In C write a grading program as follows. - Ask the user for the number of students and store it in an integer variable. - Create an array of floats with four rows and columns equal to the number of students stored earlier. - Initialize the array to zeros. Create a menu with the following options (use a do-while loop and repeatedly display the menu): A or a to add student info one student at a time T or t to display class average for homework S or s to display class average for quizzes B or b to display class average for exams Z or z to exit program (program repeats until this exit command is entered)
In C write a grading program as follows. - Ask the user for the number of students and store it in an integer variable. - Create an array of floats with four rows and columns equal to the number of students stored earlier. - Initialize the array to zeros. Create a menu with the following options (use a do-while loop and repeatedly display the menu): A or a to add student info one student at a time T or t to display class average for homework S or s to display class average for quizzes B or b to display class average for exams Z or z to exit program (program repeats until this exit command is entered)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In C write a grading
- Ask the user for the number of students and store it in an integer variable.
- Create an array of floats with four rows and columns equal to the number of students stored
earlier.
- Initialize the array to zeros.
Create a menu with the following options (use a do-while loop and repeatedly display the menu):
A or a to add student info one student at a time
T or t to display class average for homework
S or s to display class average for quizzes
B or b to display class average for exams
Z or z to exit program (program repeats until this exit command is entered)
Expert Solution

Step 1: Step 1
Here's a C program that implements the grading program as described:
1#include <stdio.h>
2
3int main() {
4 int numStudents;
5
6 printf("Enter the number of students: ");
7 scanf("%d", &numStudents);
8
9 float grades[numStudents][4]; // Create a 2D array for student grades
10 for (int i = 0; i < numStudents; i++) {
11 for (int j = 0; j < 4; j++) {
12 grades[i][j] = 0.0; // Initialize the array to zeros
13 }
14 }
15
16 char choice;
17 do {
18 printf("\nMenu:\n");
19 printf("A or a: Add student info\n");
20 printf("T or t: Display class average for homework\n");
21 printf("S or s: Display class average for quizzes\n");
22 printf("B or b: Display class average for exams\n");
23 printf("Z or z: Exit program\n");
24 printf("Enter your choice: ");
25 scanf(" %c", &choice);
26
27 switch (choice) {
28 case 'A':
29 case 'a':
30 // Add student info
31 printf("Enter student ID (0-%d): ", numStudents - 1);
32 int studentID;
33 scanf("%d", &studentID);
34 if (studentID >= 0 && studentID < numStudents) {
35 printf("Enter homework, quizzes, and exams grades (space-separated): ");
36 float hw, quiz, exam;
37 scanf("%f %f %f", &hw, &quiz, &exam);
38 grades[studentID][0] = hw;
39 grades[studentID][1] = quiz;
40 grades[studentID][2] = exam;
41 printf("Student info added.\n");
42 } else {
43 printf("Invalid student ID.\n");
44 }
45 break;
46
47 case 'T':
48 case 't':
49 // Display class average for homework
50 float hwTotal = 0.0;
51 for (int i = 0; i < numStudents; i++) {
52 hwTotal += grades[i][0];
53 }
54 printf("Class average for homework: %.2f\n", hwTotal / numStudents);
55 break;
56
57 case 'S':
58 case 's':
59 // Display class average for quizzes
60 float quizTotal = 0.0;
61 for (int i = 0; i < numStudents; i++) {
62 quizTotal += grades[i][1];
63 }
64 printf("Class average for quizzes: %.2f\n", quizTotal / numStudents);
65 break;
66
67 case 'B':
68 case 'b':
69 // Display class average for exams
70 float examTotal = 0.0;
71 for (int i = 0; i < numStudents; i++) {
72 examTotal += grades[i][2];
73 }
74 printf("Class average for exams: %.2f\n", examTotal / numStudents);
75 break;
76
77 case 'Z':
78 case 'z':
79 // Exit program
80 printf("Exiting program.\n");
81 break;
82
83 default:
84 printf("Invalid choice. Please try again.\n");
85 }
86 } while (choice != 'Z' && choice != 'z');
87
88 return 0;
89}
90
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
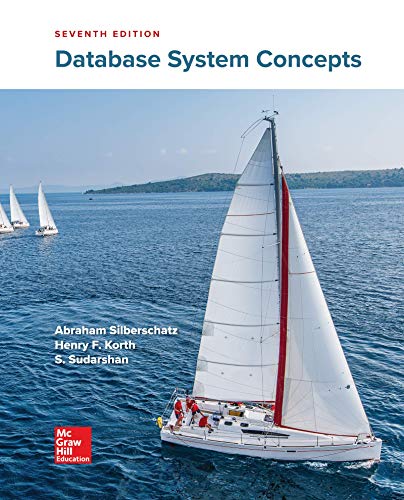
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
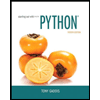
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
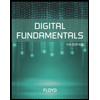
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
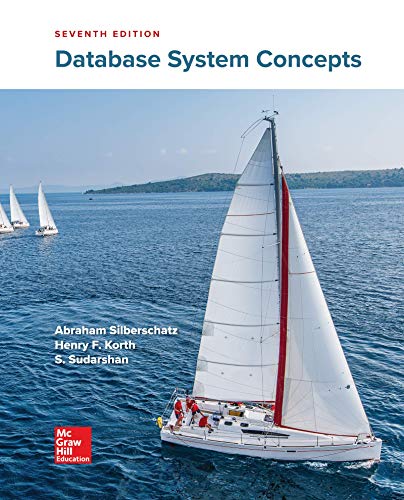
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
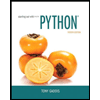
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
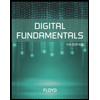
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
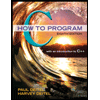
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
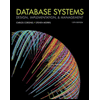
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
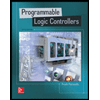
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education