In numerical methods, one source of error occurs when we use an approximation for a mathematical expression that would otherwise be too costly to compute in terms of run-time or memory resources. One routine example is the approximation of infinite series by a finite series that mostly captures the important behavior of the infinite series. In this problem you will implement an approximation to the exp(2) as represented by the following infinite series, Your approximation will be a truncated finite series with N +1 terms, Part 1 For the first part of this problem, you are given a random real number and will investigate how well a finite series expansion for exp(2) approximates the infinite series. • Compute exp(2) using a finite series approximation with N = [0,9] CN (i.e. N is an integer). • Save the 10 floating point values from your approximation in a numpy array named exp_approx. exp_approx should be of shape (10.) and should be ordered with increasing N (i.e. the first entry of exp_approx should correspond to exp(, N) when I = 0 and the last entry when N = 9). X Part 2 For the second part of this problem, you are asked to find an integer value Noutoff € [0,9] such that the finite series is guaranteed to have a relative error less than some desired amount. The relative error is with respect to the true exp(x) value that would result from an infinite series. Noutoff should be the smallest integer that satisfies the desired relative error constraint. Input/Output The setup code provides the following variables: Name desired_rel_error First, save the absolute and relative errors for each value of N in abs_error and rel_error. • Given a desired decimal relative error, desired_rel_error, find the smallest N that guarantees the relative error is below desired_rel_error. Assign this N to a variable N_cutoff. • Plot the relative error vs. N (error on the y-axis and N on the x-axis). Make the y-axis log scale. You should have 10 values. Provide appropriate formatting (e.g. labels, title, markers, et cetera). Name exp(*) exp_approx abs_error = rel_error +0 N_cutoff n! Your code snippet should define the following variables: Description array giving your estimates for exp(x) array giving the absolute error for using the taylor approximation array giving the relative error for using the taylor approximation the smallest N that satisfies the desired relative error constraint semilogy plot of the relative error vs. N plot N 2" exp(x, N) => n! 7-0 Type 1-D Numpy Array 1-D Numpy Array 1-D Numpy Array int subplot Type Description float random real number where the function will be evaluated float the error limit in computing the value of N Your code snippet mote also generate the following plot • Plot of relative error vs. N. log-scale on the y-axis (plt.semilogy). Provide appropriate axes labels and a title. Save your plot to a variable plot.
In numerical methods, one source of error occurs when we use an approximation for a mathematical expression that would otherwise be too costly to compute in terms of run-time or memory resources. One routine example is the approximation of infinite series by a finite series that mostly captures the important behavior of the infinite series. In this problem you will implement an approximation to the exp(2) as represented by the following infinite series, Your approximation will be a truncated finite series with N +1 terms, Part 1 For the first part of this problem, you are given a random real number and will investigate how well a finite series expansion for exp(2) approximates the infinite series. • Compute exp(2) using a finite series approximation with N = [0,9] CN (i.e. N is an integer). • Save the 10 floating point values from your approximation in a numpy array named exp_approx. exp_approx should be of shape (10.) and should be ordered with increasing N (i.e. the first entry of exp_approx should correspond to exp(, N) when I = 0 and the last entry when N = 9). X Part 2 For the second part of this problem, you are asked to find an integer value Noutoff € [0,9] such that the finite series is guaranteed to have a relative error less than some desired amount. The relative error is with respect to the true exp(x) value that would result from an infinite series. Noutoff should be the smallest integer that satisfies the desired relative error constraint. Input/Output The setup code provides the following variables: Name desired_rel_error First, save the absolute and relative errors for each value of N in abs_error and rel_error. • Given a desired decimal relative error, desired_rel_error, find the smallest N that guarantees the relative error is below desired_rel_error. Assign this N to a variable N_cutoff. • Plot the relative error vs. N (error on the y-axis and N on the x-axis). Make the y-axis log scale. You should have 10 values. Provide appropriate formatting (e.g. labels, title, markers, et cetera). Name exp(*) exp_approx abs_error = rel_error +0 N_cutoff n! Your code snippet should define the following variables: Description array giving your estimates for exp(x) array giving the absolute error for using the taylor approximation array giving the relative error for using the taylor approximation the smallest N that satisfies the desired relative error constraint semilogy plot of the relative error vs. N plot N 2" exp(x, N) => n! 7-0 Type 1-D Numpy Array 1-D Numpy Array 1-D Numpy Array int subplot Type Description float random real number where the function will be evaluated float the error limit in computing the value of N Your code snippet mote also generate the following plot • Plot of relative error vs. N. log-scale on the y-axis (plt.semilogy). Provide appropriate axes labels and a title. Save your plot to a variable plot.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
python.
Starter Code:
import numpy as np
import matplotlib.pyplot as plt
exp_approx = np.zeros(10)
abs_error = np.zeros(10)
rel_error = np.zeros(10)
N_cutoff = 0
# Save plot for grading
plot = plt.gca()
![In numerical methods, one source of error occurs when we use an approximation for a mathematical
expression that would otherwise be too costly to compute in terms of run-time or memory resources. One
routine example is the approximation of infinite series by a finite series that mostly captures the important
behavior of the infinite series.
In this problem you will implement an approximation to the exp(2) as represented by the following infinite
series,
exp(*)
Name
=
Your approximation will be a truncated finite series with N + 1 terms,
Input/Output
The setup code provides the following variables:
X
00
desired_rel_error
+0
Type
float
exp(x, N) =
Z"
Part 1
For the first part of this problem, you are given a random real number x and will investigate how well a finite
series expansion for exp(x) approximates the infinite series.
• Compute exp(2) using a finite series approximation with N = [0,9] CN (i.e. N is an integer).
• Save the 10 floating point values from your approximation in a numpy array named exp_approx.
exp_approx should be of shape (10.) and should be ordered with increasing N (i.e. the first entry of
exp_approx should correspond to exp(, N) when N = 0 and the last entry when N = 9).
n!
N
Part 2
For the second part of this problem, you are asked to find an integer value Noutoff € [0,9] such that the finite
series is guaranteed to have a relative error less than some desired amount. The relative error is with respect to
the true exp(2) value that would result from an infinite series. Newtoff should be the smallest integer that
satisfies the desired relative error constraint.
• First, save the absolute and relative errors for each value of N in abs_error and rel_error.
• Given a desired decimal relative error, desired_rel_error, find the smallest N that guarantees the
relative error is below desired_rel_error. Assign this N to a variable N_cutoff.
•
Plot the relative error vs. N (error on the y-axis and N on the x-axis). Make the y-axis log scale. You
should have 10 values. Provide appropriate formatting (e.g. labels, title, markers, et cetera).
7-0
7-11
I
Description
random real number where the function will be evaluated
float the error limit in computing the value of N
Your code snippet should define the following variables:
Name Type
Description
array giving your estimates for exp(x)
array giving the absolute error for using the taylor approximation
exp_approx 1-D Numpy Array
abs_error 1-D Numpy Array
rel_error 1-D Numpy Array
N_cutoff int
array giving the relative error for using the taylor approximation
the smallest N that satisfies the desired relative error constraint
semilogy plot of the relative error vs. N
plot
subplot
Your code snippet mote also generate the following plot
• Plot of relative error vs. N. log-scale on the y-axis (plt.senilogy). Provide appropriate axes labels and a
title. Save your plot to a variable plot.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F840ac9d4-eb27-4280-b70d-21eed9a81895%2F65bf4566-7a2b-4d4c-aed2-60ffab9fe0c1%2Fs747mo_processed.png&w=3840&q=75)
Transcribed Image Text:In numerical methods, one source of error occurs when we use an approximation for a mathematical
expression that would otherwise be too costly to compute in terms of run-time or memory resources. One
routine example is the approximation of infinite series by a finite series that mostly captures the important
behavior of the infinite series.
In this problem you will implement an approximation to the exp(2) as represented by the following infinite
series,
exp(*)
Name
=
Your approximation will be a truncated finite series with N + 1 terms,
Input/Output
The setup code provides the following variables:
X
00
desired_rel_error
+0
Type
float
exp(x, N) =
Z"
Part 1
For the first part of this problem, you are given a random real number x and will investigate how well a finite
series expansion for exp(x) approximates the infinite series.
• Compute exp(2) using a finite series approximation with N = [0,9] CN (i.e. N is an integer).
• Save the 10 floating point values from your approximation in a numpy array named exp_approx.
exp_approx should be of shape (10.) and should be ordered with increasing N (i.e. the first entry of
exp_approx should correspond to exp(, N) when N = 0 and the last entry when N = 9).
n!
N
Part 2
For the second part of this problem, you are asked to find an integer value Noutoff € [0,9] such that the finite
series is guaranteed to have a relative error less than some desired amount. The relative error is with respect to
the true exp(2) value that would result from an infinite series. Newtoff should be the smallest integer that
satisfies the desired relative error constraint.
• First, save the absolute and relative errors for each value of N in abs_error and rel_error.
• Given a desired decimal relative error, desired_rel_error, find the smallest N that guarantees the
relative error is below desired_rel_error. Assign this N to a variable N_cutoff.
•
Plot the relative error vs. N (error on the y-axis and N on the x-axis). Make the y-axis log scale. You
should have 10 values. Provide appropriate formatting (e.g. labels, title, markers, et cetera).
7-0
7-11
I
Description
random real number where the function will be evaluated
float the error limit in computing the value of N
Your code snippet should define the following variables:
Name Type
Description
array giving your estimates for exp(x)
array giving the absolute error for using the taylor approximation
exp_approx 1-D Numpy Array
abs_error 1-D Numpy Array
rel_error 1-D Numpy Array
N_cutoff int
array giving the relative error for using the taylor approximation
the smallest N that satisfies the desired relative error constraint
semilogy plot of the relative error vs. N
plot
subplot
Your code snippet mote also generate the following plot
• Plot of relative error vs. N. log-scale on the y-axis (plt.senilogy). Provide appropriate axes labels and a
title. Save your plot to a variable plot.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
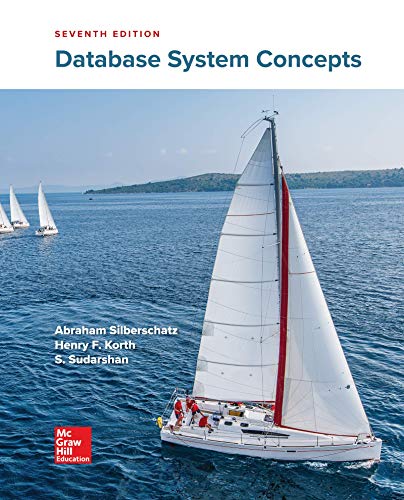
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
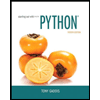
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
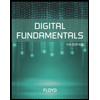
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
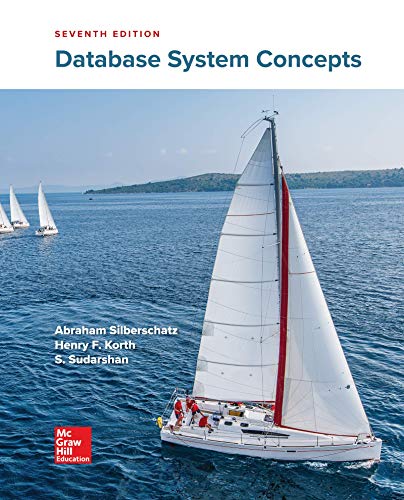
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
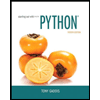
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
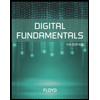
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
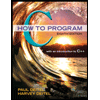
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
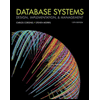
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
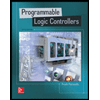
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education