```python import numpy as np from scipy.integrate import odeint def model(y, t, m, g): h, v = y dhdt = v dvdt = (m*g - 5*v - 120*h) / m return [dhdt, dvdt] t = np.linspace(0, 10, 1000) # time grid m = 1.0 # mass h0 = 100.0 # initial height v0 = 0.0 # initial velocity g = 9.8 # acceleration due to gravity # solve ODE y0 = [h0, v0] sol = odeint(model, y0, t, args=(m, g)) # extract h(t) and v(t) h = sol[:, 0] v = sol[:, 1] ``` ### Explanation This code snippet is designed to simulate the motion of an object under the influence of gravity and a damping force. Here's a breakdown of the components: 1. **Imports**: - `numpy` as `np` for numerical operations. - `odeint` from `scipy.integrate` to solve ordinary differential equations (ODEs). 2. **Function Definition**: - `model(y, t, m, g)`: Defines the differential equations governing the system. - `y` is the state vector `[h, v]`, where `h` is height and `v` is velocity. - `t` is time. - `m` is mass. - `g` is gravitational acceleration. - The function returns `[dhdt, dvdt]`, which are the derivatives of height and velocity. 3. **Parameters and Initialization**: - `t`: A time grid from 0 to 10 seconds with 1000 points. - `m`: Mass of the object (1.0 kg). - `h0`: Initial height (100 meters). - `v0`: Initial velocity (0 m/s). - `g`: Gravitational acceleration (9.8 m/s²). 4. **Solving the ODE**: - `y0`: Initial conditions `[h0, v0]`. - `sol`: Solution of the ODE system using `odeint` with `model`, initial
```python import numpy as np from scipy.integrate import odeint def model(y, t, m, g): h, v = y dhdt = v dvdt = (m*g - 5*v - 120*h) / m return [dhdt, dvdt] t = np.linspace(0, 10, 1000) # time grid m = 1.0 # mass h0 = 100.0 # initial height v0 = 0.0 # initial velocity g = 9.8 # acceleration due to gravity # solve ODE y0 = [h0, v0] sol = odeint(model, y0, t, args=(m, g)) # extract h(t) and v(t) h = sol[:, 0] v = sol[:, 1] ``` ### Explanation This code snippet is designed to simulate the motion of an object under the influence of gravity and a damping force. Here's a breakdown of the components: 1. **Imports**: - `numpy` as `np` for numerical operations. - `odeint` from `scipy.integrate` to solve ordinary differential equations (ODEs). 2. **Function Definition**: - `model(y, t, m, g)`: Defines the differential equations governing the system. - `y` is the state vector `[h, v]`, where `h` is height and `v` is velocity. - `t` is time. - `m` is mass. - `g` is gravitational acceleration. - The function returns `[dhdt, dvdt]`, which are the derivatives of height and velocity. 3. **Parameters and Initialization**: - `t`: A time grid from 0 to 10 seconds with 1000 points. - `m`: Mass of the object (1.0 kg). - `h0`: Initial height (100 meters). - `v0`: Initial velocity (0 m/s). - `g`: Gravitational acceleration (9.8 m/s²). 4. **Solving the ODE**: - `y0`: Initial conditions `[h0, v0]`. - `sol`: Solution of the ODE system using `odeint` with `model`, initial
Advanced Engineering Mathematics
10th Edition
ISBN:9780470458365
Author:Erwin Kreyszig
Publisher:Erwin Kreyszig
Chapter2: Second-order Linear Odes
Section: Chapter Questions
Problem 1RQ
Related questions
Question
below is a python code for solving a system of non-homogeneous linear
![```python
import numpy as np
from scipy.integrate import odeint
def model(y, t, m, g):
h, v = y
dhdt = v
dvdt = (m*g - 5*v - 120*h) / m
return [dhdt, dvdt]
t = np.linspace(0, 10, 1000) # time grid
m = 1.0 # mass
h0 = 100.0 # initial height
v0 = 0.0 # initial velocity
g = 9.8 # acceleration due to gravity
# solve ODE
y0 = [h0, v0]
sol = odeint(model, y0, t, args=(m, g))
# extract h(t) and v(t)
h = sol[:, 0]
v = sol[:, 1]
```
### Explanation
This code snippet is designed to simulate the motion of an object under the influence of gravity and a damping force. Here's a breakdown of the components:
1. **Imports**:
- `numpy` as `np` for numerical operations.
- `odeint` from `scipy.integrate` to solve ordinary differential equations (ODEs).
2. **Function Definition**:
- `model(y, t, m, g)`: Defines the differential equations governing the system.
- `y` is the state vector `[h, v]`, where `h` is height and `v` is velocity.
- `t` is time.
- `m` is mass.
- `g` is gravitational acceleration.
- The function returns `[dhdt, dvdt]`, which are the derivatives of height and velocity.
3. **Parameters and Initialization**:
- `t`: A time grid from 0 to 10 seconds with 1000 points.
- `m`: Mass of the object (1.0 kg).
- `h0`: Initial height (100 meters).
- `v0`: Initial velocity (0 m/s).
- `g`: Gravitational acceleration (9.8 m/s²).
4. **Solving the ODE**:
- `y0`: Initial conditions `[h0, v0]`.
- `sol`: Solution of the ODE system using `odeint` with `model`, initial](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6d0ee2cb-cfe2-4eb9-a097-d38324436758%2F036e5429-9dde-4b57-9db9-bfacc4330cdf%2Fxrn4ko_processed.jpeg&w=3840&q=75)
Transcribed Image Text:```python
import numpy as np
from scipy.integrate import odeint
def model(y, t, m, g):
h, v = y
dhdt = v
dvdt = (m*g - 5*v - 120*h) / m
return [dhdt, dvdt]
t = np.linspace(0, 10, 1000) # time grid
m = 1.0 # mass
h0 = 100.0 # initial height
v0 = 0.0 # initial velocity
g = 9.8 # acceleration due to gravity
# solve ODE
y0 = [h0, v0]
sol = odeint(model, y0, t, args=(m, g))
# extract h(t) and v(t)
h = sol[:, 0]
v = sol[:, 1]
```
### Explanation
This code snippet is designed to simulate the motion of an object under the influence of gravity and a damping force. Here's a breakdown of the components:
1. **Imports**:
- `numpy` as `np` for numerical operations.
- `odeint` from `scipy.integrate` to solve ordinary differential equations (ODEs).
2. **Function Definition**:
- `model(y, t, m, g)`: Defines the differential equations governing the system.
- `y` is the state vector `[h, v]`, where `h` is height and `v` is velocity.
- `t` is time.
- `m` is mass.
- `g` is gravitational acceleration.
- The function returns `[dhdt, dvdt]`, which are the derivatives of height and velocity.
3. **Parameters and Initialization**:
- `t`: A time grid from 0 to 10 seconds with 1000 points.
- `m`: Mass of the object (1.0 kg).
- `h0`: Initial height (100 meters).
- `v0`: Initial velocity (0 m/s).
- `g`: Gravitational acceleration (9.8 m/s²).
4. **Solving the ODE**:
- `y0`: Initial conditions `[h0, v0]`.
- `sol`: Solution of the ODE system using `odeint` with `model`, initial
AI-Generated Solution
Unlock instant AI solutions
Tap the button
to generate a solution
Recommended textbooks for you
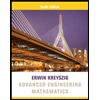
Advanced Engineering Mathematics
Advanced Math
ISBN:
9780470458365
Author:
Erwin Kreyszig
Publisher:
Wiley, John & Sons, Incorporated
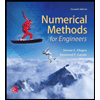
Numerical Methods for Engineers
Advanced Math
ISBN:
9780073397924
Author:
Steven C. Chapra Dr., Raymond P. Canale
Publisher:
McGraw-Hill Education
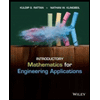
Introductory Mathematics for Engineering Applicat…
Advanced Math
ISBN:
9781118141809
Author:
Nathan Klingbeil
Publisher:
WILEY
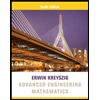
Advanced Engineering Mathematics
Advanced Math
ISBN:
9780470458365
Author:
Erwin Kreyszig
Publisher:
Wiley, John & Sons, Incorporated
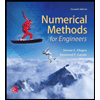
Numerical Methods for Engineers
Advanced Math
ISBN:
9780073397924
Author:
Steven C. Chapra Dr., Raymond P. Canale
Publisher:
McGraw-Hill Education
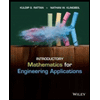
Introductory Mathematics for Engineering Applicat…
Advanced Math
ISBN:
9781118141809
Author:
Nathan Klingbeil
Publisher:
WILEY
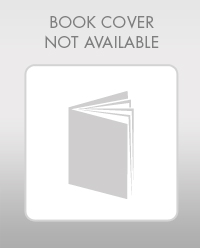
Mathematics For Machine Technology
Advanced Math
ISBN:
9781337798310
Author:
Peterson, John.
Publisher:
Cengage Learning,
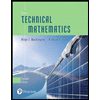
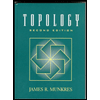