I need help explaining each and every line of the code functionality ========================================================== import React, { useState, useRef } from "react"; import Modal from "react-modal"; import { Cropper } from "react-advanced-cropper"; import "react-advanced-cropper/dist/style.css"; import { resizeImage } from "../util/Helper"; const ImageCropper = ({ modalIsOpen, closeModal, uploadImageData, setImageSrc, }) => { //customstyles constant is an object that defines the custom styles for the modal const customStyles = { content: { top: "50%", left: "50%", right: "auto", bottom: "auto", marginRight: "-50%", transform: "translate(-50%, -50%)", border: "none", backgroundColor: "transparent", }, }; const cropperRef = useRef(); const [image] = useState(uploadImageData); // gets the canvas and resize the image // sets the resized image as the new source and close the modal const cropImage = async () => { const cropper = cropperRef.current; if (cropper) { const canvas = cropper.getCanvas(); const resizedImage = await resizeImage(canvas.toDataURL()); setImageSrc(resizedImage); closeModal(); } }; return ( Crop Photo Cancel Crop ) }; export default ImageCropper;
I need help explaining each and every line of the code functionality ========================================================== import React, { useState, useRef } from "react"; import Modal from "react-modal"; import { Cropper } from "react-advanced-cropper"; import "react-advanced-cropper/dist/style.css"; import { resizeImage } from "../util/Helper"; const ImageCropper = ({ modalIsOpen, closeModal, uploadImageData, setImageSrc, }) => { //customstyles constant is an object that defines the custom styles for the modal const customStyles = { content: { top: "50%", left: "50%", right: "auto", bottom: "auto", marginRight: "-50%", transform: "translate(-50%, -50%)", border: "none", backgroundColor: "transparent", }, }; const cropperRef = useRef(); const [image] = useState(uploadImageData); // gets the canvas and resize the image // sets the resized image as the new source and close the modal const cropImage = async () => { const cropper = cropperRef.current; if (cropper) { const canvas = cropper.getCanvas(); const resizedImage = await resizeImage(canvas.toDataURL()); setImageSrc(resizedImage); closeModal(); } }; return ( Crop Photo Cancel Crop ) }; export default ImageCropper;
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I need help explaining each and every line of the code functionality
==========================================================
import React, { useState, useRef } from "react";
import Modal from "react-modal";
import { Cropper } from "react-advanced-cropper";
import "react-advanced-cropper/dist/style.css";
import { resizeImage } from "../util/Helper";
const ImageCropper = ({ modalIsOpen, closeModal, uploadImageData, setImageSrc, }) => {
//customstyles constant is an object that defines the custom styles for the modal
const customStyles = {
content: {
top: "50%",
left: "50%",
right: "auto",
bottom: "auto",
marginRight: "-50%",
transform: "translate(-50%, -50%)",
border: "none",
backgroundColor: "transparent",
},
};
const cropperRef = useRef();
const [image] = useState(uploadImageData);
// gets the canvas and resize the image
// sets the resized image as the new source and close the modal
const cropImage = async () => {
const cropper = cropperRef.current;
if (cropper) {
const canvas = cropper.getCanvas();
const resizedImage = await resizeImage(canvas.toDataURL());
setImageSrc(resizedImage);
closeModal();
}
};
return (
<Modal
isOpen={modalIsOpen}
onRequestClose={closeModal}
style={customStyles}
>
<div className="bg-white shadow rounded-lg mb-6 p-5">
<div className="text-gray-600 text-lg font-semibold mt-2 mb-7">
Crop Photo
</div>
<Cropper
ref={cropperRef}
src={image}
className={"cropper"}
aspectRatio={1}
/>
<footer className="flex justify-end mt-8 gap-2">
<button
className="w-full px-4 py-2 tracking-wide text-white transition-colors duration-200 transform bg-gray-400 rounded-md hover:bg-gray-600 focus:outline-none focus:bg-purple-400 focus:ring focus:ring-purple-300 focus:ring-opacity-50"
onClick={closeModal}
>
Cancel
</button>
<button
className="w-full px-4 py-2 tracking-wide text-white transition-colors duration-200 transform bg-purple-600 rounded-md hover:bg-purple-400 focus:outline-none focus:bg-purple-400 focus:ring focus:ring-purple-300 focus:ring-opacity-50"
onClick={cropImage}
>
Crop
</button>
</footer>
</div>
</Modal>
)
};
export default ImageCropper;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
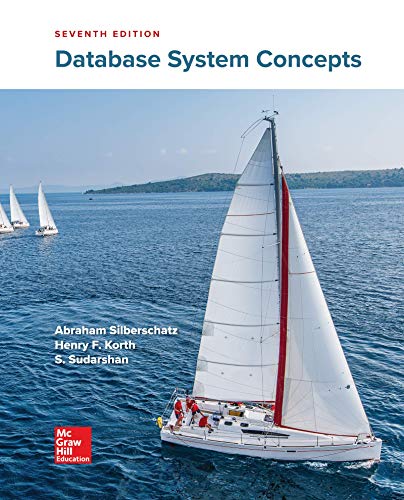
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
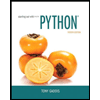
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
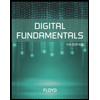
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
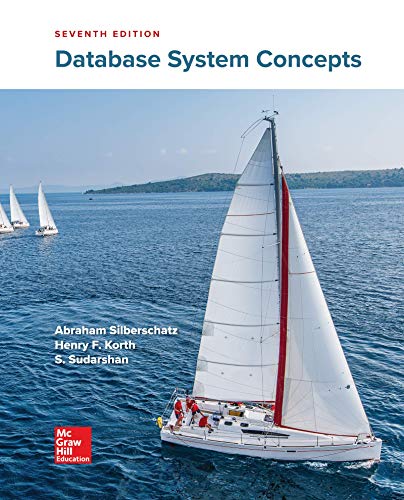
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
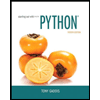
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
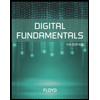
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
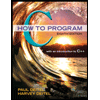
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
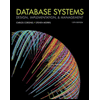
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
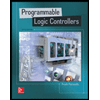
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education