import javafx.application.Application; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.geometry.Pos; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.layout.BorderPane; import javafx.scene.layout.HBox; import javafx.scene.layout.StackPane; import javafx.scene.paint.Color; import javafx.scene.shape.Circle; public class Main extends Application { private CirclePane circlePane=new CirclePane(); @Override public void start(Stage primaryStage) { //holds two bottoms in an HBox HBox hBox=new HBox(); hBox.setSpacing(10); hBox.setAlignment(Pos.CENTER); Button btEnlarge = new Button("Enlarge"); Button btShrink = new Button("Shrink"); hBox.getChildren().add(btEnlarge); hBox.getChildren().add(btShrink); //create and register the handler btEnlarge.setOnAction(new EnlargeHandler()); btShrink.setOnAction(new ShrinkHandler()); BorderPane borderPane=new BorderPane(); borderPane.setCenter(circlePane); borderPane.setBottom(hBox); BorderPane.setAlignment(hBox,Pos.CENTER); //create a scene and place it on the stage Scene scene =new Scene(borderPane,200,150); primaryStage.setTitle("Control Circle"); primaryStage.setScene(scene); primaryStage.show(); } class EnlargeHandler implements EventHandler{ @Override public void handle(ActionEvent e) { circlePane.enlarge(); } } class ShrinkHandler implements EventHandler{ @Override public void handle(ActionEvent e) { circlePane.shrink(); } } } class CirclePane extends StackPane{ private Circle circle = new Circle(); public CirclePane() { getChildren().add(circle); circle.setStroke(Color.BLACK); circle.setFill(Color.WHITE); } public void enlarge() { circle.setRadius(circle.getRadius()+2); } public void shrink() { circle.setRadius(circle.getRadius()>2? circle.getRadius()-2 :circle.getRadius()); } public static void main(String[] args) { launch(args);// } }
help with a code
package application;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Pos;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.HBox;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
public class Main extends Application {
private CirclePane circlePane=new CirclePane();
@Override
public void start(Stage primaryStage) {
//holds two bottoms in an HBox
HBox hBox=new HBox();
hBox.setSpacing(10);
hBox.setAlignment(Pos.CENTER);
Button btEnlarge = new Button("Enlarge");
Button btShrink = new Button("Shrink");
hBox.getChildren().add(btEnlarge);
hBox.getChildren().add(btShrink);
//create and register the handler
btEnlarge.setOnAction(new EnlargeHandler());
btShrink.setOnAction(new ShrinkHandler());
BorderPane borderPane=new BorderPane();
borderPane.setCenter(circlePane);
borderPane.setBottom(hBox);
BorderPane.setAlignment(hBox,Pos.CENTER);
//create a scene and place it on the stage
Scene scene =new Scene(borderPane,200,150);
primaryStage.setTitle("Control Circle");
primaryStage.setScene(scene);
primaryStage.show();
}
class EnlargeHandler implements EventHandler<ActionEvent>{
@Override
public void handle(ActionEvent e) {
circlePane.enlarge();
}
}
class ShrinkHandler implements EventHandler<ActionEvent>{
@Override
public void handle(ActionEvent e) {
circlePane.shrink();
}
}
}
class CirclePane extends StackPane{
private Circle circle = new Circle();
public CirclePane() {
getChildren().add(circle);
circle.setStroke(Color.BLACK);
circle.setFill(Color.WHITE);
}
public void enlarge() {
circle.setRadius(circle.getRadius()+2);
}
public void shrink() {
circle.setRadius(circle.getRadius()>2?
circle.getRadius()-2 :circle.getRadius());
}
public static void main(String[] args) {
launch(args);//
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

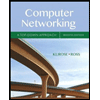
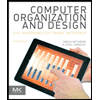
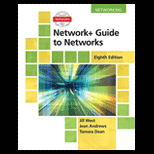
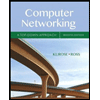
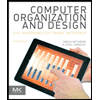
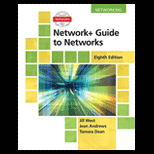
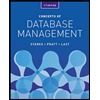
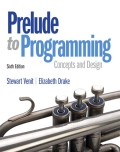
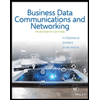