I'm trying to construct 2 methods to sort an array One method will sort an array in ascending order the other method will sort the array in the sending order. To try to implement concurrency of threading. Writen in java.
I'm trying to construct 2 methods to sort an array One method will sort an array in ascending order the other method will sort the array in the sending order. To try to implement concurrency of threading. Writen in java.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I'm trying to construct 2 methods to sort an array One method will sort an array in ascending order the other method will sort the array in the sending order. To try to implement concurrency of threading. Writen in java.
![This Java code snippet is designed to demonstrate process synchronization using mutex locks or semaphores. The code features a class named `Question3_SortingArrays` that operates on a shared resource, which is a one-dimensional array referred to as a buffer. Two threads, Thread 1 and Thread 2, perform sorting operations on this buffer. Thread 1 sorts the buffer in ascending order, while Thread 2 sorts it in descending order.
### Code Explanation:
1. **Package Declaration**
- `package Threads_Synchronization;`
- This line declares the package to which this class belongs.
2. **Imports**
- `import java.util.Random;`
- `import java.util.concurrent.locks.Lock;`
- `import java.util.concurrent.locks.ReentrantLock;`
- These import statements import the necessary Java libraries for random number generation and lock mechanisms.
3. **Class Declaration**
- `public class Question3_SortingArrays {`
- The class `Question3_SortingArrays` encapsulates the logic for this synchronization task.
4. **Comments**
- Detailed comments describe the goal of using mutex locks or semaphores for synchronization.
- The comments explain the shared use of a buffer by two threads, each tasked with sorting in different orders.
5. **Shared Resources**
- `public static int BufferSize = 10;`
- Declares a static integer `BufferSize`, set to 10, indicating the size of the shared buffer.
- `public static int buffer[] = new int[BufferSize];`
- Declares and initializes an integer array `buffer` with the size defined by `BufferSize`.
6. **Additional Comment**
- Instructions to add further resources for `Ascending()` and `Descending()` functions as necessary for implementing the sorting logic.
This setup illustrates implementing synchronization mechanisms in concurrent programming, ensuring that multiple threads can safely and efficiently share resources.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F84b9759d-3bd7-481b-9da5-01ee09be0953%2F8ac34944-74a1-4c51-8c87-20bef22bd168%2Fgrapp3j_processed.jpeg&w=3840&q=75)
Transcribed Image Text:This Java code snippet is designed to demonstrate process synchronization using mutex locks or semaphores. The code features a class named `Question3_SortingArrays` that operates on a shared resource, which is a one-dimensional array referred to as a buffer. Two threads, Thread 1 and Thread 2, perform sorting operations on this buffer. Thread 1 sorts the buffer in ascending order, while Thread 2 sorts it in descending order.
### Code Explanation:
1. **Package Declaration**
- `package Threads_Synchronization;`
- This line declares the package to which this class belongs.
2. **Imports**
- `import java.util.Random;`
- `import java.util.concurrent.locks.Lock;`
- `import java.util.concurrent.locks.ReentrantLock;`
- These import statements import the necessary Java libraries for random number generation and lock mechanisms.
3. **Class Declaration**
- `public class Question3_SortingArrays {`
- The class `Question3_SortingArrays` encapsulates the logic for this synchronization task.
4. **Comments**
- Detailed comments describe the goal of using mutex locks or semaphores for synchronization.
- The comments explain the shared use of a buffer by two threads, each tasked with sorting in different orders.
5. **Shared Resources**
- `public static int BufferSize = 10;`
- Declares a static integer `BufferSize`, set to 10, indicating the size of the shared buffer.
- `public static int buffer[] = new int[BufferSize];`
- Declares and initializes an integer array `buffer` with the size defined by `BufferSize`.
6. **Additional Comment**
- Instructions to add further resources for `Ascending()` and `Descending()` functions as necessary for implementing the sorting logic.
This setup illustrates implementing synchronization mechanisms in concurrent programming, ensuring that multiple threads can safely and efficiently share resources.
![Below is the transcribed text from the given image, designed to appear on an educational website:
---
### Function: `displayStatus()`
This function displays the content of the shared buffer and indicates which thread made the call. **Do not change this function.**
```java
public static void displayStatus() {
if (Thread.currentThread().getName().equals("ascending"))
System.out.println("Ascending successfully sorted the array");
else
System.out.println("Descending successfully sorted the array");
System.out.print(" the " + Thread.currentThread().getName() + " is displaying the content of the buffer: ");
for (int i = 0; i < BufferSize; i++) {
System.out.print(buffer[i] + " ");
}
System.out.println();
}
```
### Function: `Ascending()`
This function sorts the shared buffer in ascending order.
```java
public static void Ascending() {
try {
System.out.println("The Ascending is trying to sort the shared buffer");
// Sort the buffer in ascending order
// Call displayStatus after you sort and before releasing the lock
// Implement the Ascending functionality in the area below
}
```
---](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F84b9759d-3bd7-481b-9da5-01ee09be0953%2F8ac34944-74a1-4c51-8c87-20bef22bd168%2F0i1hf37_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Below is the transcribed text from the given image, designed to appear on an educational website:
---
### Function: `displayStatus()`
This function displays the content of the shared buffer and indicates which thread made the call. **Do not change this function.**
```java
public static void displayStatus() {
if (Thread.currentThread().getName().equals("ascending"))
System.out.println("Ascending successfully sorted the array");
else
System.out.println("Descending successfully sorted the array");
System.out.print(" the " + Thread.currentThread().getName() + " is displaying the content of the buffer: ");
for (int i = 0; i < BufferSize; i++) {
System.out.print(buffer[i] + " ");
}
System.out.println();
}
```
### Function: `Ascending()`
This function sorts the shared buffer in ascending order.
```java
public static void Ascending() {
try {
System.out.println("The Ascending is trying to sort the shared buffer");
// Sort the buffer in ascending order
// Call displayStatus after you sort and before releasing the lock
// Implement the Ascending functionality in the area below
}
```
---
Expert Solution

Step 1
In this question we will write java code for ascending and descending order.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
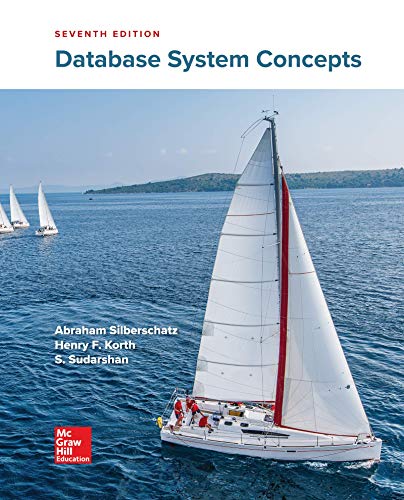
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
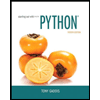
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
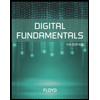
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
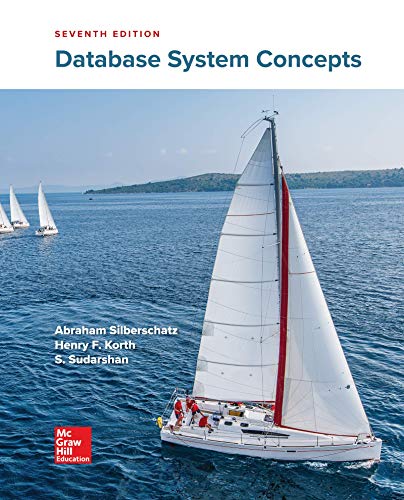
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
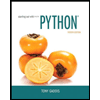
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
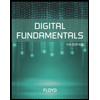
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
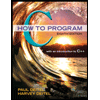
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
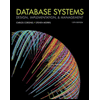
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
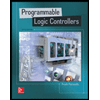
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education