I need help finalizing this C program assignment. [This is not a graded assignment] my code here for each of the 3 .files cis158g1.c #include #include "grades.h" int main() { Student students[] = { {"Adams, Tom", {95, 93, 95, 99, 94}, 90, 85, 80, 75}, {"Curry, Jane", {95, 86, 88, 87, 89}, 90, 85, 80, 75}, {"Franklin, John", {70, 50, 65, 50, 57}, 90, 85, 80, 75}, {"George, Pat", {85, 78, 81, 82, 88}, 90, 85, 80, 75}, {"Keene, Mary", {85, 72, 75, 68, 75}, 90, 85, 80, 75}, {"Kraft, Martin", {80, 85, 80, 88, 93}, 90, 85, 80, 75}, {"Martin, James", {75, 65, 65, 52, 55}, 90, 85, 80, 75}, {"Oakley, Ann", {95, 85, 95, 88, 92}, 90, 85, 80, 75}, {"Smith, Luke", {95, 85, 75, 81, 75}, 90, 85, 80, 75}, }; int num_students = sizeof(students) / sizeof(Student); int i; for (i = 0; i < num_students; i++) { Student student = students[i]; double grade = calculate_final_grade(&student); char* letter_grade = get_letter_grade(grade); char message[50]; get_message(letter_grade, message); printf("%s %s\n", student.name, message); } return 0; } grades.h typedef struct { char* name; int grades[5]; int assignments; int midterm; int final_exam; int participation; } Student; double calculate_final_grade(Student* student); char* get_letter_grade(double grade); grades.c #include #include #include "grades.h" double calculate_final_grade(Student* student) { double assignment_weight = 0.40; double midterm_weight = 0.15; double final_exam_weight = 0.35; double participation_weight = 0.10; double total_grade = 0.0; int i; for (i = 0; i < 5; i++) { total_grade += student->grades[i]; } double average_grade = total_grade / 5.0; total_grade = (average_grade * assignment_weight) + (student->midterm * midterm_weight) + (student->final_exam * final_exam_weight) + (student->participation * participation_weight); return total_grade; } char* get_letter_grade(double grade) { if (grade >= 90.0) { return "A"; } else if (grade >= 80.0) { return "B"; } else if (grade >= 70.0) { return "C"; } else if (grade >= 60.0) { return "D"; } else { return "F"; } } Makefile: #Create binary cis158g1: cis158g1.o grades.o gcc -o cis158g1 cis158g1.o grades.o -lm #Create object file for cis158g1.c cis158g1.o: cis158g1.c gcc -c cis158g1.c #Create object file for grades.c grades.o: grades.c gcc -c grades.c #Delete the all of the object files clean: rm *.o I get an undefined reference to 'get_message' error 1d returned 1 exit staus when running the 'make' command. Please make fixes and apply changes if needed to fully follow the assignment. This is close to being finished!
I need help finalizing this C program assignment. [This is not a graded assignment]
my code here for each of the 3 .files
cis158g1.c
#include <stdio.h>
#include "grades.h"
int main() {
Student students[] = {
{"Adams, Tom", {95, 93, 95, 99, 94}, 90, 85, 80, 75},
{"Curry, Jane", {95, 86, 88, 87, 89}, 90, 85, 80, 75},
{"Franklin, John", {70, 50, 65, 50, 57}, 90, 85, 80, 75},
{"George, Pat", {85, 78, 81, 82, 88}, 90, 85, 80, 75},
{"Keene, Mary", {85, 72, 75, 68, 75}, 90, 85, 80, 75},
{"Kraft, Martin", {80, 85, 80, 88, 93}, 90, 85, 80, 75},
{"Martin, James", {75, 65, 65, 52, 55}, 90, 85, 80, 75},
{"Oakley, Ann", {95, 85, 95, 88, 92}, 90, 85, 80, 75},
{"Smith, Luke", {95, 85, 75, 81, 75}, 90, 85, 80, 75},
};
int num_students = sizeof(students) / sizeof(Student);
int i;
for (i = 0; i < num_students; i++) {
Student student = students[i];
double grade = calculate_final_grade(&student);
char* letter_grade = get_letter_grade(grade);
char message[50];
get_message(letter_grade, message);
printf("%s %s\n", student.name, message);
}
return 0;
}
grades.h
typedef struct {
char* name;
int grades[5];
int assignments;
int midterm;
int final_exam;
int participation;
} Student;
double calculate_final_grade(Student* student);
char* get_letter_grade(double grade);
grades.c
#include <stdlib.h>
#include <stdio.h>
#include "grades.h"
double calculate_final_grade(Student* student) {
double assignment_weight = 0.40;
double midterm_weight = 0.15;
double final_exam_weight = 0.35;
double participation_weight = 0.10;
double total_grade = 0.0;
int i;
for (i = 0; i < 5; i++) {
total_grade += student->grades[i];
}
double average_grade = total_grade / 5.0;
total_grade = (average_grade * assignment_weight) + (student->midterm * midterm_weight)
+ (student->final_exam * final_exam_weight) + (student->participation * participation_weight);
return total_grade;
}
char* get_letter_grade(double grade) {
if (grade >= 90.0) {
return "A";
} else if (grade >= 80.0) {
return "B";
} else if (grade >= 70.0) {
return "C";
} else if (grade >= 60.0) {
return "D";
} else {
return "F";
}
}
Makefile:
#Create binary
cis158g1: cis158g1.o grades.o
gcc -o cis158g1 cis158g1.o grades.o -lm
#Create object file for cis158g1.c
cis158g1.o: cis158g1.c
gcc -c cis158g1.c
#Create object file for grades.c
grades.o: grades.c
gcc -c grades.c
#Delete the all of the object files
clean:
rm *.o
I get an undefined reference to 'get_message' error 1d returned 1 exit staus when running the 'make' command.
Please make fixes and apply changes if needed to fully follow the assignment. This is close to being finished!

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 4 images

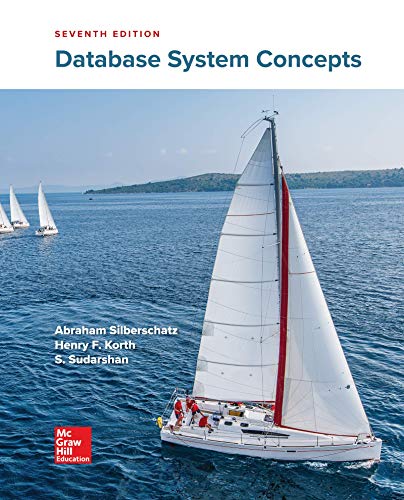
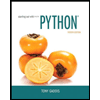
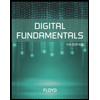
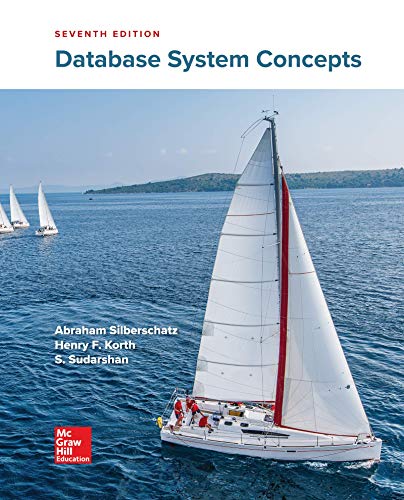
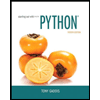
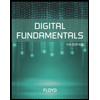
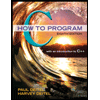
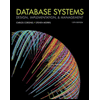
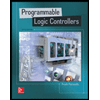