In a file named Octagon.java write an Octagon class that extends GeometricObject and implements the Comparable and Cloneable interfaces. Assume that all eight sides of an octagon are of equal length. Write methods for computing the area and the perimeter. The perimeter is obviously 8 times the side length. The area can be computed using the following formula: area = (2 + 4 / Math.sqrt(2)) * side * side In addition to a side data field, every Octagon should have an isClone data field of type boolean. By default the isClone value is false. However, if an Octagon was created by your clone method, then the isClone value should be true (i.e., the clone() method should set the isClone value to true of the newly created Octagon.) Write a toString method in the Octagon class that returns a string containing the side length, the perimeter, the area, and the value of wasCloned. Write a test program (TestOctagon.java) that creates an Octagon object with side value 5. Clone the object. Then display the original object and the clone. Finally, display the value that you get when you compare the two objects using the compareTo method. Your output should follow this general format, which oct1 and oct2 are the names of the reference variables. oct1: Octagon with side = 5.0; perimeter = 40.0; area = 120.71067811865476; isClone = false oct2: Octagon with side = 5.0; perimeter = 40.0; area = 120.71067811865476; isClone = true oct1.compareTo(oct2): 0 public abstract class GeometricObject { private String color = "white"; private boolean filled; private java.util.Date dateCreated; /** Construct a default geometric object */ protected GeometricObject() { dateCreated = new java.util.Date(); } /** Construct a geometric object with color and filled value */ protected GeometricObject(String color, boolean filled) { dateCreated = new java.util.Date(); this.color = color; this.filled = filled; } /** Return color */ public String getColor() { return color; } /** Set a new color */ public void setColor(String color) { this.color = color; } /** Return filled. Since filled is boolean, * the get method is named isFilled */ public boolean isFilled() { return filled; } /** Set a new filled */ public void setFilled(boolean filled) { this.filled = filled; } /** Get dateCreated */ public java.util.Date getDateCreated() { return dateCreated; } /** Return a string representation of this object */ public String toString() { return "created on " + dateCreated + "\ncolor: " + color + " and filled: " + filled; } /** Abstract method getArea */ public abstract double getArea(); /** Abstract method getPerimeter */ public abstract double getPerimeter(); }
In a file named Octagon.java write an Octagon class that extends GeometricObject and implements the Comparable and Cloneable interfaces. Assume that all eight sides of an octagon are of equal length. Write methods for computing the area and the perimeter. The perimeter is obviously 8 times the side length. The area can be computed using the following formula:
area = (2 + 4 / Math.sqrt(2)) * side * side
In addition to a side data field, every Octagon should have an isClone data field of type boolean. By default the isClone value is false. However, if an Octagon was created by your clone method, then the isClone value should be true (i.e., the clone() method should set the isClone value to true of the newly created Octagon.) Write a toString method in the Octagon class that returns a string containing the side length, the perimeter, the area, and the value of wasCloned. Write a test program (TestOctagon.java) that creates an Octagon object with side value 5. Clone the object. Then display the original object and the clone. Finally, display the value that you get when you compare the two objects using the compareTo method. Your output should follow this general format, which oct1 and oct2 are the names of the reference variables.
oct1: Octagon with side = 5.0; perimeter = 40.0; area = 120.71067811865476;
isClone = false
oct2: Octagon with side = 5.0; perimeter = 40.0; area = 120.71067811865476;
isClone = true
oct1.compareTo(oct2): 0
public abstract class GeometricObject { private String color = "white"; private boolean filled; private java.util.Date dateCreated; /** Construct a default geometric object */ protected GeometricObject() { dateCreated = new java.util.Date(); } /** Construct a geometric object with color and filled value */ protected GeometricObject(String color, boolean filled) { dateCreated = new java.util.Date(); this.color = color; this.filled = filled; } /** Return color */ public String getColor() { return color; } /** Set a new color */ public void setColor(String color) { this.color = color; } /** Return filled. Since filled is boolean, * the get method is named isFilled */ public boolean isFilled() { return filled; } /** Set a new filled */ public void setFilled(boolean filled) { this.filled = filled; } /** Get dateCreated */ public java.util.Date getDateCreated() { return dateCreated; } /** Return a string representation of this object */ public String toString() { return "created on " + dateCreated + "\ncolor: " + color + " and filled: " + filled; } /** Abstract method getArea */ public abstract double getArea(); /** Abstract method getPerimeter */ public abstract double getPerimeter(); }

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

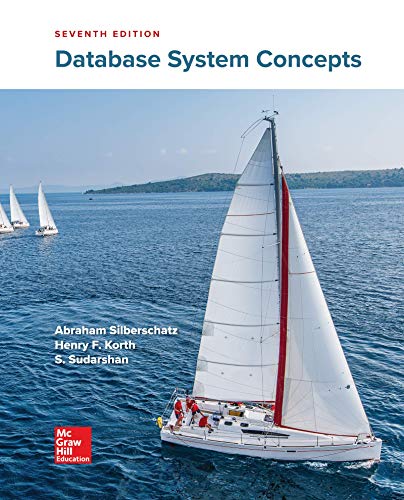
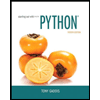
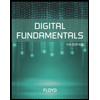
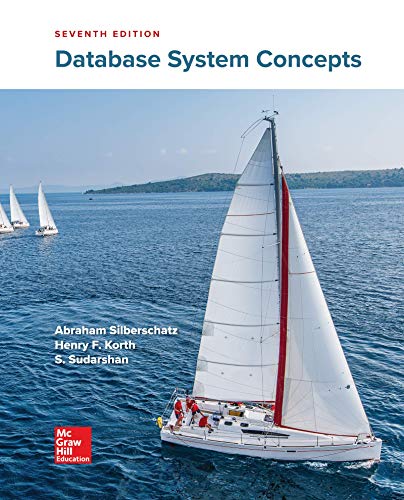
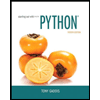
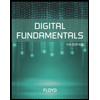
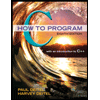
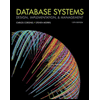
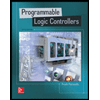