Implement the Binary Search Tree in Python class BTNode: '''Binary Tree Node class - do not modify''' def __init__(self, value, left, right): '''Stores a reference to the value, left child node, and right child node. If no left or right child, attributes should be None.''' self.value = value self.left = left self.right = right class BST: def __init__(self): # reference to root node - do not modify self.root = None def insert(self, value): '''Takes a numeric value as argument. Inserts value into tree, maintaining correct ordering. Returns nothing.''' pass def search(self, value): '''Takes a numeric value as argument. Returns True if its in the tree, false otherwise.''' pass def height(self): '''Returns the height of the tree. A tree with 0 or 1 nodes has a height of 0.''' pass def preorder(self): '''Returns a list of the values in the tree, in pre-order order.''' pass
Implement the Binary Search Tree in Python
class BTNode:
'''Binary Tree Node class - do not modify'''
def __init__(self, value, left, right):
'''Stores a reference to the value, left child node, and right child node. If no left or right child, attributes should be None.'''
self.value = value
self.left = left
self.right = right
class BST:
def __init__(self):
# reference to root node - do not modify
self.root = None
def insert(self, value):
'''Takes a numeric value as argument.
Inserts value into tree, maintaining correct ordering.
Returns nothing.'''
pass
def search(self, value):
'''Takes a numeric value as argument.
Returns True if its in the tree, false otherwise.'''
pass
def height(self):
'''Returns the height of the tree.
A tree with 0 or 1 nodes has a height of 0.'''
pass
def preorder(self):
'''Returns a list of the values in the tree,
in pre-order order.'''
pass

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

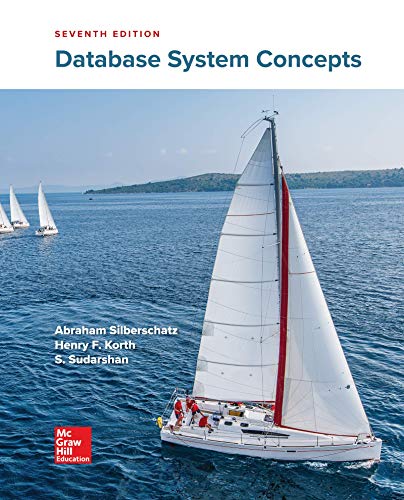
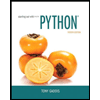
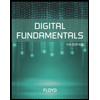
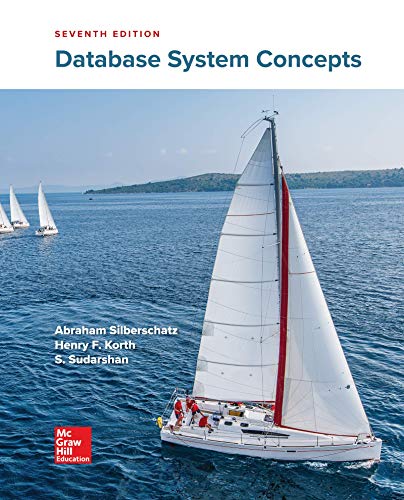
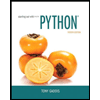
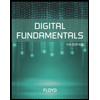
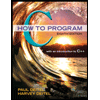
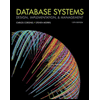
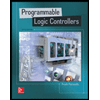