{ if(used capacity) { resize((1.5*capacity) + 1); } if(current index >= used) current_index = 0; { }
{ if(used capacity) { resize((1.5*capacity) + 1); } if(current index >= used) current_index = 0; { }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Topic Video
Question
![The image shows a list of error messages from a code execution or compilation process. Each error message is presented in a tabular format with several columns. Here's the transcription of the table contents:
1. **Line 112, Column 9**
Path: `C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...`
Error: `[Error] 'current_index' was not declared in this scope`
2. **Line 112, Column 26**
Path: `C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...`
Error: `[Error] 'used' was not declared in this scope`
3. **Line 117, Column 18**
Path: `C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...`
Error: `[Error] 'used' was not declared in this scope`
4. **Line 117, Column 28**
Path: `C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...`
Error: `[Error] 'current_index' was not declared in this scope`
5. **Line 119, Column 8**
Path: `C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...`
Error: `[Error] 'data' was not declared in this scope`
These errors indicate that certain variables (`current_index`, `used`, `data`) are being used in the code without having been declared in the visible parts of the code's scope. This type of error typically requires checking the variable declarations within the source code to ensure they are correctly defined before use.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Faad9e4ed-ed50-4fe3-96ca-72ed3d364f6f%2F3db6ef49-3da0-4c95-b946-28b98e7b0a21%2Fj256y5e_processed.png&w=3840&q=75)
Transcribed Image Text:The image shows a list of error messages from a code execution or compilation process. Each error message is presented in a tabular format with several columns. Here's the transcription of the table contents:
1. **Line 112, Column 9**
Path: `C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...`
Error: `[Error] 'current_index' was not declared in this scope`
2. **Line 112, Column 26**
Path: `C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...`
Error: `[Error] 'used' was not declared in this scope`
3. **Line 117, Column 18**
Path: `C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...`
Error: `[Error] 'used' was not declared in this scope`
4. **Line 117, Column 28**
Path: `C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...`
Error: `[Error] 'current_index' was not declared in this scope`
5. **Line 119, Column 8**
Path: `C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...`
Error: `[Error] 'data' was not declared in this scope`
These errors indicate that certain variables (`current_index`, `used`, `data`) are being used in the code without having been declared in the visible parts of the code's scope. This type of error typically requires checking the variable declarations within the source code to ensure they are correctly defined before use.
![The image shows a snippet of C++ code for a method named `insert` in a `sequence` class. This method takes an entry of type `value_type` as a parameter. Below is the transcription and explanation of each part of the code:
```cpp
void sequence::insert(const value_type& entry)
{
if (used >= capacity)
{
resize((1.5 * capacity) + 1);
}
if (current_index >= used)
{
current_index = 0;
}
for (int i = used; i > current_index; i--)
{
data[i] = data[i-1];
}
```
### Explanation:
1. **Method Declaration (Lines 106-107):**
- `void sequence::insert(const value_type& entry)`:
- This line declares a method named `insert`, which belongs to the `sequence` class. It doesn't return any value (`void`) and takes a single parameter, `entry`, which is a constant reference to a `value_type`.
2. **Conditional Statement for Capacity (Lines 108-111):**
- `if (used >= capacity) { resize((1.5 * capacity) + 1); }`
- Checks if the current number of elements (`used`) is greater than or equal to the array's capacity.
- If true, it calls the `resize` function to increase the capacity by 1.5 times the current capacity, plus one additional space (to accommodate the new insertion).
3. **Current Index Adjustment (Lines 113-116):**
- `if (current_index >= used) { current_index = 0; }`
- Ensures that if `current_index` is beyond the last used element, it resets to `0`. This likely prepares for an insertion at the beginning if no valid index is otherwise provided.
4. **Element Shifting Loop (Lines 118-120):**
- `for (int i = used; i > current_index; i--) { data[i] = data[i-1]; }`
- Initializes a loop with `i` starting at `used` and decrements down to one more than `current_index`.
- Shifts elements one position to the right to make space at `current_index` for the new entry. This is a common technique in dynamic array insertions.
This method efficiently manages dynamic array](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Faad9e4ed-ed50-4fe3-96ca-72ed3d364f6f%2F3db6ef49-3da0-4c95-b946-28b98e7b0a21%2Fgnaiqtf_processed.png&w=3840&q=75)
Transcribed Image Text:The image shows a snippet of C++ code for a method named `insert` in a `sequence` class. This method takes an entry of type `value_type` as a parameter. Below is the transcription and explanation of each part of the code:
```cpp
void sequence::insert(const value_type& entry)
{
if (used >= capacity)
{
resize((1.5 * capacity) + 1);
}
if (current_index >= used)
{
current_index = 0;
}
for (int i = used; i > current_index; i--)
{
data[i] = data[i-1];
}
```
### Explanation:
1. **Method Declaration (Lines 106-107):**
- `void sequence::insert(const value_type& entry)`:
- This line declares a method named `insert`, which belongs to the `sequence` class. It doesn't return any value (`void`) and takes a single parameter, `entry`, which is a constant reference to a `value_type`.
2. **Conditional Statement for Capacity (Lines 108-111):**
- `if (used >= capacity) { resize((1.5 * capacity) + 1); }`
- Checks if the current number of elements (`used`) is greater than or equal to the array's capacity.
- If true, it calls the `resize` function to increase the capacity by 1.5 times the current capacity, plus one additional space (to accommodate the new insertion).
3. **Current Index Adjustment (Lines 113-116):**
- `if (current_index >= used) { current_index = 0; }`
- Ensures that if `current_index` is beyond the last used element, it resets to `0`. This likely prepares for an insertion at the beginning if no valid index is otherwise provided.
4. **Element Shifting Loop (Lines 118-120):**
- `for (int i = used; i > current_index; i--) { data[i] = data[i-1]; }`
- Initializes a loop with `i` starting at `used` and decrements down to one more than `current_index`.
- Shifts elements one position to the right to make space at `current_index` for the new entry. This is a common technique in dynamic array insertions.
This method efficiently manages dynamic array
Expert Solution

Step 1
we must declare sequence variables them before using them .
Below program is a similar example:
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
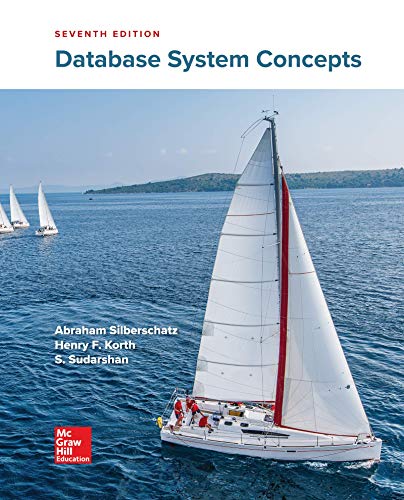
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
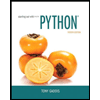
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
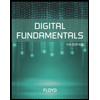
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
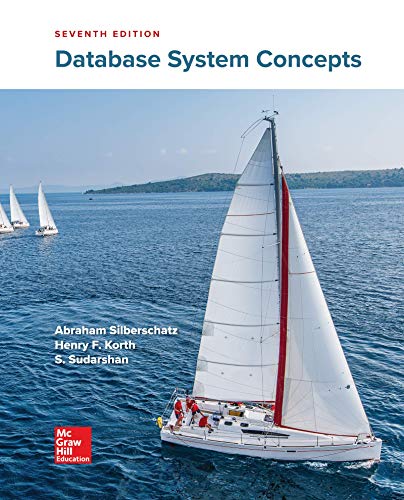
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
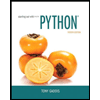
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
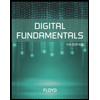
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
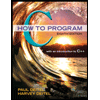
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
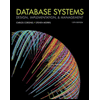
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
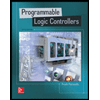
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education