I need image part in this program // C++ program to create and implement FCFS Queue of customers #include #include using namespace std; class Customer { private: char name[15]; int arrivalTime; int serviceTime; int finishTime; public: // function to set name, arrivalTime and serviceTime to specified values void setCustomer(char *name, int arrivalTime, int serviceTime) { strcpy(this->name, name); this->arrivalTime = arrivalTime; this->serviceTime = serviceTime; this->finishTime = arrivalTime + serviceTime; // set finishTime as sum of arrivalTime and serviceTime } // function to display the customer details void displayCustomer() { cout<<"Name: "< 0) // queue is not empty { Customer customer = customerList[0]; // get the customer at the front // loop to shift the elements to left by 1 position from second element to end for(int i=0;i
I need image part
in this program
// C++ program to create and implement FCFS Queue of customers
#include <iostream>
#include <cstring>
using namespace std;
class Customer
{
private:
char name[15];
int arrivalTime;
int serviceTime;
int finishTime;
public:
// function to set name, arrivalTime and serviceTime to specified values
void setCustomer(char *name, int arrivalTime, int serviceTime)
{
strcpy(this->name, name);
this->arrivalTime = arrivalTime;
this->serviceTime = serviceTime;
this->finishTime = arrivalTime + serviceTime; // set finishTime as sum of arrivalTime and serviceTime
}
// function to display the customer details
void displayCustomer()
{
cout<<"Name: "<<name<<", Arrival Time: "<<arrivalTime<<", Service Time: "<<serviceTime<<", Finish Time: "<<finishTime<<endl;
}
};
class FCFSQueue
{
private:
Customer customerList[100];
int length = 0;
public:
// function that returns true if queue is empty else return false
bool isEmpty()
{
return length == 0;
}
// function to return number of elements in queue
int GetLength()
{
return length;
}
// function to insert customer at end of queue
void Enqueue(Customer customer)
{
if(length < 100) // queue is not full, insert customer at index length
{
customerList[length] = customer;
length++; // increment length by 1
}
else // queue is full
cout<<"Queue is full"<<endl;
}
// function to remove the customer at the front
void Dequeue()
{
if(length > 0) // queue is not empty
{
Customer customer = customerList[0]; // get the customer at the front
// loop to shift the elements to left by 1 position from second element to end
for(int i=0;i<length-1;i++)
customerList[i] = customerList[i+1];
length--; // decrement length by 1
// display the customer removed
cout<<"Customer removed: "<<endl;
customer.displayCustomer();
}
else // queue is empty
cout<<"Queue is empty"<<endl;
}
};
int main()
{
FCFSQueue queue; // create an empty queue
Customer customer;
cout<<"Queue is empty: "<<boolalpha<<queue.isEmpty()<<endl;
cout<<"Number of customers in queue: "<<queue.GetLength()<<endl;
// add customers
cout<<"\nAdding customers"<<endl;
customer.setCustomer("Adam Hope", 5, 15);
queue.Enqueue(customer);
customer.setCustomer("Harry Johnson", 7, 18);
queue.Enqueue(customer);
customer.setCustomer("John Smith", 10, 12);
queue.Enqueue(customer);
cout<<"Queue is empty: "<<boolalpha<<queue.isEmpty()<<endl;
cout<<"Number of customers in queue: "<<queue.GetLength()<<endl;
cout<<"\nRemoving all customers:"<<endl;
// loop to remove all customers from queue
while(!queue.isEmpty())
{
queue.Dequeue();
}
cout<<"Queue is empty: "<<boolalpha<<queue.isEmpty()<<endl;
cout<<"Number of customers in queue: "<<queue.GetLength()<<endl;
return 0;
}


Step by step
Solved in 2 steps with 1 images

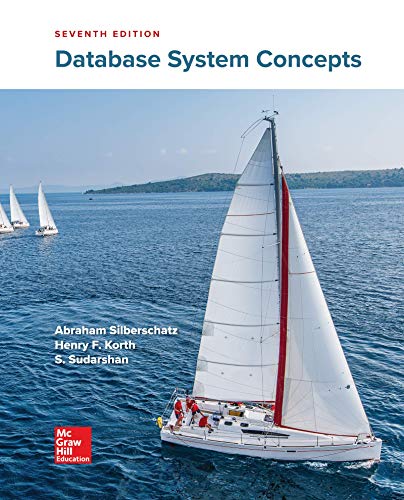
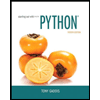
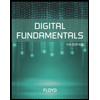
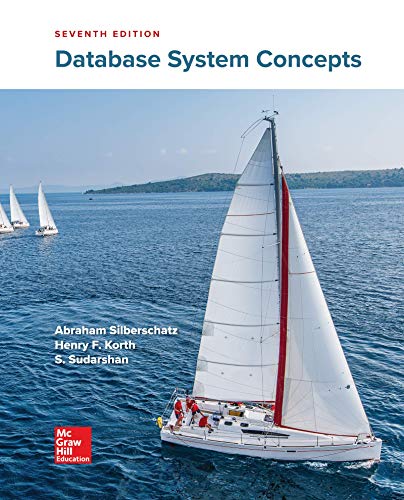
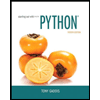
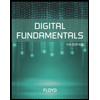
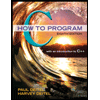
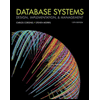
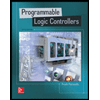