I need help in constructing the function (indicated in the bold and italics present in the end). Following are the given struct that can't be changed // name structure data type struct nameTag { String last; String first; }; typedef struct nameTag nameType; // vaccinee structure data type struct vaccineeTag { int ID; // ID number of the vaccinee, note that this number is system-generated (i.e., not a user input) nameType name; // name of vacinee int age; // age of vacinee int bool_frontliner; // 0 means not frontliner, 1 means frontliner int bool_comorbidity; // 0 means no comorbidity, 1 means with comorbiditity int priority; // 0 means priority not yet set, 1 to 4 means priority is set // where 1 is the highest priority and 4 is the lowest priority }; typedef struct vaccineeTag vaccineeType; Instructions void Set_Priority(vaccineeType *ptr_vaccinee) Implement Set_Priority() which will determine and store the value of the structure member priority. Assume that the priority member contains a value of 0 (which means it is not yet determined) before the call to Set_Priority(). Note that you’ll need to access the structure members indirectly via the structure pointer ptr_vaccinee formal parameter. Vaccinees are categorized based on a predetermined priority scheme shown in the table below: Priority (1 is the highest, 4 is the lowest) Condition 1 person is a frontliner 2 person is a senior citizen, i.e., the age is 60 or above 3 person has comorbidity (note: the actual comorbidity is not included in the data) 4 none of the above It is possible that a person can be a frontliner, a senior citizen and has comorbidity, the priority will be 1. If the person is a senior citizen, and has comorbidity, the priority is 2. Some examples are given below with reference to the sample data in Figure 1: Example #1: The priority of SANTOS, JOSE (age 45, not a frontliner, no comorbidity) will be set to 4. Example #2: The priority of ORTIZ, MANNY (age 62, imagine he’s a medical doctor, and with comorbidity) will be set to 1. Example #3: The priority of ALONZO, LUISA (age 65, not a frontliner, and with comorbidity) will be set to 2. Example #4: The priority of CRUZ, JAIME (age 38, not a frontliner, and with comorbidity) will be set to 3. The figure below shows the updated VACCINEE[] contents with the priority values (in bold font) set accordingly. Index Element (structure) contents 0 101 SANTOS JOSE 45 0 0 4 1 102 ORTIZ MANNY 62 1 1 1 2 103 ALONZO LUISA 65 0 1 2 . . 12 112 CRUZ CORNELIA 50 0 1 3 13 114 SY ALEXA 33 1 0 1 14 115 CRUZ JAIME 38 0 1 3 /* TO DO #2: Implement Set_Priority() as described in the problem specs. You'll have to acccess the structure members indirectly via the formal structure pointer parameter ptr_vaccine. NOTE: Set_Priority() is called inside the Prioritize() function. See test_functions.c. @param: ptr_vaccine contains the memory address of a variable containing data about a vaccinee returns: none (no return value) */ void Set_Priority(vaccineeType *ptr_vaccinee) { /* Declare your own local variables. Do NOT call printf() or scanf() in this function. */ }
I need help in constructing the function (indicated in the bold and italics present in the end).
Following are the given struct that can't be changed
// name structure data type
struct nameTag {
String last;
String first;
};
typedef struct nameTag nameType;
// vaccinee structure data type
struct vaccineeTag {
int ID; // ID number of the vaccinee, note that this number is system-generated (i.e., not a user input)
nameType name; // name of vacinee
int age; // age of vacinee
int bool_frontliner; // 0 means not frontliner, 1 means frontliner
int bool_comorbidity; // 0 means no comorbidity, 1 means with comorbiditity
int priority; // 0 means priority not yet set, 1 to 4 means priority is set
// where 1 is the highest priority and 4 is the lowest priority
};
typedef struct vaccineeTag vaccineeType;
Instructions
void Set_Priority(vaccineeType *ptr_vaccinee)
Implement Set_Priority() which will determine and store the value of the structure member priority. Assume that the
priority member contains a value of 0 (which means it is not yet determined) before the call to Set_Priority(). Note that you’ll need to access the structure members indirectly via the structure pointer ptr_vaccinee formal parameter.
Vaccinees are categorized based on a predetermined priority scheme shown in the table below:
Priority (1 is the highest, 4 is the lowest) Condition
1 person is a frontliner 2 person is a senior citizen, i.e., the age is 60 or above 3 person has comorbidity (note: the actual comorbidity is not included in the data) 4 none of the above |
It is possible that a person can be a frontliner, a senior citizen and has comorbidity, the priority will be 1. If the person is a senior citizen, and has comorbidity, the priority is 2. Some examples are given below with reference to the sample data in Figure 1:
Example #1: The priority of SANTOS, JOSE (age 45, not a frontliner, no comorbidity) will be set to 4.
Example #2: The priority of ORTIZ, MANNY (age 62, imagine he’s a medical doctor, and with comorbidity) will be set to 1.
Example #3: The priority of ALONZO, LUISA (age 65, not a frontliner, and with comorbidity) will be set to 2.
Example #4: The priority of CRUZ, JAIME (age 38, not a frontliner, and with comorbidity) will be set to 3.
The figure below shows the updated VACCINEE[] contents with the priority values (in bold font) set accordingly.
Index | Element (structure) contents |
0 | 101 SANTOS JOSE 45 0 0 4 |
1 | 102 ORTIZ MANNY 62 1 1 1 |
2 | 103 ALONZO LUISA 65 0 1 2 |
. | |
. | |
12 | 112 CRUZ CORNELIA 50 0 1 3 |
13 | 114 SY ALEXA 33 1 0 1 |
14 | 115 CRUZ JAIME 38 0 1 3 |
/*
TO DO #2: Implement Set_Priority() as described in the problem specs.
You'll have to acccess the structure members indirectly via the formal
structure pointer parameter ptr_vaccine.
NOTE: Set_Priority() is called inside the Prioritize() function.
See test_functions.c.
@param: ptr_vaccine contains the memory address of a variable containing
data about a vaccinee
returns: none (no return value)
*/
void Set_Priority(vaccineeType *ptr_vaccinee)
{
/*
Declare your own local variables.
Do NOT call printf() or scanf() in this function.
*/
}

Step by step
Solved in 3 steps with 1 images

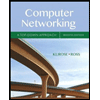
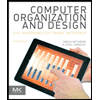
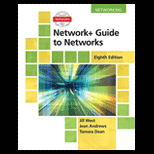
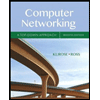
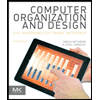
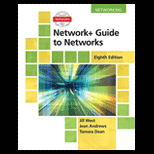
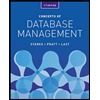
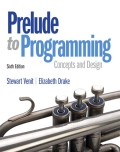
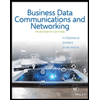