Hello, can you please help me fix this error in my code using the LC3 simulator using assembly. please show what changes you have made while still meeting the requirements here is the error I am getting while checking if the operator is -: Oh no! You've got an error in your assembly code: at line 128: immediate field is out of range: expected value to fit in 5 bits (i.e., to be between -16 and 15, inclusive), but found -45 ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;; .ORIG x3000 Main;====== Main Program ====== LEA R0, PROMPT1 ; Load the first number prompt and display to the user PUTS LD R0, NEWLINE ; OUT JSR GETNUM ; LD R0, NEWLINE ; OUT LEA R0, PROMPT2 ; Load the operator prompt and display to the user PUTS LD R0, NEWLINE ; Line break to prepare for user input OUT JSR GETOP ; Get operator and save it in the OP memory location LD R0, NEWLINE ; Line break to prepare for user input OUT LEA R0, PROMPT3 ; Load the second number prompt and display to the user PUTS LD R0, NEWLINE ; Line break to prepare for user input OUT JSR GETNUM ; Get second number and save it in the NUM2 memory location LD R0, NEWLINE ; Line break to prepare for user input OUT JSR CALC ; Calculate the result of NUM1 and NUM2 based on the operator in OP and save it in RESULT LD R0, NEWLINE ; Line break to prepare for user input OUT LEA R0, PROMPT4 ; Load the results prompt and display to the user PUTS LD R0, NEWLINE ; Line break to prepare for user input OUT JSR DISPLAY ; Display the stored result from RESULT BRnzp Main ; Branch back to the beginning of main to start looping END ;End program ;===== Sub-routines ===== GETNUM AND R0, R0, #0000 ; Clear R0 AND R1, R1, #0000 ; Clear R1 AND R2, R2, #0000 ; Clear R2 AND R3, R3, #0000 ; Clear R3 GETC ; Get user input and save it in R0 ST R0, NUM1 ; Store the number in the NUM1 memory location ; Convert ASCII character to decimal LD R2, NUM1 ; Load memory content stored in memory location NUM1 AND R2, R2, #11 ; Mask and find out the last digit of the number ADD R2, R2, #0015 ; Convert to ASCII character ADD R2,R2, R3 ; Add both ASCII characters AND R2, R2, #0011 ;Mask and extract the last digit of the number ADD R2, R2, #0015 ;Convert to ASCII character ADD R2, R2, R1 ;Add both ASCII characters ST R2, NUM1 ; Store the adjusted number in the NUM1 memory location RET GETOP AND R0, R0, #0000 ; Clear R0 AND R1, R1, #0000 ; Clear R1 GETC ; Get user input and save it in R0 ST R0, RESULT ; Store the operand in the OP memory location RET CALC LD R2, NUM1 ;Load content stored in NUM1 memory location LD R3, NUM2 ;Load content stored in NUM2 memory location LD R4, OP ; Load content stored in OP memory location ADD R2, R2, R3 ; Add both memory locations, and save result in R2 BRn RESET ; If number is less than 0, jump to RESET label BRp RESET ; If number is greater than 99, jump to RESET BRzp CONT ; If result is within the range of 0-99, jump to CONT RESET AND R0, R0, #0000 ; Clear R0 ST R0, RESULT ; Clear RESULT memory location CONT ; Check and convert ASCII operands to machine language ; Addition ADD R4, R4, #0011 ; Check if operator is + BRz PLUS ; If operator is +, Jump to PLUS ; Subtraction ADD R4, R4, #-45 ; Check if operator is - BRz MINUS ; If operator is -, Jump to MINUS ; Multiplication ADD R4, #002A ; Check if operator is * BRz MULTIPLY ; If operator is *, Jump to MULTIPLY PLUS ADD R2, R3 ; Add both numbers for addition ST R2, RESULT ; Store result in RESULT memory location RET MINUS NOT R3, R3 ; Negate the number ADD R2, R3 ; Perform subtraction ST R2, RESULT ; Store result in RESULT RET MULTIPLY ; Multiply both numbers and save result in R2 ; Subtract by 1 NOT R3, R3 ; Negate R3 ADD R2, R3 ; Perform subtraction ; Check if result is less than 0 BRz Zero ; If result is < 0, jump to ZERO BRp RESET ; If the result is > 99, jump to RESRICON Zero ADD R2, #0001 ; Add 1 to the result ST R2, RESULT ; Store result in RESULT RET DISPLAY LD R0, RESULT ; Load the result of the calculation from RESULT memory location ADD R0, #0030 ; Convert to ASCII character OUT ; Output the result to the screen RET ;===== Constants, Messages, and Prompts ===== PROMPT1 .STRINGZ "Enter first number (0 - 99): " PROMPT2 .STRINGZ "Enter an operation (+, -, *): " PROMPT3 .STRINGZ "Enter second number (0 - 99): " PROMPT4 .STRINGZ "Result: " NEWLINE .FILL x000A ;===== Memory Locations ===== NUM1 .BLKW #1 NUM2 .BLKW #1 OP .BLKW #1 RESULT .BLKW #1 .END
Hello, can you please help me fix this error in my code using the LC3 simulator using assembly. please show what changes you have made while still meeting the requirements
here is the error I am getting while checking if the operator is -:
Oh no! You've got an error in your assembly code:
- at line 128: immediate field is out of range: expected value to fit in 5 bits (i.e., to be between -16 and 15, inclusive), but found -45
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
.ORIG x3000
Main;====== Main
LEA R0, PROMPT1 ; Load the first number prompt and display to the user
PUTS
LD R0, NEWLINE ;
OUT
JSR GETNUM ;
LD R0, NEWLINE ;
OUT
LEA R0, PROMPT2 ; Load the operator prompt and display to the user
PUTS
LD R0, NEWLINE ; Line break to prepare for user input
OUT
JSR GETOP ; Get operator and save it in the OP memory location
LD R0, NEWLINE ; Line break to prepare for user input
OUT
LEA R0, PROMPT3 ; Load the second number prompt and display to the user
PUTS
LD R0, NEWLINE ; Line break to prepare for user input
OUT
JSR GETNUM ; Get second number and save it in the NUM2 memory location
LD R0, NEWLINE ; Line break to prepare for user input
OUT
JSR CALC ; Calculate the result of NUM1 and NUM2 based on the operator in OP and save it in RESULT
LD R0, NEWLINE ; Line break to prepare for user input
OUT
LEA R0, PROMPT4 ; Load the results prompt and display to the user
PUTS
LD R0, NEWLINE ; Line break to prepare for user input
OUT
JSR DISPLAY ; Display the stored result from RESULT
BRnzp Main ; Branch back to the beginning of main to start looping
END ;End program
;===== Sub-routines =====
GETNUM
AND R0, R0, #0000 ; Clear R0
AND R1, R1, #0000 ; Clear R1
AND R2, R2, #0000 ; Clear R2
AND R3, R3, #0000 ; Clear R3
GETC ; Get user input and save it in R0
ST R0, NUM1 ; Store the number in the NUM1 memory location
; Convert ASCII character to decimal
LD R2, NUM1 ; Load memory content stored in memory location NUM1
AND R2, R2, #11 ; Mask and find out the last digit of the number
ADD R2, R2, #0015 ; Convert to ASCII character
ADD R2,R2, R3 ; Add both ASCII characters
AND R2, R2, #0011 ;Mask and extract the last digit of the number
ADD R2, R2, #0015 ;Convert to ASCII character
ADD R2, R2, R1 ;Add both ASCII characters
ST R2, NUM1 ; Store the adjusted number in the NUM1 memory location
RET
GETOP
AND R0, R0, #0000 ; Clear R0
AND R1, R1, #0000 ; Clear R1
GETC ; Get user input and save it in R0
ST R0, RESULT ; Store the operand in the OP memory location
RET
CALC
LD R2, NUM1 ;Load content stored in NUM1 memory location
LD R3, NUM2 ;Load content stored in NUM2 memory location
LD R4, OP ; Load content stored in OP memory location
ADD R2, R2, R3 ; Add both memory locations, and save result in R2
BRn RESET ; If number is less than 0, jump to RESET label
BRp RESET ; If number is greater than 99, jump to RESET
BRzp CONT ; If result is within the range of 0-99, jump to CONT
RESET
AND R0, R0, #0000 ; Clear R0
ST R0, RESULT ; Clear RESULT memory location
CONT
; Check and convert ASCII operands to machine language
; Addition
ADD R4, R4, #0011 ; Check if operator is +
BRz PLUS ; If operator is +, Jump to PLUS
; Subtraction
ADD R4, R4, #-45 ; Check if operator is -
BRz MINUS ; If operator is -, Jump to MINUS
; Multiplication
ADD R4, #002A ; Check if operator is *
BRz MULTIPLY ; If operator is *, Jump to MULTIPLY
PLUS
ADD R2, R3 ; Add both numbers for addition
ST R2, RESULT ; Store result in RESULT memory location
RET
MINUS
NOT R3, R3 ; Negate the number
ADD R2, R3 ; Perform subtraction
ST R2, RESULT ; Store result in RESULT
RET
MULTIPLY
; Multiply both numbers and save result in R2
; Subtract by 1
NOT R3, R3 ; Negate R3
ADD R2, R3 ; Perform subtraction
; Check if result is less than 0
BRz Zero ; If result is < 0, jump to ZERO
BRp RESET ; If the result is > 99, jump to RESRICON
Zero
ADD R2, #0001 ; Add 1 to the result
ST R2, RESULT ; Store result in RESULT
RET
DISPLAY
LD R0, RESULT ; Load the result of the calculation from RESULT memory location
ADD R0, #0030 ; Convert to ASCII character
OUT ; Output the result to the screen
RET
;===== Constants, Messages, and Prompts =====
PROMPT1 .STRINGZ "Enter first number (0 - 99): "
PROMPT2 .STRINGZ "Enter an operation (+, -, *): "
PROMPT3 .STRINGZ "Enter second number (0 - 99): "
PROMPT4 .STRINGZ "Result: "
NEWLINE .FILL x000A
;===== Memory Locations =====
NUM1 .BLKW #1
NUM2 .BLKW #1
OP .BLKW #1
RESULT .BLKW #1
.END

Step by step
Solved in 3 steps

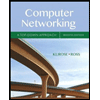
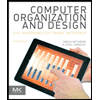
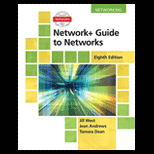
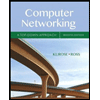
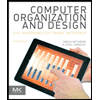
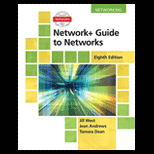
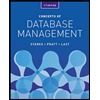
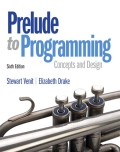
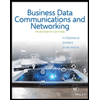