If N represents the number of elements in the queue, then the enqueue method of the ArrayBoundedQueue class is O(N). true or false
If N represents the number of elements in the queue, then the enqueue method of the ArrayBoundedQueue class is O(N). true or false
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
If N represents the number of elements in the queue, then the enqueue method of the ArrayBoundedQueue class is O(N).
true or false
![```java
public class ArrayBoundedQueue<T> implements QueueInterface<T>
{
protected final int DEFCAP = 100; // default capacity
protected T[] elements; // array that holds queue elements
protected int numElements = 0; // number of elements in this queue
protected int front = 0; // index of front of queue
protected int rear; // index of rear of queue
public ArrayBoundedQueue()
{
elements = (T[]) new Object[DEFCAP];
rear = DEFCAP - 1;
}
public ArrayBoundedQueue(int maxSize)
{
elements = (T[]) new Object[maxSize];
rear = maxSize - 1;
}
public void enqueue(T element)
// Throws QueueOverflowException if this queue is full;
// otherwise, adds element to the rear of this queue.
{
if (isFull())
throw new QueueOverflowException("Enqueue attempted on a full queue.");
else
{
rear = (rear + 1) % elements.length;
elements[rear] = element;
numElements = numElements + 1;
}
}
public T dequeue()
// Throws QueueUnderflowException if this queue is empty;
// otherwise, removes front element from this queue and returns it.
{
if (isEmpty())
throw new QueueUnderflowException("Dequeue attempted on empty queue.");
else
{
T toReturn = elements[front];
elements[front] = null;
front = (front + 1) % elements.length;
numElements = numElements - 1;
return toReturn;
}
}
public boolean isEmpty()
// Returns true if this queue is empty; otherwise, returns false.
{
return (numElements == 0);
}
public boolean isFull()
// Returns true if this queue is full; otherwise, returns false.
{
return (numElements == elements.length);
}
public int size()
// Returns the number of elements in this queue.
{
return numElements;
}
}
```
This code represents an implementation of a generic `ArrayBoundedQueue` class, which is a type of data structure known as a queue. The queue elements are stored in an array and are designed](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1b2c1e36-9974-49eb-8a0c-fe4d3c635f06%2F1154ec18-47ec-4afb-9bb9-ffe2c9a5ba88%2Fb5e9s4e_processed.png&w=3840&q=75)
Transcribed Image Text:```java
public class ArrayBoundedQueue<T> implements QueueInterface<T>
{
protected final int DEFCAP = 100; // default capacity
protected T[] elements; // array that holds queue elements
protected int numElements = 0; // number of elements in this queue
protected int front = 0; // index of front of queue
protected int rear; // index of rear of queue
public ArrayBoundedQueue()
{
elements = (T[]) new Object[DEFCAP];
rear = DEFCAP - 1;
}
public ArrayBoundedQueue(int maxSize)
{
elements = (T[]) new Object[maxSize];
rear = maxSize - 1;
}
public void enqueue(T element)
// Throws QueueOverflowException if this queue is full;
// otherwise, adds element to the rear of this queue.
{
if (isFull())
throw new QueueOverflowException("Enqueue attempted on a full queue.");
else
{
rear = (rear + 1) % elements.length;
elements[rear] = element;
numElements = numElements + 1;
}
}
public T dequeue()
// Throws QueueUnderflowException if this queue is empty;
// otherwise, removes front element from this queue and returns it.
{
if (isEmpty())
throw new QueueUnderflowException("Dequeue attempted on empty queue.");
else
{
T toReturn = elements[front];
elements[front] = null;
front = (front + 1) % elements.length;
numElements = numElements - 1;
return toReturn;
}
}
public boolean isEmpty()
// Returns true if this queue is empty; otherwise, returns false.
{
return (numElements == 0);
}
public boolean isFull()
// Returns true if this queue is full; otherwise, returns false.
{
return (numElements == elements.length);
}
public int size()
// Returns the number of elements in this queue.
{
return numElements;
}
}
```
This code represents an implementation of a generic `ArrayBoundedQueue` class, which is a type of data structure known as a queue. The queue elements are stored in an array and are designed
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
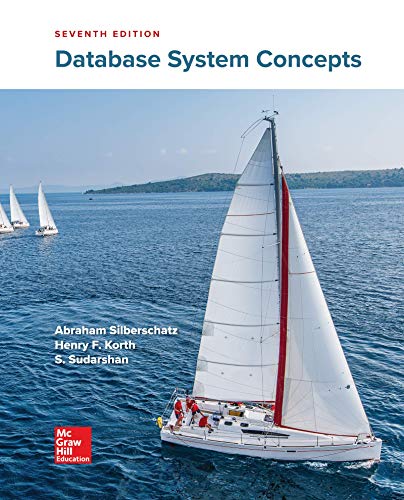
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
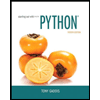
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
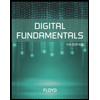
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
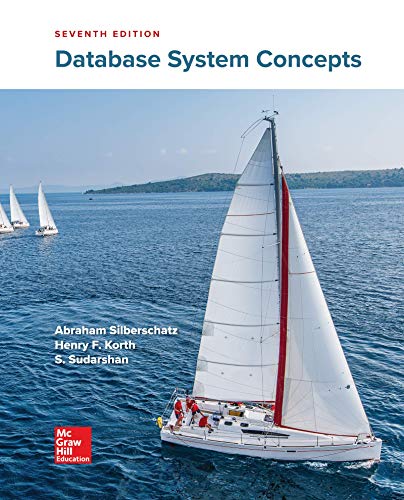
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
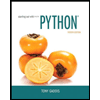
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
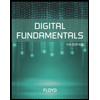
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
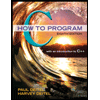
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
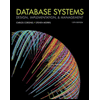
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
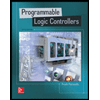
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education