave in data
R- Restore data from file
Q- Quit this progran
Program flow
The expected program flow is
The main program starts with a menu of
options given above
Loop indefinitely (while the user didn't choose
to ext
e-
Print the menu to the user
Get the user's choice from input()
Check if the user's choice is a valid
option in the menus one of the
dictionary keys?).
. If the input is a valid option, print
the option that user selected
not, simply continue from the
top of the loop
If the user entered , break the while
loop
Instructions
1. Fix TODO 1: Add the options from the
instructions to the menu dictionary inside
the main program
2. Fx TODO 2 Implement the "Quit" option,
breaking from the while loop if the user input
is an uppercase OR lowercase "Q"
3. Fix TODO & Check whether a provided option
is a valid menu option.
Each time a valid menu option is provided, the
program 'echoes' it back to the user as follows
print (f*You selected option (opt)
to the menu [opt]).")
Hints
Make sure you do not hard-code the menu
options in your functions the options need to
betrieved from the dictionary provided as a
parameter to the function
LAS
ACTIVITY 5.22. 1: LAB CHECKPOINT Interactive Me
1 def print_main_(menu):
9
9
Given a dictionary with the mens,
prints the keys and values as the
Termatted options.
5
6 Adds additional prints for decora
and outputs aquestion
7
!
8 "What would you like to do?"
18
11
12
13 5: Save
14 R Restore
15 Q: Quit
42-4
A Add
U Update
D Delete
16
17 for 1 in the menu
Develop mode
Submit mode
Run program
Enter program input (optional)
If your code requires input values, provide them her
Program output displayed here
Coding all of your work what is this?
9/2 0,0,0 min 16
Run your pr
Input value
second box
Input (from
+5.23 LAB: Print Beautification](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc6ce88e4-cc47-4e74-a0df-c59527d65207%2F1aebac1a-9b62-41cf-a4fd-ea93220a742c%2Fybqkj7_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

can you adjust it so the format looks like this with the equal signs and dashes please
==========================
What would you like to do?
L - List A - Add
U - Update
D - Delete
S - Save the data
R - Restore data from file
Q - Quit this
==========================
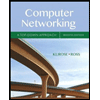
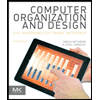
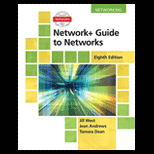
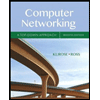
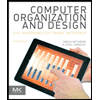
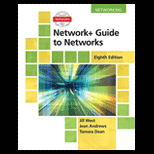
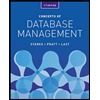
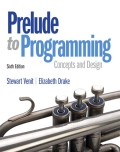
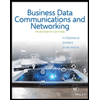