In the existing CircularlyLinkedList class, Write a non-static method named getMax for finding the maximum element in a circularly linked list. Write the testing code in the main method of the class CircularlyLinkedList. For this purpose, you must only use and update the CircularlyLinkedList.java file provided in Lesson3Examples posted in the eCentennialmodule "Lesson Examples (from textbook)"
In the existing CircularlyLinkedList class, Write a non-static method named getMax for finding the maximum element in a circularly linked list. Write the testing code in the main method of the class CircularlyLinkedList. For this purpose, you must only use and update the CircularlyLinkedList.java file provided in Lesson3Examples posted in the eCentennialmodule "Lesson Examples (from textbook)"
package exercise3;
public class CircularlyLinkedList<E> {
private static class Node<E> {
private E element;
private Node<E> next;
public Node(E e, Node<E> n) {
element = e;
next = n;
}
public E getElement() { return element; }
public Node<E> getNext() { return next; }
public void setNext(Node<E> n) { next = n; }
}
private Node<E> tail = null;
private int size = 0;
public CircularlyLinkedList() { }
public int size() { return size; }
public boolean isEmpty() { return size == 0; }
public E first() {
if (isEmpty()) return null;
return tail.getNext().getElement();
}
public E last() {
if (isEmpty()) return null;
return tail.getElement();
}
public void rotate() {
if (tail != null)
tail = tail.getNext();
}
public void addFirst(E e) {
if (size == 0) {
tail = new Node<>(e, null);
tail.setNext(tail);
} else {
Node<E> newest = new Node<>(e, tail.getNext());
tail.setNext(newest);
}
size++;
}
public void addLast(E e) {
addFirst(e);
tail = tail.getNext();
}
public E removeFirst() {
if (isEmpty()) return null;
Node<E> head = tail.getNext();
if (head == tail) tail = null;
else tail.setNext(head.getNext());
size--;
return head.getElement();
}
public String toString() { if (tail == null) return "()";
StringBuilder sb = new StringBuilder("(");
Node<E> walk = tail;
do {
walk = walk.getNext();
sb.append(walk.getElement());
if (walk != tail)
sb.append(", ");
} while (walk != tail);
sb.append(")");
return sb.toString();
}
@SuppressWarnings ("unchecked")
public CircularlyLinkedList<E> clone() throws CloneNotSupportedException{
CircularlyLinkedList<E> other = (CircularlyLinkedList<E>) super.clone();
if (size > 0) {
other.tail = new Node<>(tail.getElement(), null);
Node<E> walk = tail.getNext();
Node<E> otherTail = other.tail;
while (walk != tail) {
Node<E> newest = new Node<>(walk.getElement(), null);
otherTail.setNext(newest);
otherTail = newest;
walk = walk.getNext();
}
if(walk == tail) {
otherTail. setNext (other .tail);
otherTail = other.tail;
}
}
return other;
}
public static void main(String[] args)
{
CircularlyLinkedList<String> circularList = new
CircularlyLinkedList<String>();
circularList.addFirst("LAX");
circularList.addLast("MSP");
circularList.addLast("ATL");
circularList.addLast("BOS");
System.out.println("The Original List: " + circularList);
circularList.removeFirst();
System.out.println("List after removing First Node: " +circularList);
circularList.rotate();
System.out.println("List after Rotation: " + circularList);
try {
CircularlyLinkedList<String> cloneList = circularList.clone();
System.out.println("Cloned List:" + cloneList);
}catch(CloneNotSupportedException ce) {
System.out.println("Exception is thrown:" + ce);
}
}
}

Step by step
Solved in 3 steps with 1 images

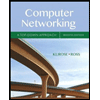
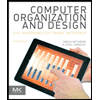
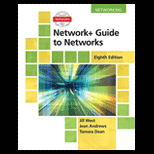
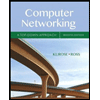
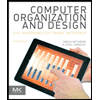
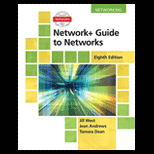
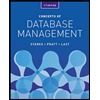
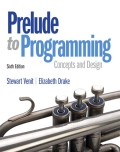
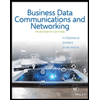