in python 1. Looking at the following class definition. What is the name of the superclass? What is the name of the subclass? class Rectangle(BBox): 2.For this question you will be writting a subclass to the superclass written below. You are given class Account below that has appropriate attributes and behaviors. You will write class CheckingAccount to be a subclass of class Account that has the added or changed features Class CheckingAccount Has a static attribute called minBalance that is initialized to 500.00 Has a consturctor that accepts a persons name and initialbalance by parameter and initializes an Account correctly using that information Includes an overridden withdraw method that will not allow you to withdraw an amount that would put your current balance below the minimumBalance. You must use the static attribute for this method for full points. It should adjust the balance appropriately if the withdraw can be made and should make no change to the balance if the withdraw can not be made. class Account(object): def __init__(self, name, balance): self.__name = name self.__balance = balance def deposit(self, amount): self.__balance += amount def withdraw(self, amount): if amount <= self.__balance: self.__balance -= amount def getBalance(self): return self.__balance def getName(self): return self.__name
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
in python
1. Looking at the following class definition. What is the name of the superclass? What is the name of the subclass?
class Rectangle(BBox):
2.For this question you will be writting a subclass to the superclass written below.
You are given class Account below that has appropriate attributes and behaviors. You will write class CheckingAccount to be a subclass of class Account that has the added or changed features
Class CheckingAccount
- Has a static attribute called minBalance that is initialized to 500.00
- Has a consturctor that accepts a persons name and initialbalance by parameter and initializes an Account correctly using that information
- Includes an overridden withdraw method that will not allow you to withdraw an amount that would put your current balance below the minimumBalance. You must use the static attribute for this method for full points. It should adjust the balance appropriately if the withdraw can be made and should make no change to the balance if the withdraw can not be made.
class Account(object):
def __init__(self, name, balance):
self.__name = name
self.__balance = balance
def deposit(self, amount):
self.__balance += amount
def withdraw(self, amount):
if amount <= self.__balance:
self.__balance -= amount
def getBalance(self):
return self.__balance
def getName(self):
return self.__name

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

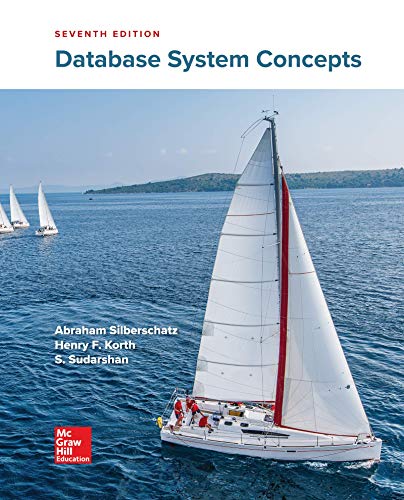
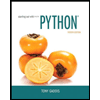
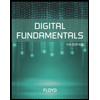
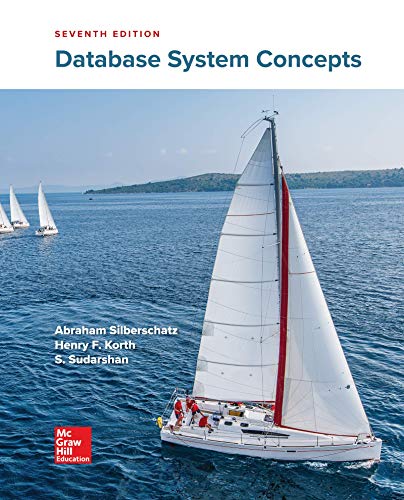
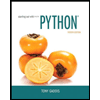
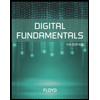
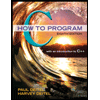
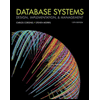
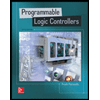