Implement a program that implements a sorted list using dynamic allocated arrays. Use C++ and do not use Standard Library Template. (Implement the steps in each bullet point and show correct output) Do not post previous solution but a original one that meets the OUTPUTS listed below! DataFile.txt contains the information of poker cards. C: clubs(lowest),D: diamonds, H: hearts, S:spades(highest) 2 (lowest), 3, 4, 5, 6, 7, 8, 9, 10, J, Q, K, A No Joker cards Any C cards are lower than any D cards. DataFile Content
Implement a
DataFile.txt contains the information of poker cards.
- C: clubs(lowest),D: diamonds, H: hearts, S:spades(highest)
- 2 (lowest), 3, 4, 5, 6, 7, 8, 9, 10, J, Q, K, A
- No Joker cards
- Any C cards are lower than any D cards.
DataFile Content(You can write the file specification into your program.):
H4,C8,HJ,C9,D10,D5,DK,D2,S7,DJ,H3,H6,S10,HK,DQ,C2,CJ,C4,CQ,D8,C3,SA,S2,HQ,S8,C6,D9,S3,SQ,C5,S4,H5,SJ,D3,H8,CK,S6,D7,S9,H2,CA,C7,H7,DA,D4,H9,D6,HA,H10,S5,C10
H4, D5, HK, D2
H4, HK, SK
C9,C10
For examples, DJ means J of Diamonds;H7 means 7 of hearts.
Take into account each bullet point for output!
- Create a list by dynamic allocated array and set the size to 20
- Read the first 20 cards in the first line of the file, the put them one by one into the list by implementing and using putItem(). The list must be kept sorted in ascending order. Then print out all the cards in the list in one line separating by commas.
- Then delete the cards indicated in the second line of the file by using deleteItem()
- Then print out all the cards in the list in one line separating by commas.
- Then put the items in the third line in to the list. Must use putItem()
Then print out all the cards in the listin one line separating by commas.
- Search the current list for the elements in the list.
Then output the result as the follows. Yes or No depends on whether the card exists in
the current list. Must implement and use getItem()
C9 NO, C10 YES( include in output)
- A printAll() function should be defined and called in order to output all the contents in
the list.
- A compareTo() function must be defined and used to compare which card is greater
Copy following to test with your own .txt file exactly as it follows with commas!
- Output must sort the cards as specified here:
- C: clubs(lowest),D: diamonds, H: hearts, S:spades(highest)
- 2 (lowest), 3, 4, 5, 6, 7, 8, 9, 10, J, Q, K, A
- No Joker cards
- Any C cards are lower than any D cards.
- Must have implemented each bullet point above
- OUTPUT MOST HAVE CARDS SORTED FOR FIRST LINE OF OUTPUT
- SECOND LINE SHOULD HAVE DELETED THE CARDS SPECIFIED IN SECOND LINE OF .TXT FILE.
- THIRD LINE OF OUTPUT SHOULD BE THE CARDS LISTED IN THIRD LINE OF .TXT FILE TO BE ADDED INTO THE SORTED LIST OF CARDS
- FOURTH LINE OF OUTPUT NEEDS TO PRINT WHETHER OR NOT THE CARDS LISTED IN THE LAST LINE OF THE . TXT FILE ARE THERE!
H4,C8,HJ,C9,D10,D5,DK,D2,S7,DJ,H3,H6,S10,HK,DQ,C2,CJ,C4,CQ,D8,C3,SA,S2,HQ,S8,C6,D9,S3,SQ,C5,S4,H5,SJ,D3,H8,CK,S6,D7,S9,H2,CA,C7,H7,DA,D4,H9,D6,HA,H10,S5,C10
H4, D5, HK, D2
H4, HK, SK
C9,C10

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

The output looks great! Although, in the text file, you did not include ;
H4,C8,HJ,C9,D10,D5,DK,D2,S7,DJ,H3,H6,S10,HK,DQ,C2,CJ,C4,CQ,D8,C3,SA,S2,HQ,S8,C6,D9,S3,SQ,C5,S4,H5,SJ,D3,H8,CK,S6,D7,S9,H2,CA,C7,H7,DA,D4,H9,D6,HA,H10,S5,C10
All into the first line of output. I also noticed that the second line of output has some errors as it does not delete all of the other cards listed in the second line of the text file. Can you test it with more data as I added in the follow up?
Must have met the requirements for output:
1.Then delete the cards indicated in the second line of the file by using deleteItem()
2.Then print out all the cards in the list in one line separating by commas.
3. Then put the items in the third line in to the list. Must use putItem()
Don't reject please, it just needs to meet those requirements and show in the output!
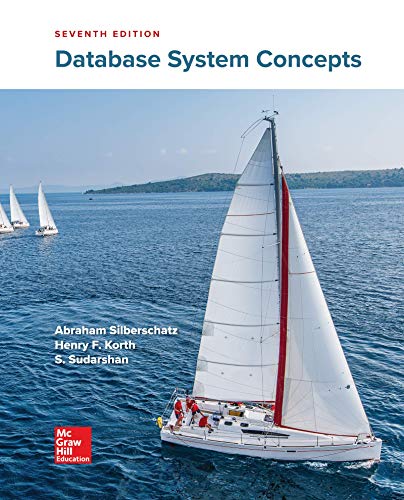
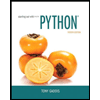
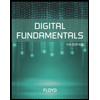
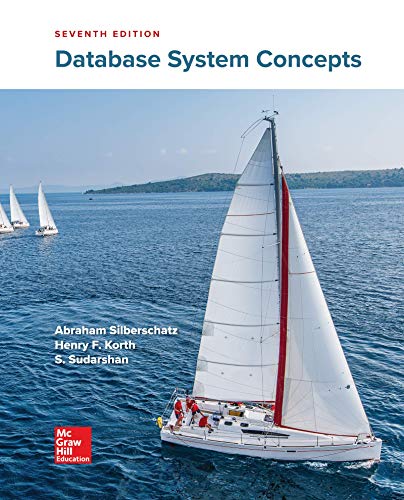
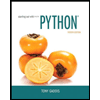
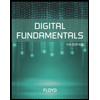
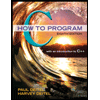
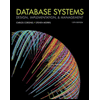
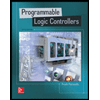