2. Tree-reduction: Each thread will receive an segment of array of numbers, it will fork/spawn two new slaves each perform summing on different half of the received array segment, and the parent aggregate and return the total from its two slaves to its parent thread. Start with 7 nodes thread tree, when you are comfortable, you can extend it to a full thread tree . Test your code on different size of data What to submit: 1. Your python code 2. a screen (or video) capture of your code execution
/**
Iluustration of RecursiveTask
*/
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ForkJoinPool;
import java.util.concurrent.RecursiveTask;
public class SumWithPool{
public static void main(String[] args) throws InterruptedException,
ExecutionException {
//get the number of avaialbe CPUs
int nThreads = Runtime.getRuntime().availableProcessors();
System.out.println("Available CPUs: " + nThreads);
//create an array of data
int n = 10; //initital data size
if(args.length > 0) // use the user given data size
n = Integer.parseInt(args[0]);
int[] numbers = new int[n];
for(int i = 0; i < numbers.length; i++) {
numbers[i] = i;
}
ForkJoinPool forkJoinPool = new ForkJoinPool(nThreads);
Sum s = new Sum(numbers,0,numbers.length);
long startTime = System.currentTimeMillis(); //start timer
Long result = forkJoinPool.invoke(s);
long endTime = System.currentTimeMillis();
long timeElapsed = endTime - startTime;
System.out.println("Concurrent executing time: " + timeElapsed + "ms");
System.out.println("The conccurent sum: " + result);
//redo the task sequentially
startTime = System.currentTimeMillis(); //start timer
result = sum(numbers);
endTime = System.currentTimeMillis();
timeElapsed = endTime - startTime;
System.out.println("\nSequential executing time: " + timeElapsed + "ms");
System.out.println("The sequential sum: " + result);
}
/** sequential sum */
private static long sum(int[] arr){
long sum = 0;
for(int i = 0; i < arr.length; i++)
sum += arr[i];
return sum;
}
}
/** create a class that handle a task */
class Sum extends RecursiveTask<Long> {
private int low;
private int high;
private int[] array; //only reference to the given data
/** map the attribute to the given data */
public Sum(int[] array, int low, int high) {
this.array = array;
this.low = low;
this.high = high;
}
/** overriding tehe comput method */
protected Long compute() {
if(high - low <= 10) { //do the sum if the block of data is managable
long sum = 0;
for(int i = low; i < high; ++i)
sum += array[i];
return sum;
} else {
int mid = low + (high - low) / 2;
Sum left = new Sum(array, low, mid);
Sum right = new Sum(array, mid, high);
left.fork();
long rightResult = right.compute();
long leftResult = left.join();
return leftResult + rightResult;
}
}
}


Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 6 images

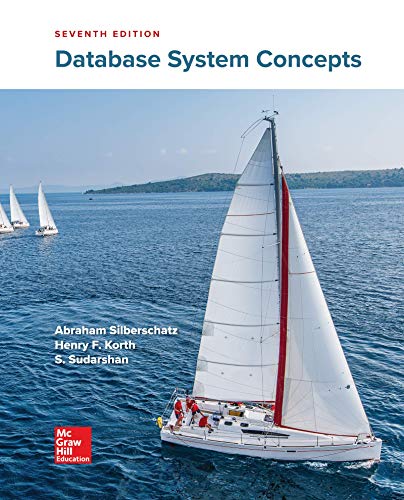
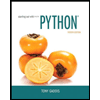
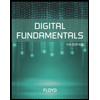
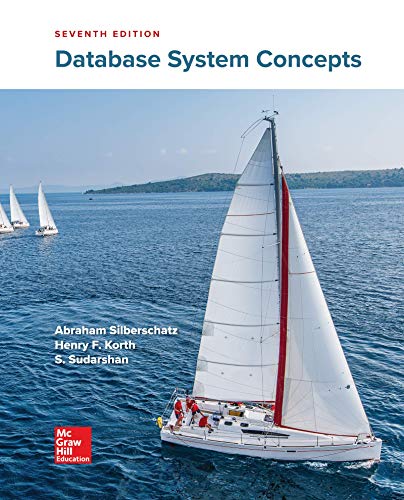
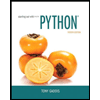
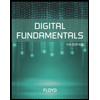
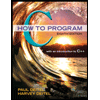
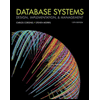
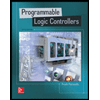