I need help in solving this programming problem. Thank you! // ********************* MODIFY ME!!! ********************* #include #include #include "lab4_ex1.h" // Removes or cleans the dangling 'new line' character in stdin // @param void // @return none void flush() { char c; while ((c = getchar()) != '\n' && c != EOF); } // Creates the course (one course only) by asking the for user input // @param num course number, e.g. 1,2,3 // @return course Course createCourse(int num) { Course course; //input code here return course; } // Displays the course // @param course number, e.g. 1, 2, 3 // @return none void displayCourse(Course course) { printf("course name: %s\n", course.name); printf("course grade: %0.2f\n", course.grade); printf("course units: %d\n", course.unit); } // Computes the GPA from three courses, based on the formula in README.md // @param courses[TOTAL_COURSE] // @return GPA float computeGPA(Course courses[TOTAL_COURSE]) { // ********************* YOUR CODE HERE ********************* return 0.0f; } // Creates the student by asking for user input // @param none // @return student structure with id, name, degree, courses, and GPA Student createStudent() { Student student; // input code here return student; } // Displays the student // @param student struct // @return none void displayStudent(Student student) { printf("student name: %s\n", student.name); printf("ID number: %d\n", student.id); printf("degree: %s\n", student.degree); for (int i = 0; i < TOTAL_COURSE; i++) { displayCourse(student.courses[i]); } } // Displays the student with GPA // @param student struct // @return none void displayStudentGPA(Student student) { printf("%s with ID number %d has a GPA of %.3f\n", student.name, student.id, student.GPA); } this is the header file // ********************* MODIFY ME!!! ********************* #ifndef lab4_ex1_h #define lab4_ex1_h #define TOTAL_COURSE 3 #define BUFFER_SIZE 80 typedef struct Course{ char name[BUFFER_SIZE]; int unit; float grade; } Course; typedef struct Student{ int id; char name[BUFFER_SIZE]; char degree[BUFFER_SIZE]; Course courses[TOTAL_COURSE]; float GPA; } Student; Student createStudent(); Course createCourse(int); void displayStudent(Student); void displayStudentGPA(Student student); void displayCourse(Course); float computeGPA(Course courses[TOTAL_COURSE]); void flush(); #endif this is the main.cpp file #include // needed by printf, scanf #include // needed by malloc #include "lab4_ex1.h" int main(void) { int N; // Get the number of students N do { printf("Enter number of students to record: "); scanf("%d", &N); } while (N < 1); flush(); // Allocate memory to the data of students Student *record = (Student *)malloc(N * sizeof(Student)); // Create student record/s for (int i = 0; i < N; i++) record[i] = createStudent(); // Display GPA for all student records for (int i = 0; i < N; i++) displayStudentGPA(record[i]); free(record); return 0; }
I need help in solving this programming problem. Thank you!
// ********************* MODIFY ME!!! *********************
#include <stdio.h>
#include <string.h>
#include "lab4_ex1.h"
// Removes or cleans the dangling 'new line' character in stdin
// @param void
// @return none
void flush()
{
char c;
while ((c = getchar()) != '\n' && c != EOF);
}
// Creates the course (one course only) by asking the for user input
// @param num course number, e.g. 1,2,3
// @return course
Course createCourse(int num)
{
Course course;
//input code here
return course;
}
// Displays the course
// @param course number, e.g. 1, 2, 3
// @return none
void displayCourse(Course course)
{
printf("course name: %s\n", course.name);
printf("course grade: %0.2f\n", course.grade);
printf("course units: %d\n", course.unit);
}
// Computes the GPA from three courses, based on the formula in README.md
// @param courses[TOTAL_COURSE]
// @return GPA
float computeGPA(Course courses[TOTAL_COURSE])
{
// ********************* YOUR CODE HERE *********************
return 0.0f;
}
// Creates the student by asking for user input
// @param none
// @return student structure with id, name, degree, courses, and GPA
Student createStudent()
{
Student student;
// input code here
return student;
}
// Displays the student
// @param student struct
// @return none
void displayStudent(Student student)
{
printf("student name: %s\n", student.name);
printf("ID number: %d\n", student.id);
printf("degree: %s\n", student.degree);
for (int i = 0; i < TOTAL_COURSE; i++)
{
displayCourse(student.courses[i]);
}
}
// Displays the student with GPA
// @param student struct
// @return none
void displayStudentGPA(Student student)
{
printf("%s with ID number %d has a GPA of %.3f\n", student.name, student.id, student.GPA);
}
this is the header file
// ********************* MODIFY ME!!! *********************
#ifndef lab4_ex1_h
#define lab4_ex1_h
#define TOTAL_COURSE 3
#define BUFFER_SIZE 80
typedef struct Course{
char name[BUFFER_SIZE];
int unit;
float grade;
} Course;
typedef struct Student{
int id;
char name[BUFFER_SIZE];
char degree[BUFFER_SIZE];
Course courses[TOTAL_COURSE];
float GPA;
} Student;
Student createStudent();
Course createCourse(int);
void displayStudent(Student);
void displayStudentGPA(Student student);
void displayCourse(Course);
float computeGPA(Course courses[TOTAL_COURSE]);
void flush();
#endif
this is the main.cpp file
#include <stdio.h> // needed by printf, scanf
#include <stdlib.h> // needed by malloc
#include "lab4_ex1.h"
int main(void)
{
int N;
// Get the number of students N
do
{
printf("Enter number of students to record: ");
scanf("%d", &N);
} while (N < 1);
flush();
// Allocate memory to the data of students
Student *record = (Student *)malloc(N * sizeof(Student));
// Create student record/s
for (int i = 0; i < N; i++)
record[i] = createStudent();
// Display GPA for all student records
for (int i = 0; i < N; i++)
displayStudentGPA(record[i]);
free(record);
return 0;
}



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

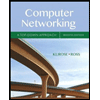
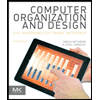
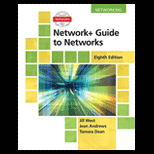
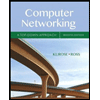
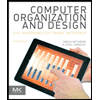
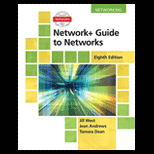
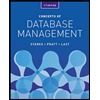
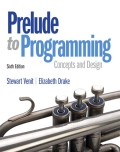
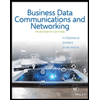