in java : Implement the following class: Class name: Student - where an instance of class Student represents a student. Private member variables: · name, which is a String representing the name of the student · class, which is a String representing the class the student is enrolled in (i.e. CS155) · grade, which is a String representing the grade of the student (i.e. A, B, C, D, F) (they are all-capital-letters) Public member methods: - a constructor () - set name - set class - set grade - get name - get class -get grade Write a test program (main method) that: · Maintains an array studentArray of base type Student Student [] studentArray; · The program will read from the user and store the information of the following 7 (SEVEN) students: Dalia Thomas CS155 A Sarah Johns CS155 A Adam Jack CS155 B Sandra Johnson CS256 A Tim Locks CS155 C Laila Zelle CS265 C Sam Adams CS265 A Helpful hint: You may use a loop to fill the array with the information listed in the table using the following logic for i goes from 0 to 6 and incremented by 1 in each iteration { Create the object of the studentArray[i] Ask user to enter name; Set name of studentArray[i] with name value read from previous line Ask user to enter class Set class of studentArray[i] with class value read from previous line Ask user to enter grade Set grade of stduentArray[i] with grade value read from previous line } . The program will prompt the user to enter a grade and then check the studentArray and PRINTS only those students with matching grade. For example, if the user enters A, the program will search the array studentArray for all the students whose grade is A and display them. Otherwise, the program will display "No students with that grade".
in java :
Implement the following class:
Class name: Student
- where an instance of class Student represents a student.
Private member variables:
· name, which is a String representing the name of the student
· class, which is a String representing the class the student is enrolled in (i.e. CS155)
· grade, which is a String representing the grade of the student (i.e. A, B, C, D, F) (they are all-capital-letters)
Public member methods:
- a constructor ()
- set name
- set class
- set grade
- get name
- get class
-get grade
Write a test program (main method) that:
· Maintains an array studentArray of base type Student
Student [] studentArray;
· The program will read from the user and store the information of the following 7 (SEVEN) students:
Dalia Thomas |
CS155 |
A |
Sarah Johns |
CS155 |
A |
Adam Jack |
CS155 |
B |
Sandra Johnson |
CS256 |
A |
Tim Locks |
CS155 |
C |
Laila Zelle |
CS265 |
C |
Sam Adams |
CS265 |
A |
Helpful hint:
You may use a loop to fill the array with the information listed in the table using the following logic
for i goes from 0 to 6 and incremented by 1 in each iteration
{
Create the object of the studentArray[i]
Ask user to enter name;
Set name of studentArray[i] with name value read from previous line
Ask user to enter class
Set class of studentArray[i] with class value read from previous line
Ask user to enter grade
Set grade of stduentArray[i] with grade value read from previous line
}
. The program will prompt the user to enter a grade and then check the studentArray and PRINTS only those students with matching grade. For example, if the user enters A, the program will search the array studentArray for all the students whose grade is A and display them. Otherwise, the program will display "No students with that grade".
Test your program on grade <A>.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 6 images

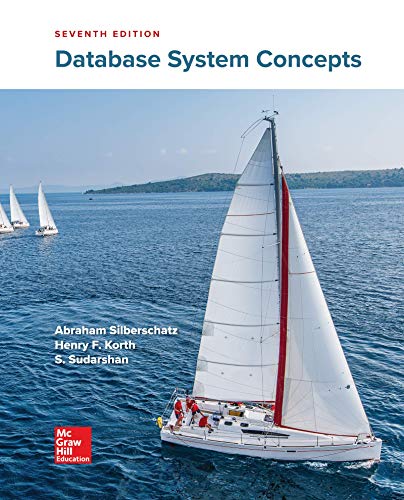
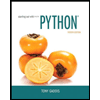
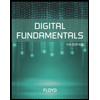
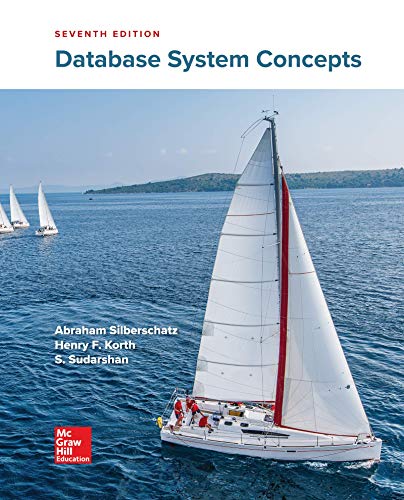
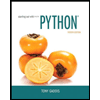
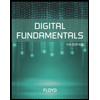
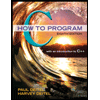
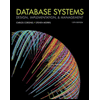
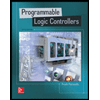