Implement a function called index_of_min with two parameters, l and start_index, which computes the index of the minimum of the sublist starting at index start_index. Do not use methods from the library such as min(), sort() etc. Implement a function called selection_sort which sorts the input list (in place). This function must use index_of_min and must not use use methods from the library such as min(), sort() etc.
slecetion sort (python)
Selection sort is a sorting
Selection sort divides the input list into two parts: a sublist of sorted items (left part) and a sublist of still unsorted items (right part). Initially, the sorted sublist is empty and the whole input consists of the unsorted sublist. To fill the sorted sublist, the algorithm computes the (index of) the minimum of the unsorted sublist and swaps the first unsorted element and the minimum element (if the minimum is already the first element, nothing happens). Afterward, the sorted sublist is one bigger. This continues until the unsorted sublist is empty and the entire input is sorted.
Example:
Sorted sublist | Unsorted sublist | Least element in unsorted list |
() | (11, 25, 12, 22, 64) | 11 |
(11) | (25, 12, 22, 64) | 12 |
(11, 12) | (25, 22, 64) | 22 |
(11, 12, 22) | (25, 64) | 25 |
(11, 12, 22, 25) | (64) | 64 |
(11, 12, 22, 25, 64) | () |
Implement this algorithm.
- Implement a function called index_of_min with two parameters, l and start_index, which computes the index of the minimum of the sublist starting at index start_index. Do not use methods from the library such as min(), sort() etc.
- Implement a function called selection_sort which sorts the input list (in place). This function must use index_of_min and must not use use methods from the library such as min(), sort() etc.

Step by step
Solved in 3 steps with 2 images

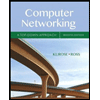
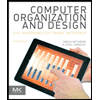
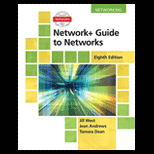
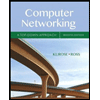
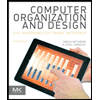
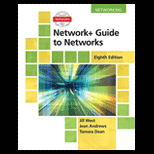
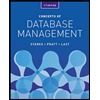
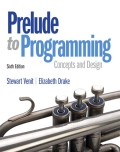
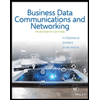