I need help with this Java problem to output like in this image below (Not the one highlighted in yellow): Output numbers in reverse Write a program that reads a list of integers and outputs those integers in reverse. The input begins with an integer indicating the number of integers that follow. For coding simplicity, follow each output integer by a comma, including the last one. Assume that the list will always contain fewer than 20 integers. Ex: If the input is: 5 2 4 6 8 10 the output is: 10,8,6,4,2, To achieve the above, first read the integers into an array. Then output the array in reverse. Java Code: import java.util.Scanner; public class LabProgram { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int[] userList = new int[20]; // List of numElement integers specified by the user int numElements; // Number of integers in user's list numElements = scnr.nextInt(); for (int i = 0; i < numElements; i++) { userList[i] = scnr.nextInt(); } for (int i = numElements - 1; i >= 0; i--) { System.out.print(userList[i]); if (i > 0) { System.out.print(","); } } } }
I need help with this Java problem to output like in this image below (Not the one highlighted in yellow):
Output numbers in reverse
Write a program that reads a list of integers and outputs those integers in reverse. The input begins with an integer indicating the number of integers that follow. For coding simplicity, follow each output integer by a comma, including the last one. Assume that the list will always contain fewer than 20 integers.
Ex: If the input is:
5 2 4 6 8 10the output is:
10,8,6,4,2,To achieve the above, first read the integers into an array. Then output the array in reverse.
Java Code:
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int[] userList = new int[20]; // List of numElement integers specified by the user
int numElements; // Number of integers in user's list
numElements = scnr.nextInt();
for (int i = 0; i < numElements; i++) {
userList[i] = scnr.nextInt();
}
for (int i = numElements - 1; i >= 0; i--) {
System.out.print(userList[i]);
if (i > 0) {
System.out.print(",");
}
}
}
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

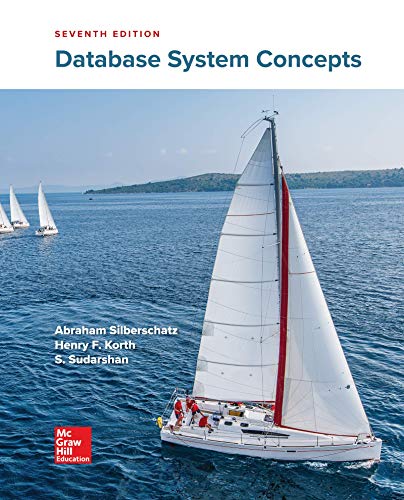
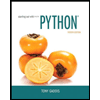
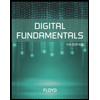
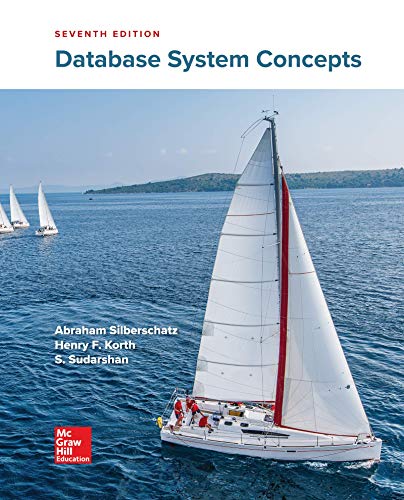
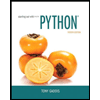
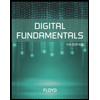
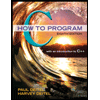
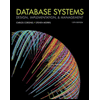
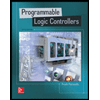