Implement the class Student. This class should contain 4 properties: firstName a string which represents the first name of the student lastName a string which represents the last name of the student age an integer which represents the age of the student isMinor a boolean value which will be true if the age is less than 18 and false otherwise. this will automatically be set every time an instance of this class is created and will automatically be updated appropriately every time the age of the student increases. This class should have a constructor with the following signature: - public Student(String firstName, String lastName, int age) Additionally, it should have the following methods: 1. getName Returns the name of the student in the following format: "{lastName}, {firstName}" 2. increaseAge Increases the age by one (1). This returns nothing. 3. toString Returns the details of the student in a single String. The format is: {name_in_the_same_format_as_getName} - {age} - {"minor" or "adult"} Example: Stan, Mark Jon - 25 - adult Reyes, Rose- 15 - minor Main.java import java.lang.*; import java.util.*; class Main { public static void main(String args[]) { Student student = new Student("First", "Last", 16); } } Student.java public class Student { String firstName; String lastName; int age; bool isMinor; public Student(String firstName, String lastName, int age) { this.firstName = firstName; this.lastName = lastName; this.age = age; } public void getName(){ System.out.println("{" + lastName + "}, {" + firstName + "}"); } public void increaseAge(){ System.out.println(age + 1); } public String toString(){ } }
JAVA- Improve my code
Implement the class Student. This class should contain 4 properties:
-
firstName
- a string which represents the first name of the student
-
lastName
- a string which represents the last name of the student
-
age
- an integer which represents the age of the student
-
isMinor
- a boolean value which will be true if the age is less than 18 and false otherwise.
- this will automatically be set every time an instance of this class is created and will automatically be updated appropriately every time the age of the student increases.
This class should have a constructor with the following signature:
- public Student(String firstName, String lastName, int age)
Additionally, it should have the following methods:
1. getName
- Returns the name of the student in the following format: "{lastName}, {firstName}"
2. increaseAge
- Increases the age by one (1). This returns nothing.
3. toString
- Returns the details of the student in a single String. The format is: {name_in_the_same_format_as_getName} - {age} - {"minor" or "adult"}
-
Example:
- Stan, Mark Jon - 25 - adult
- Reyes, Rose- 15 - minor
Main.java
import java.lang.*;
import java.util.*;
class Main {
public static void main(String args[]) {
Student student = new Student("First", "Last", 16);
}
}
Student.java
public class Student {
String firstName;
String lastName;
int age;
bool isMinor;
public Student(String firstName, String lastName, int age) {
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
}
public void getName(){
System.out.println("{" + lastName + "}, {" + firstName + "}");
}
public void increaseAge(){
System.out.println(age + 1);
}
public String toString(){
}
}

Step by step
Solved in 3 steps with 2 images

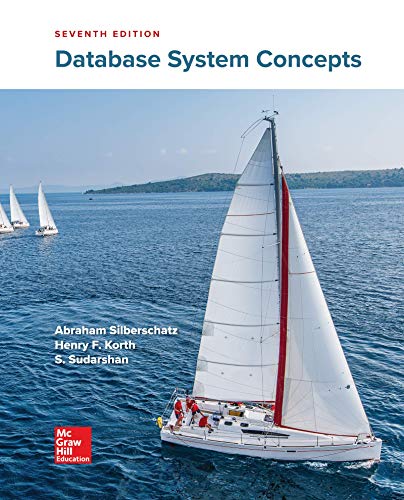
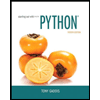
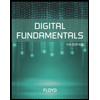
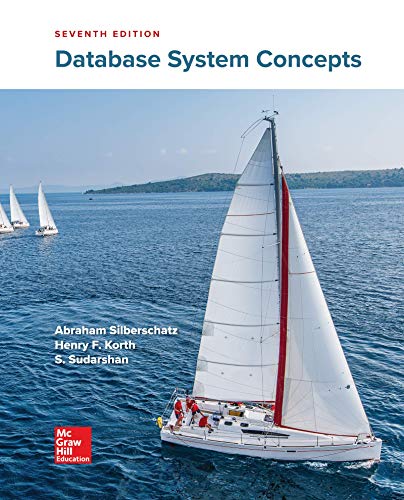
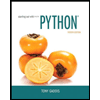
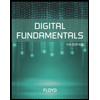
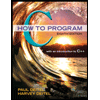
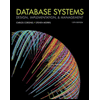
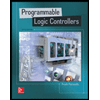