I'm getting an error message where the FindText() is returning 0 instead of the proper amount of parameters. Here is the code #include #include using namespace std; char print_menu(string); int GetNumOfNonWSCharacters(string); int GetNumOfWords(string); void ReplaceExclamation(string&); void ShortenSpace(string&); int FindText(string, string); int main() { char opt; string tx, ph; cout << "Enter a sample text:\n\n"; getline(cin, tx); cout << "You entered: " << tx; do { opt = print_menu(tx); } while (opt == 'Q' || 'q'); // system("pause"); return 0; } char print_menu(string tx) { char ch1; string ph; cout << "\n\nMENU\n"; cout << "c - Number of non-whitespace characters\nw - Number of words\nf - Find text\nr - Replace all !'s\ns - Shorten spaces\nq - Quit"; cout << "\n\nChoose an option:\n"; cin >> ch1; switch (ch1) { case 'q': case 'Q': exit(0); case 'c': case 'C': cout << "\nNumber of non-whitespace characters: " << GetNumOfNonWSCharacters(tx); break; case 'w': case 'W': cout << "\n\n Number of words: " << GetNumOfWords(tx); break; case 'f': case 'F': cin.ignore(); cout << "\nEnter a word or phrase to be found:"; //getvalue getline(cin, ph); cout << "\n\"" << ph << "\" instances: " << FindText(tx, ph); break; case 'r': case 'R': ReplaceExclamation(tx); cout << "\n\nEdited text: " << tx; break; case 's': case 'S': ShortenSpace(tx); cout << "\n\nEdited text: " << tx; break; default: cout << "\n\n Invalid \n\n"; break; } return ch1; } int GetNumOfNonWSCharacters(const string tx) { int cnt = 0, i; int leng = tx.size(); for (i = 0; i
I'm getting an error message where the FindText() is returning 0 instead of the proper amount of parameters.
Here is the code
#include <iostream>
#include <string>
using namespace std;
char print_menu(string);
int GetNumOfNonWSCharacters(string);
int GetNumOfWords(string);
void ReplaceExclamation(string&);
void ShortenSpace(string&);
int FindText(string, string);
int main()
{
char opt;
string tx, ph;
cout << "Enter a sample text:\n\n";
getline(cin, tx);
cout << "You entered: " << tx;
do
{
opt = print_menu(tx);
} while (opt == 'Q' || 'q');
// system("pause");
return 0;
}
char print_menu(string tx)
{
char ch1;
string ph;
cout << "\n\nMENU\n";
cout << "c - Number of non-whitespace characters\nw - Number of words\nf - Find text\nr - Replace all !'s\ns - Shorten spaces\nq - Quit";
cout << "\n\nChoose an option:\n";
cin >> ch1;
switch (ch1)
{
case 'q':
case 'Q':
exit(0);
case 'c':
case 'C':
cout << "\nNumber of non-whitespace characters: " << GetNumOfNonWSCharacters(tx);
break;
case 'w':
case 'W':
cout << "\n\n Number of words: " << GetNumOfWords(tx);
break;
case 'f':
case 'F':
cin.ignore();
cout << "\nEnter a word or phrase to be found:";
//getvalue
getline(cin, ph);
cout << "\n\"" << ph << "\" instances: " << FindText(tx, ph);
break;
case 'r':
case 'R': ReplaceExclamation(tx); cout << "\n\nEdited text: " << tx;
break;
case 's':
case 'S': ShortenSpace(tx); cout << "\n\nEdited text: " << tx;
break;
default:
cout << "\n\n Invalid \n\n";
break;
}
return ch1;
}
int GetNumOfNonWSCharacters(const string tx)
{
int cnt = 0, i;
int leng = tx.size();
for (i = 0; i<leng; i++)
{
if (!isspace(tx[i]))
cnt++;
}
return cnt;
}
int GetNumOfWords(const string tx)
{
int words11 = 0, i;
int leng = tx.size();
for (i = 0; i<leng;)
{
if (isspace(tx[i]))
{
while (isspace(tx[i]))
i++;
words11++;
}
else
{
i++;
}
}
words11 = words11 + 1;
return words11;
}
void ReplaceExclamation(string& tx)
{
string newtx = tx;
int i, leng = tx.size();
for (i = 0; i<leng; i++)
{
if (tx[i] == '!')
newtx[i] = '.';
}
tx = newtx;
}
void ShortenSpace(string& tx)
{
char *newtx;
int i1, leng = tx.size(), k = 0;
newtx = new char[leng + 1];
for (i1 = 0; i1<leng; k++)
{
newtx[k] = tx[i1];
if (isspace(tx[i1]))
{
while (isspace(tx[i1]))
i1++;
}
else
{
i1++;
}
}
newtx[k] = '\0';
tx = newtx;
}
int FindText(string tx, string ph)
{
int cnt = 0;
//size value
if (ph.size() == 0)
return 0;
//find value
for (size_t offset = tx.find(ph); offset != string::npos; offset = tx.find(ph, offset + ph.size()))
{
++cnt;
}
return cnt;
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

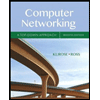
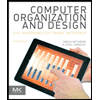
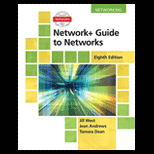
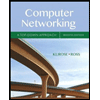
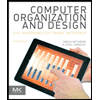
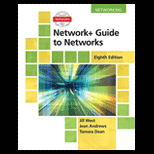
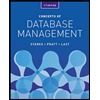
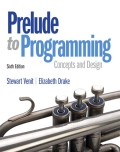
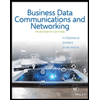