I'm trying to get functions to run concurrently but currently it's running one child first then running the second child. Need them to run concurrently not sequentially. So I need child A to complete a standard Collatz, then I need child B to do the collatz but the initial number is added 6. But with the code below, it runs child A first then completes, then child2 begins. I need both child A and childB to start at the same time #include #include #include #include #include int main() { int number = 0; printf("Enter a number between 0-40\n"); scanf("%d", &number); if (number < 0 || number > 40){ printf("That is not a valid entry, Try again"); scanf("%d", &number); } int pid = fork(); if (pid == 0) { printf("Initial Val1: %d", number); while (number > 1){ if (number % 2 == 0){ number = number / 2; printf("\nFrom child1 %d", number); } else { number = (number * 3) + 1; printf("\nFrom child1 %d", number); } } printf("\nChild1 Done!\n"); } else { if(pid != 0) { pid = fork(); } number = number + 6; if(pid == 0) { printf("Initial Val2: %d", number); while (number > 1){ if (number % 2 == 0){ number = number / 2; printf("\nFrom child2 %d", number); } else { number = (number * 3) + 1; printf("\nFrom child2 %d", number); } } printf("\nChild2 Done!\n"); } wait(NULL); wait(NULL); printf("\nParent: All Children Complete"); } return 0; }
I'm trying to get functions to run concurrently but currently it's running one child first then running the second child. Need them to run concurrently not sequentially. So I need child A to complete a standard Collatz, then I need child B to do the collatz but the initial number is added 6. But with the code below, it runs child A first then completes, then child2 begins. I need both child A and childB to start at the same time
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
int main() {
int number = 0;
printf("Enter a number between 0-40\n");
scanf("%d", &number);
if (number < 0 || number > 40){
printf("That is not a valid entry, Try again");
scanf("%d", &number);
}
int pid = fork();
if (pid == 0) {
printf("Initial Val1: %d", number);
while (number > 1){
if (number % 2 == 0){
number = number / 2;
printf("\nFrom child1 %d", number);
}
else {
number = (number * 3) + 1;
printf("\nFrom child1 %d", number);
}
}
printf("\nChild1 Done!\n");
}
else {
if(pid != 0) {
pid = fork();
}
number = number + 6;
if(pid == 0) {
printf("Initial Val2: %d", number);
while (number > 1){
if (number % 2 == 0){
number = number / 2;
printf("\nFrom child2 %d", number);
}
else {
number = (number * 3) + 1;
printf("\nFrom child2 %d", number);
}
}
printf("\nChild2 Done!\n");
}
wait(NULL);
wait(NULL);
printf("\nParent: All Children Complete");
}
return 0;
}

Step by step
Solved in 4 steps with 2 images

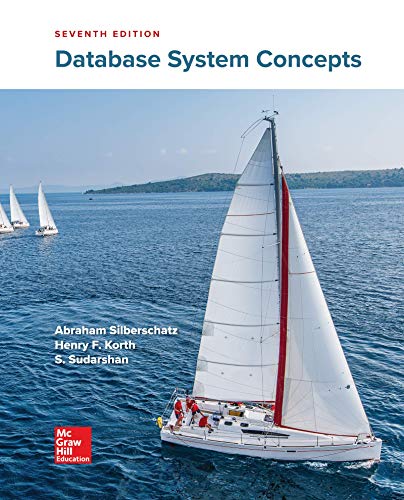
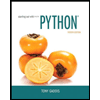
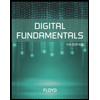
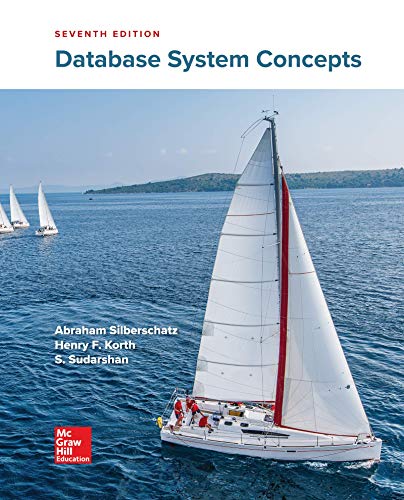
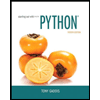
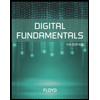
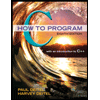
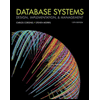
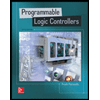