(in java)- In class HashTable implement a hash table and consider the following: (i) Keys are integers (therefore also negative!) and should be stored in the table int[] data. (ii) As a hash function take h(x) = (x · 701) mod 2000. The size of the table is therefore 2000. Be careful when computing the index of a negative key. For example, the index of the key x = −10 is h(−10) = (−7010) mod 2000 = (2000(−4) + 990) mod 2000 = 990. Hence, indices should be non-negative integers between 0 and 1999! (iii) Implement insert, which takes an integer and inserts it into a table. The method returns true, if the insertion is successful. If an element is already in the table, the function insert should return false. (iv) Implement search, which takes an integer and finds it in the table. The method returns true, if the search is successful and false otherwise. (v) Implement delete, which takes an integer and deletes it form the table. The method returns true, if the deletion is successful and false otherwise. (vi) Collision should be solved by chaining. However, not as usually by linked list, but with a hash table implemented in class HashTable2 (combined structure). - In class HashTable2 implement a hash table and consider the following: (i) Keys are integers (therefore also negative!) and should be stored in the table int[] data. (ii) As a hash function take h(x) = (x·53) mod 100. The size of the table is therefore 100. As above be careful to compute the index of a negative key correctly. In this case indices should be non-negative integers between 0 and 99! 1 (iii) Implement insert, which takes an integer and inserts it into a table. The method returns true, if the insertion is successful. If an element is already in the table, the function insert should return false. When inserting an element into a full table, the function insert should return false. (iv) Implement search, which takes an integer and finds it in the table. The method returns true, if the search is successful and false otherwise. (v) Implement delete, which takes an integer and deletes it form the table. The method returns true, if the deletion is successful and false otherwise. (vi) Collision should be solved by open-addressing with linear probing. Be careful when deleting an element. You need a special character to indicate that the element was deleted so that the function search will work correctly. Also, be careful when inserting new element, since it can be inserted into the place indicated with the special character.
(in java)- In class HashTable implement a hash table and consider the following:
(i) Keys are integers (therefore also negative!) and should be stored in the table
int[] data.
(ii) As a hash function take h(x) = (x · 701) mod 2000. The size of the table is
therefore 2000. Be careful when computing the index of a negative key. For
example, the index of the key x = −10 is
h(−10) = (−7010) mod 2000 = (2000(−4) + 990) mod 2000 = 990.
Hence, indices should be non-negative integers between 0 and 1999!
(iii) Implement insert, which takes an integer and inserts it into a table. The
method returns true, if the insertion is successful. If an element is already in
the table, the function insert should return false.
(iv) Implement search, which takes an integer and finds it in the table. The method
returns true, if the search is successful and false otherwise.
(v) Implement delete, which takes an integer and deletes it form the table. The
method returns true, if the deletion is successful and false otherwise.
(vi) Collision should be solved by chaining. However, not as usually by linked list,
but with a hash table implemented in class HashTable2 (combined structure).
- In class HashTable2 implement a hash table and consider the following:
(i) Keys are integers (therefore also negative!) and should be stored in the table
int[] data.
(ii) As a hash function take h(x) = (x·53) mod 100. The size of the table is therefore
100. As above be careful to compute the index of a negative key correctly. In
this case indices should be non-negative integers between 0 and 99!
1
(iii) Implement insert, which takes an integer and inserts it into a table. The
method returns true, if the insertion is successful. If an element is already in
the table, the function insert should return false. When inserting an element
into a full table, the function insert should return false.
(iv) Implement search, which takes an integer and finds it in the table. The method
returns true, if the search is successful and false otherwise.
(v) Implement delete, which takes an integer and deletes it form the table. The
method returns true, if the deletion is successful and false otherwise.
(vi) Collision should be solved by open-addressing with linear probing. Be careful
when deleting an element. You need a special character to indicate that the
element was deleted so that the function search will work correctly. Also,
be careful when inserting new element, since it can be inserted into the place
indicated with the special character.

Step by step
Solved in 4 steps with 4 images

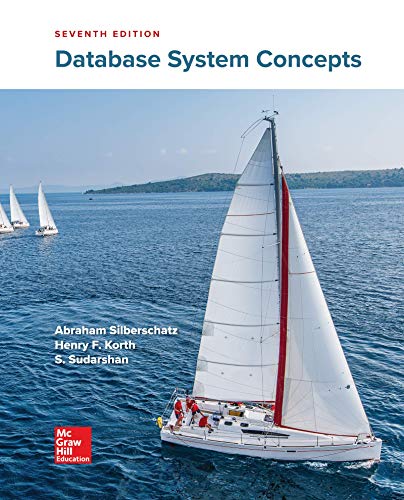
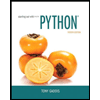
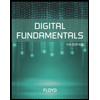
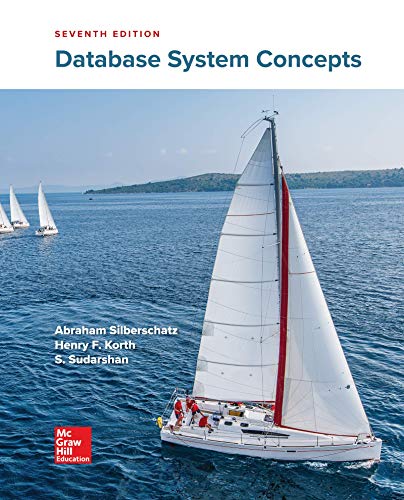
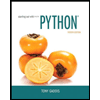
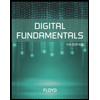
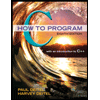
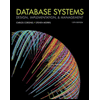
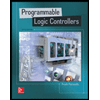