Given a sorted list of integers, output the middle integer. A negative number indicates the end of the input (the negative number is not a part of the sorted list). Assume the number of integers is always odd. Ex: If the input is: 2 3 4 8 11 -1 the output is: Middle item: 4 The maximum number of list values for any test case should not exceed 9. If exceeded, output "Too many numbers". Hint: First read the data into an array. Then, based on the array's size, find the middle item. //LabProgram.Java import java.util.Scanner; public class LabProgram { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int[] userValues = new int[9]; int size = 0; while (size < 9) { int num = scnr.nextInt(); if (num < 0) { break; } userValues[size] = num; size++; } if (size > 8) { System.out.println("Too many numbers"); } else { int middleIndex = size / 2; System.out.println("Middle item: " + userValues[middleIndex]); } } }
I need help with this Java problem to output like in this image below (Not the one highlighted in yellow):
Middle item
Given a sorted list of integers, output the middle integer. A negative number indicates the end of the input (the negative number is not a part of the sorted list). Assume the number of integers is always odd.
Ex: If the input is:
2 3 4 8 11 -1the output is:
Middle item: 4The maximum number of list values for any test case should not exceed 9. If exceeded, output "Too many numbers".
Hint: First read the data into an array. Then, based on the array's size, find the middle item.
//LabProgram.Java
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int[] userValues = new int[9];
int size = 0;
while (size < 9) {
int num = scnr.nextInt();
if (num < 0) {
break;
}
userValues[size] = num;
size++;
}
if (size > 8) {
System.out.println("Too many numbers");
}
else {
int middleIndex = size / 2;
System.out.println("Middle item: " + userValues[middleIndex]);
}
}
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

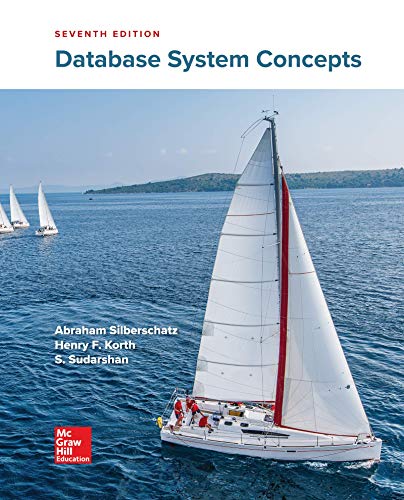
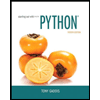
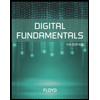
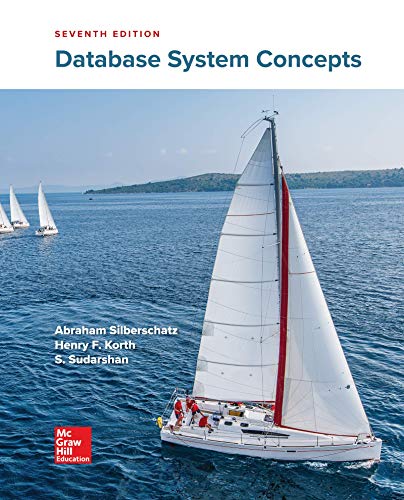
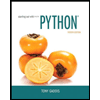
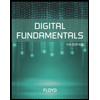
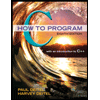
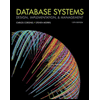
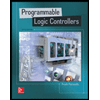