I submited this quesiton but it got refuned because the expert said my code needed to be more clear I don't understand what he ment but I copyed my code and pasted it here. I need help with this python code because my teacher asked me to change some stuff around. These are her exact request. The loop to control the menu should be in main() while not 5 call menu You should have the user enter the ISBN with the hyphens (-) and use your string function, method, etc to process the option. Hope this helps, def main(): menu() def menu(): print('1. Verify the check digit of an ISBN-10') print("2. verify the check digit of an ISBN-13") print("3. Convert an ISBN-10 to an ISBN-13") print('4. Convert an ISBN-13 to an ISBN-10') print('5. Exit') command = input("Enter the Command (1-5): ") if command == '5': return elif command == '1' or command == '3': #get 10 digit input ISBN = input("Please enter the 10 digit number: ") while len(ISBN) != 10: print("Please make sure you have entered a number which is exactly 10 characters long.") ISBN = input("Please enter the 10 digit number: ") print(format(ISBN)) if command == '1': print(check_10_digit(ISBN)) else: print(convert_10_to_13(ISBN)) elif command == '2' or command == '4': #get 13 digit input ISBN = input("Please enter the 13 digit number: ") while len(format(ISBN)) != 13: print("Please make sure you have entered a number which is exactly 13 characters long") ISBN = input("Please enter the 13 digit number: ") if command == '2': print(check_13_digit(ISBN)) else: print(convert_13_to_10(ISBN)) #show the menu again menu() def check_10_digit(ISBN): #This portion of code will pull the entered ISBN from my Menu() statement and add all the numbers. #It will then find the remainder of those numbers, if th remainder is 0 it will say the ISBN is valid #If it comes back with the formula having a remainder, it will say NOT Valid. digit = 0 for i in range(len(ISBN)): n = ISBN[i] num = int(n) digit += (i+1) * num d10 = digit % 11 print("Your ISBN-10 is: ", ISBN, "with a remainder of", str(d10)) if d10 == 0: print("Your ISBN-10 is valid") else: print("Your ISBN-10 is NOT valid") def check_13_digit(ISBN): #Calcualte the ISBN Check Digit for 13 digit numbers n = str(ISBN) digit = 0 for i in range(len(n)): if i % 2 == 0: digit += int(n[i]) else: digit += int(n[i]) * 3 digit = digit % 10 if digit == 0: print("Your remainder is: ", digit, "your ISBN-13 is Valid") else: print("Your remainder is: ", digit, "your ISBN-13 is NOT Valid") def convert_10_to_13(ISBN): #Convert an ISBN-10 number to a ISBN-13 number ISBN_13 = '978' + ISBN[:-1] n = str(ISBN_13) digit = 0 for i in range(len(n)): if i % 2 == 0: digit += int(n[i]) else: digit += int(n[i]) * 3 digit = digit % 10 ISBN_New = str(ISBN_13) + str(digit) print("The ISBN-10 number ", ISBN, "is converted to the ISBN-13 number ", ISBN_New) def convert_13_to_10(ISBN): #Convert an ISBN-13 number to a ISBN-10 number if ISBN[:3] == '978': n = str(ISBN[3:-1]) else : raise "ISBN is not convertible." digit = 0 for i in range(len(n)): if i % 2 == 0: digit += int(n[i]) else: digit += int(n[i]) * 3 digit = 10 - (digit % 10) ISBN_New = str(n) + str(digit) print("The ISBN-13 number ", ISBN, "is converted to the ISBN-10 number ", ISBN_New) main()
I submited this quesiton but it got refuned because the expert said my code needed to be more clear I don't understand what he ment but I copyed my code and pasted it here. I need help with this python code because my teacher asked me to change some stuff around. These are her exact request.
def main():
menu()
def menu():
print('1. Verify the check digit of an ISBN-10')
print("2. verify the check digit of an ISBN-13")
print("3. Convert an ISBN-10 to an ISBN-13")
print('4. Convert an ISBN-13 to an ISBN-10')
print('5. Exit')
command = input("Enter the Command (1-5): ")
if command == '5':
return
elif command == '1' or command == '3':
#get 10 digit input
ISBN = input("Please enter the 10 digit number: ")
while len(ISBN) != 10:
print("Please make sure you have entered a number which is exactly 10 characters long.")
ISBN = input("Please enter the 10 digit number: ")
print(format(ISBN))
if command == '1':
print(check_10_digit(ISBN))
else:
print(convert_10_to_13(ISBN))
elif command == '2' or command == '4':
#get 13 digit input
ISBN = input("Please enter the 13 digit number: ")
while len(format(ISBN)) != 13:
print("Please make sure you have entered a number which is exactly 13 characters long")
ISBN = input("Please enter the 13 digit number: ")
if command == '2':
print(check_13_digit(ISBN))
else:
print(convert_13_to_10(ISBN))
#show the menu again
menu()
def check_10_digit(ISBN):
#This portion of code will pull the entered ISBN from my Menu() statement and add all the numbers.
#It will then find the remainder of those numbers, if th remainder is 0 it will say the ISBN is valid
#If it comes back with the formula having a remainder, it will say NOT Valid.
digit = 0
for i in range(len(ISBN)):
n = ISBN[i]
num = int(n)
digit += (i+1) * num
d10 = digit % 11
print("Your ISBN-10 is: ", ISBN, "with a remainder of", str(d10))
if d10 == 0:
print("Your ISBN-10 is valid")
else:
print("Your ISBN-10 is NOT valid")
def check_13_digit(ISBN):
#Calcualte the ISBN Check Digit for 13 digit numbers
n = str(ISBN)
digit = 0
for i in range(len(n)):
if i % 2 == 0:
digit += int(n[i])
else:
digit += int(n[i]) * 3
digit = digit % 10
if digit == 0:
print("Your remainder is: ", digit, "your ISBN-13 is Valid")
else:
print("Your remainder is: ", digit, "your ISBN-13 is NOT Valid")
def convert_10_to_13(ISBN):
#Convert an ISBN-10 number to a ISBN-13 number
ISBN_13 = '978' + ISBN[:-1]
n = str(ISBN_13)
digit = 0
for i in range(len(n)):
if i % 2 == 0:
digit += int(n[i])
else:
digit += int(n[i]) * 3
digit = digit % 10
ISBN_New = str(ISBN_13) + str(digit)
print("The ISBN-10 number ", ISBN, "is converted to the ISBN-13 number ", ISBN_New)
def convert_13_to_10(ISBN):
#Convert an ISBN-13 number to a ISBN-10 number
if ISBN[:3] == '978':
n = str(ISBN[3:-1])
else :
raise "ISBN is not convertible."
digit = 0
for i in range(len(n)):
if i % 2 == 0:
digit += int(n[i])
else:
digit += int(n[i]) * 3
digit = 10 - (digit % 10)
ISBN_New = str(n) + str(digit)
print("The ISBN-13 number ", ISBN, "is converted to the ISBN-10 number ", ISBN_New)
main()
![à LaForce Final Project.py - D:\Python\LaForce Final Project.py (3.8.5)
File Edit Format Run Options Window Help
n = ISBN[i]
num = int (n)
digit += (i+1) * num
di0 = digit 11
print ("Your ISBN-10 is: ", ISBN, "with a remainder of", str (d10))
if d10 == 0:
print ("Your ISBN-10 is valid")
else:
print ("Your ISBN-10 is NOT valid")
def check 13 digit (ISBN) :
#Calcualte the ISBN Check Digit for 13 digit numbers
n = str (ISBN)
digit = 0
for i in range (len (n)) :
if i $ 2 =- 0:
digit += int (n[i])
else:
digit += int (n[i]) * 3
digit - digit * 10
if digit == 0:
print ("Your remainder is: ", digit, "your ISBN-13 is Valid")
else:
print ("Your remainder is: ", digit, "your ISBN-13 is NOT Valid")
def convert 10 to 13 (ISBN) :
#Convert an ISBN-10 number to a ISBN-13 number
ISBN 13 = '978' + ISBN[:-1]
n = str (ISBN_13)
digit = o
for i in range (len (n) ) :
if i 2 == 0:
digit += int (n[i])
else:
digit += int (n[i]) * 3
digit = digit % 10
ISBN New = str (ISBN 13) + str (digit)
print ("The ISBN-10 number ", ISBN, "is converted to the ISBN-13 number ",
def convert 13 to 10 (ISBN) :
#Convert an ISBN-13 number to a ISBN-10 number
if ISBN[:3] == '978':
n = str (ISBN[3:-1])
else :
raise "ISBN is not convertible."
digit = 0
for i in range (len (n) ) :
if i $ 2 == 0:
digit += int (n[i])
else:
digit += int (n[i])
* 3
digit = 10 - (digit $ 10)
ISBN New = str (n)
+ str (digit)
print ("The ISBN-13 number ", ISBN, "is converted to the ISBN-10 number ", ISBN New)
main ()
Ln:
Col: 0
5:04 PM
O Type here to search
12/5/2020](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc43e03e1-b353-4d50-a726-bab1a8232fef%2F51dd73a5-f2de-4332-8300-c74630e377d0%2Fpvny5o_processed.png&w=3840&q=75)
![à LaForce Final Project.py - D:\Python\LaForce Final Project.py (3.8.5)
File Edit Format Run Options Window Help
def main () :
menu ()
def menu () :
print ('1. Verify the check digit of an ISBN-10')
print ("2. verify the check digit of an ISBN-13")
print ("3. Convert an ISBN-10 to an ISBN-13")
print ('4.
Convert an ISBN-13 to an ISBN-10')
print ('5. Exit')
command
input ("Enter the Command (1-5): ")
if command == '5':
return
elif command == '1' or command == '3':
#get 10 digit input
ISBN = input ("Please enter the 10 digit number: ")
while len (ISBN)
!= 10:
print ("Please make sure you have entered a number which is exactly 10 characters long.")
ISBN = input ("Please enter the 10 digit number: ")
print (format (ISBN) )
if command == '1':
print (check_10_digit (ISBN))
else:
print (convert 10 to l3 (ISBN))
elif command == '2'
or command =='4':
#get 13 digit input
ISBN = input ("Please enter the l3 digit number: ")
while len (format (ISBN)) != 13:
print ("Please make sure you have entered a number which is exactly 13 characters long")
ISBN = input ("Please enter the l3 digit number: ")
if command =- '2':
print (check 13 digit (ISBN))
else:
print (convert 13 to 10(ISBN))
#show the menu again
menu ()
def check 10 digit (ISBN) :
#This portion of code will pull the entered ISBN from my Menu () statement and add all the numbers.
#It will then find the remainder of those numbers, if th remainder is 0 it will say the ISBN is valid
#If it comes back with the formula having a remainder, it will say NOT Valid.
digit = 0
for i in range (len (ISBN)):
n = ISBN[i]
num = int (n)
digit += (i+1) * num
d10 = digit $ 11
print ("Your ISBN-10 is: ", ISBN, "with a remainder of", str (d10))
if d10 == 0:
print ("Your ISBN-10 is valid")
else:
print ("Your ISBN-10 is NOT valid")
def check 13 digit (ISBN) :
#Calcualte the ISBN Check Digit for 13 digit numbers
n = str (ISBN)
digit = 0
for i in range (len (n) ) :
Ln: 1
Col: 0
5:04 PM
O Type here to search
12/5/2020
近](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc43e03e1-b353-4d50-a726-bab1a8232fef%2F51dd73a5-f2de-4332-8300-c74630e377d0%2Fneu7xos_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

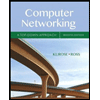
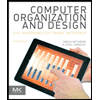
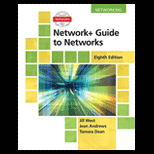
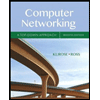
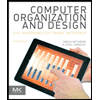
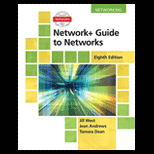
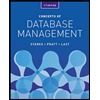
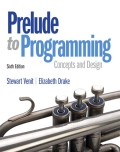
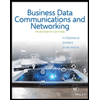