I need to create code (state machine) that when the user types on or off the LED on the board turns on or off. This is for a texas instruments CC3220s board. Now it is time to modify uartecho. Your code will need to accomplish the following: • Use only one byte of RAM for the serial buffer ( char input; ). • Use only one byte of RAM for the state ( unsigned char state; ). • Receive only one character at a time from the UART and it is not buffered. • Turn on an LED when a user types ON into the console. • Turn off the LED when a user types OFF into the console. #include #include /* Driver Header files */ #include #include /* Driver configuration */ #include "ti_drivers_config.h" /* * ======== mainThread ======== */ void *mainThread(void *arg0) { char input; const char echoPrompt[] = "Echoing characters:\r\n"; UART_Handle uart; UART_Params uartParams; /* Call driver init functions */ GPIO_init(); UART_init(); /* Configure the LED pin */ GPIO_setConfig(CONFIG_GPIO_LED_0, GPIO_CFG_OUT_STD | GPIO_CFG_OUT_LOW); /* Create a UART with data processing off. */ UART_Params_init(&uartParams); uartParams.writeDataMode = UART_DATA_BINARY; uartParams.readDataMode = UART_DATA_BINARY; uartParams.readReturnMode = UART_RETURN_FULL; uartParams.baudRate = 115200; uart = UART_open(CONFIG_UART_0, &uartParams); if (uart == NULL) { /* UART_open() failed */ while (1); } /* Turn on user LED to indicate successful initialization */ GPIO_write(CONFIG_GPIO_LED_0, CONFIG_GPIO_LED_ON); UART_write(uart, echoPrompt, sizeof(echoPrompt)); /* Loop forever echoing */ while (1) { UART_read(uart, &input, 1); //Insert State Machine Here UART_write(uart, &input, 1); } }.
I need to create code (state machine) that when the user types on or off the LED on the board turns on or off. This is for a texas instruments CC3220s board.
Now it is time to modify uartecho. Your code will need to accomplish the following:
• Use only one byte of RAM for the serial buffer ( char input; ).
• Use only one byte of RAM for the state ( unsigned char state; ).
• Receive only one character at a time from the UART and it is not buffered.
• Turn on an LED when a user types ON into the console.
• Turn off the LED when a user types OFF into the console.
#include <stdint.h>
#include <stddef.h>
/* Driver Header files */
#include <ti/drivers/GPIO.h>
#include <ti/drivers/UART.h>
/* Driver configuration */
#include "ti_drivers_config.h"
/*
* ======== mainThread ========
*/
void *mainThread(void *arg0)
{
char input;
const char echoPrompt[] = "Echoing characters:\r\n";
UART_Handle uart;
UART_Params uartParams;
/* Call driver init functions */
GPIO_init();
UART_init();
/* Configure the LED pin */
GPIO_setConfig(CONFIG_GPIO_LED_0, GPIO_CFG_OUT_STD | GPIO_CFG_OUT_LOW);
/* Create a UART with data processing off. */
UART_Params_init(&uartParams);
uartParams.writeDataMode = UART_DATA_BINARY;
uartParams.readDataMode = UART_DATA_BINARY;
uartParams.readReturnMode = UART_RETURN_FULL;
uartParams.baudRate = 115200;
uart = UART_open(CONFIG_UART_0, &uartParams);
if (uart == NULL) {
/* UART_open() failed */
while (1);
}
/* Turn on user LED to indicate successful initialization */
GPIO_write(CONFIG_GPIO_LED_0, CONFIG_GPIO_LED_ON);
UART_write(uart, echoPrompt, sizeof(echoPrompt));
/* Loop forever echoing */
while (1) {
UART_read(uart, &input, 1);
//Insert State Machine Here
UART_write(uart, &input, 1);
}
}.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

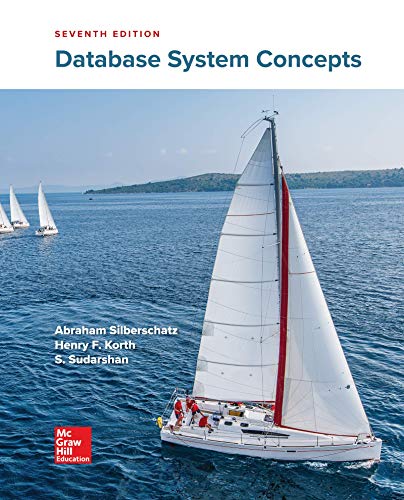
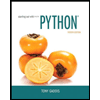
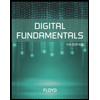
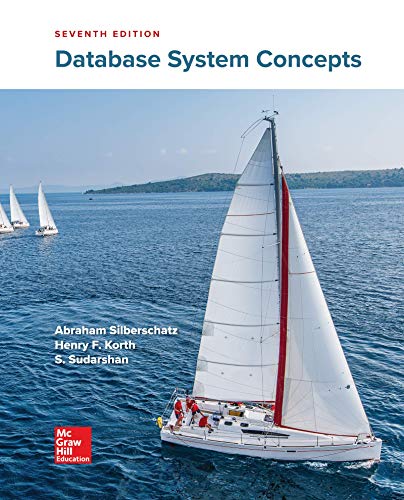
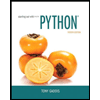
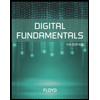
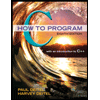
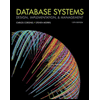
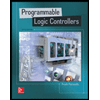