In the btnCalc_Click procedure, change the For…Next statement that controls the years to a Do…Loop statement. Option Explicit On Option Strict On Option Infer Off Public Class frmMain Private Sub btnCalc_Click(sender As Object, e As EventArgs) Handles btnCalc.Click Dim dblDeposit As Double Dim dblBalance As Double Double.TryParse(txtDeposit.Text, dblDeposit) txtBalance.Text = "Rate" & ControlChars.Tab & "Year" & ControlChars.Tab & "Balance" & ControlChars.NewLine End Sub Private Sub txtDeposit_Enter(sender As Object, e As EventArgs) Handles txtDeposit.Enter txtDeposit.SelectAll() End Sub Private Sub txtDeposit_TextChanged(sender As Object, e As EventArgs) Handles txtDeposit.TextChanged txtBalance.Text = String.Empty End Sub Private Sub btnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click Me.Close() End Sub Private Sub txtDeposit_KeyPress(sender As Object, e As KeyPressEventArgs) Handles txtDeposit.KeyPress ' Allows the text box to accept only numbers, the period, and the Backspace key. If (e.KeyChar < "0" OrElse e.KeyChar > "9") AndAlso e.KeyChar <> "." AndAlso e.KeyChar <> ControlChars.Back Then e.Handled = True End If End Sub End Class
In the btnCalc_Click procedure, change the For…Next statement that controls the years to a Do…Loop statement.
Option Explicit On
Option Strict On
Option Infer Off
Public Class frmMain
Private Sub btnCalc_Click(sender As Object, e As EventArgs) Handles btnCalc.Click
Dim dblDeposit As Double
Dim dblBalance As Double
Double.TryParse(txtDeposit.Text, dblDeposit)
txtBalance.Text = "Rate" & ControlChars.Tab &
"Year" & ControlChars.Tab & "Balance" &
ControlChars.NewLine
End Sub
Private Sub txtDeposit_Enter(sender As Object, e As EventArgs) Handles txtDeposit.Enter
txtDeposit.SelectAll()
End Sub
Private Sub txtDeposit_TextChanged(sender As Object, e As EventArgs) Handles txtDeposit.TextChanged
txtBalance.Text = String.Empty
End Sub
Private Sub btnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click
Me.Close()
End Sub
Private Sub txtDeposit_KeyPress(sender As Object, e As KeyPressEventArgs) Handles txtDeposit.KeyPress
' Allows the text box to accept only numbers, the period, and the Backspace key.
If (e.KeyChar < "0" OrElse e.KeyChar > "9") AndAlso e.KeyChar <> "." AndAlso e.KeyChar <> ControlChars.Back Then
e.Handled = True
End If
End Sub
End Class

Answer:
Visual Studio Source Code:
Method 1:
Form1.vb:
Option Explicit On
Option Strict On
Option Infer Off
Public Class Form1
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
Dim dblDeposit As Double
Dim dblBalance As Double
Double.TryParse(txtDeposit.Text, dblDeposit)
txtBalance.Text = "Rate" & ControlChars.Tab &
"Year" & ControlChars.Tab & "Balance" &
ControlChars.NewLine
For dbl_Rate As Double = 0.03 To 0.07 Step 0.01
txtBalance.Text = txtBalance.Text &
dbl_Rate.ToString("P0") & ControlChars.NewLine
For int_Year As Integer = 1 To 5
dblBalance = dblDeposit * (1 + dbl_Rate) ^ int_Year
txtBalance.Text = txtBalance.Text &
ControlChars.Tab & int_Year.ToString &
ControlChars.Tab & dblBalance.ToString("C2") &
ControlChars.NewLine
Next int_Year
Next dbl_Rate
End Sub
Private Sub txtDeposit_Enter(sender As Object, e As EventArgs) Handles txtDeposit.Enter
txtDeposit.SelectAll()
End Sub
Private Sub txtDeposit_TextChanged(sender As Object, e As EventArgs) Handles txtDeposit.TextChanged
txtBalance.Text = String.Empty
End Sub
Private Sub btnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click
Me.Close()
End Sub
Private Sub txtDeposit_KeyPress(sender As Object, e As KeyPressEventArgs) Handles txtDeposit.KeyPress
If (e.KeyChar < "0" OrElse e.KeyChar > "9") AndAlso e.KeyChar <> "." AndAlso e.KeyChar <> ControlChars.Back Then
e.Handled = True
End If
End Sub
End Class
Output Screenshot:
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

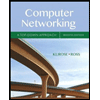
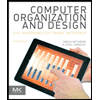
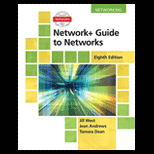
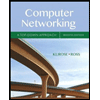
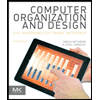
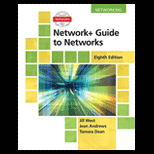
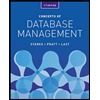
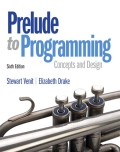
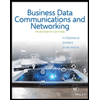