In Python Please check the pictures first. Write a program that keeps names and email addresses in a dictionary as key-value pairs. The program should display a menu that lets the user look up a person’s email address, add a new name and email address, change an existing email address, and delete an existing name and email address. The program should save the data stored in a dictionary to a file when the user exits the program. Each time the program starts, it should retrieve the data from the file and store it in a dictionary. You Must create and use at least 5 meaningful functions. A function to display a menu A function to look up a person’s email address A function to add a new name and email address A function to change an email address A function to delete a name and email address. Check the Your program should check user input; for example, if the user wants to delete a name that does not exist in the dictionary, you should print something like: the name is not in the database. A sample one run is shown below. Hints for how the format of the program HAVE to be written : a function to print the menu a function to look up an email address (person) a function to add a name and email (person, email) a function to change email (person) a function to delete a name and email address (person) flag = -1 read data from a file and store it in a dictionary – name email get user option and store it in variable flag while flag ! = 5 multi – way selection to process user option (5 options) if flag == 1 if flag == 2 if flag == 3 if flag == 4 if flag == 5 call function menu again get user option again write the data in the dictionary in a file This is the Required Output example: Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 2 Enter name: John Enter email address: John@yahoo.com That name already exists Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 2 Enter name: Jack Enter email address: Jack@yahoo.com Name and address have been added Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 1 Enter a name: Sam The specified name was not found Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 1 Enter a name: Jack Name: Jack Email: Jack@yahoo.com Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 3 Enter name: John Enter the new address: John@wayne.edu Information updated Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 1 Enter a name: John Name: John Email: John@wayne.edu Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 4 Enter name: Sam The specified name was not found Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 4 Enter name: Jack Information deleted Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 1 Enter a name: Jack The specified name was not found Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 5 Information saved >>> Please check the pictures and fix the code. Upvote for following the required steps and working code. Thank You.
In Python
Please check the pictures first.
Write a program that keeps names and email addresses in a dictionary as key-value pairs.
The program should display a menu that lets the user look up a person’s email address, add a new name and email address, change an existing email address, and delete an existing name and email address. The program should save the data stored in a dictionary to a file when the user exits the program. Each time the program starts, it should retrieve the data from the file and store it in a dictionary.
You Must create and use at least 5 meaningful functions.
- A function to display a menu
- A function to look up a person’s email address
- A function to add a new name and email address
- A function to change an email address
- A function to delete a name and email address.
Check the Your program should check user input; for example, if the user wants to delete a name that does not exist in the dictionary, you should print something like: the name is not in the
Hints for how the format of the program HAVE to be written :
a function to print the menu
a function to look up an email address (person)
a function to add a name and email (person, email)
a function to change email (person)
a function to delete a name and email address (person)
flag = -1
read data from a file and store it in a dictionary – name email
get user option and store it in variable flag
while flag ! = 5
multi – way selection to process user option (5 options)
if flag == 1
if flag == 2
if flag == 3
if flag == 4
if flag == 5
call function menu again
get user option again
write the data in the dictionary in a file
This is the Required Output example:
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 2 Enter name: John Enter email address: John@yahoo.com That name already exists
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 2 Enter name: Jack Enter email address: Jack@yahoo.com Name and address have been added
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 1 Enter a name: Sam The specified name was not found
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 1 Enter a name: Jack Name: Jack Email: Jack@yahoo.com
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 3 Enter name: John Enter the new address: John@wayne.edu Information updated
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 1 Enter a name: John Name: John Email: John@wayne.edu
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 4 Enter name: Sam The specified name was not found
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 4 Enter name: Jack Information deleted
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 1 Enter a name: Jack The specified name was not found
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 5 Information saved >>> |
Please check the pictures and fix the code.
Upvote for following the required steps and working code. Thank You.
![File Edit Format Run Options Window Help
File
Home
Insert
Design
Layout
References
Mailings
Review
View
Help
Grammarly
32
33
34
35 flag = -1
36 record = {}
37 file = open ("record.txt", "r")
38 lines = file.readlines ()
39 for line in lines:
40
41
42
else:
print ("The specified name was not found")
You Must create and use at least 5 meaningful functions.
A function to display a menu
A function to look up a person's email address
A function to add a new name and email address
A function to change an email address
A function to delete a name and email address.
x = line. split(" ")
name = x[0]
email = x[1]
record[name]
Your program should check user input; for example, if the user wants to delete a name that does
not exist in the dictionary, you should print something like: the name is not in the database. A
sample one run is shown below.
43
= email
44 file.close ()
45
46 printmenu ()
47 flag = int (input ("Enter your choice: "))
48 while True:
49
50
51
52
53
54
55
56
57
58
59
Hints for how the format of the program HAVE be written :
a function to print the menu
a function to look up an email address (person)
af unction to add a name and email (person, email)
if flag == 1:
name
input ("Enter a name: ")
lookup (record, name)
elif flag == 2:
name = input ("Enter name: ")
email = input ("Enter email address: ")
add (record, name, email)
elif flag == 3:
name = input ("Enter name: ")
email = input ("Enter the new address: ")
update (record, name, email)
elif flag == 4:
a function to change email (person)
a function to delete a name and email address (person)
flag = -1
read data from a file and store it in a dictionary – name email
get user option and store it in variable flag
-
while flag ! = 5
multi – way selection to process user option (5 options)
if flag
if flag
if flag
if flag
60
61
62
63
64
65
66
= input ("Enter a name: ")
name
== 1
delete (record, name)
elif flag == 5:
f = open ("record.txt", "w+")
for key, value in record.items () :
1 = key+" "+value+"\n"
f.write (1)
2
==
3
4
if flag
call function menu again
get user option again
==
67
68
f.close ()
69
print ("Information saved")
70
71
72
break
printmenu ()
flag = int (input ("Enter your choice: "))
write the data in the dictionary in a file
73
Page 1 of 4
843 words
English (United States)
D Focus
100%](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc425dcf7-6a36-4614-85d2-627727592108%2Fd2483f3a-10e9-4b72-8933-da5fd984360e%2Fws68tum_processed.jpeg&w=3840&q=75)
![Project Python.py - C:/Users/17737/OneDrive/Desktop/Project Python.py (3.8.4)
*Python 3.8.4 Shell*
File Edit Format Run Options Window Help
1 def printmenu () :
File Edit Shell Debug Options Window Help
Python 3.8.4 (tags/v3.8.4:dfa645a, Jul 13 2020, 16:46:45) [MSC v.1924 64 bit (AMD64)] on win32
Type "help", "copyright", "credits" or "license () " for more information.
print ("Menu")
print ('
print ("1. Look up an email address")
print ("2. Add a new name and email address")
print ("3. Change an existing email address")
print ("4. Delete a name and email address")
print ("5. Quit the program")
--")
>>>
4
%=== RESTART: C:/Users/17737/OneDrive/Desktop/Project Python.py
Traceback (most recent call last):
File "C:/Users/17737/OneDrive/Desktop/Project Python.py", line 42, in <module>
email = x[1]
IndexError: list index out of range
>>> getting an error on line 42
9.
10 def lookup (record, name) :
11
12
record - Notepad
if name in record:
print ("Name : ", name)
print ("Email:", record[name])
File Edit Format View Help
13
Hom John@yahoo.com
Jack@yahoo.com
John@wayne.edu
14
else:
File
15
print ("The specified name was not found")
16
17 def add (record, name, email):
if name in record:
18
19
20
21
22
23
24 def update (record, name, email):
25
26
27
28 def delete (record, name) :
29
30
31
32
33
34
35 flag = -1
36 record = {}
37 file = open ("record.txt", "r")
38 lines = file.readlines ()
39 for line in lines:
print ("The name already exists")
else:
Ln 2, Col 15
100%
Windows
record[name] = email
print ("Name and address have been added")
Write a program that keeps names and email addresses in a dictionary as key-value pairs.
The program should display a menu that lets the user look up a person's email address, add a
new name and email address, change an existing email address, and delete an existing name and
email address. The program should save the data stored in a dictionary to a file when the user
exits the program. Each time the program starts, it should retrieve the data from the file and store
it in a dictionary.
record[name] = email
print ("Information updated")
if name in record:
del record[name]
print ("Information deleted")
You Must create and use at least 5 meaningful functions.
else:
A function to display a menu
A function to look up a person's email address
A function to add a new name and email address
A function to change an email address
A function to delete a name and email address.
print ("The specified name was not found")
40
41
x = line. split(" ")
name = x[0]
email = x[1]
Your program should check user input; for example, if the user wants to delete a name that does
not exist in the dictionary, you should print something like: the name is not in the database. A
sample one run is shown below.
42
是](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc425dcf7-6a36-4614-85d2-627727592108%2Fd2483f3a-10e9-4b72-8933-da5fd984360e%2F8bwaga8_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

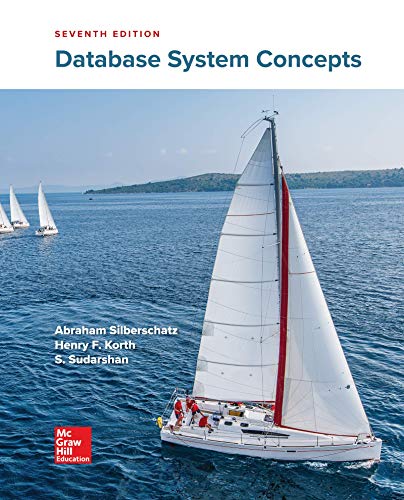
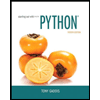
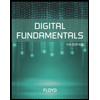
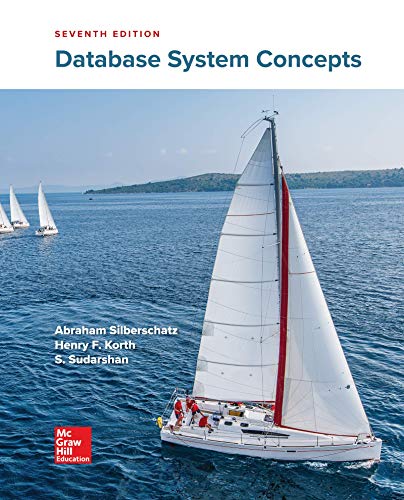
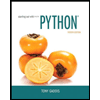
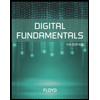
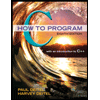
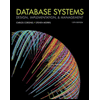
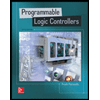