I need a line by line explination as to how the below Python program works. I am having a hard time understanding how the code under the comment "put this code into the main method" works with the rest of the program. Python program source code: import math class Complex(object): def __init__(self, real, imaginary): self.real = real self.imaginary = imaginary def __add__(self, no): # (a + ib) + (c + id) = (a + c) + i(b + d) real = self.real + no.real imaginary = self.imaginary + no.imaginary return Complex(real, imaginary) def __sub__(self, no): # (a + ib) - (c + id) = (a - c) + i(b - d) real = self.real - no.real imaginary = self.imaginary - no.imaginary return Complex(real, imaginary) def __mul__(self, no): # (a + ib) * (c + id) = (ac - bd) + i(ad + bc) real = self.real * no.real - self.imaginary * no.imaginary imaginary = self.real * no.imaginary + self.imaginary * no.real return Complex(real, imaginary) def __truediv__(self, no): # (a+ib)/(c+id) = ((ac + bd) + i(bc - ad)) / (c^2 + d^2) real = (self.real * no.real + self.imaginary * no.imaginary) / (no.real**2 + no.imaginary**2) imaginary = (self.imaginary * no.real - self.real * no.imaginary) / (no.real**2 + no.imaginary**2) return Complex(real, imaginary) def mod(self): # a + ib = sqrt(a^2 + b^2), sqrt(c^2 + d^2) real = math.sqrt((self.real ** 2 + self.imaginary ** 2)) return Complex(real, 0) def __str__(self): #Real and imaginary numbers should be correct up to two decimal places. if self.imaginary >= 0: result = '{0:.2f} + {1:.2f}i'.format(self.real, self.imaginary) else: result = '{0:.2f} - {1:.2f}i'.format(self.real, abs(self.imaginary)) return result # Put this code into the main method C = map(float, input("Input a complex number e.g.(2 spacebar 1): ").split()) D = map(float, input("Input a complex number e.g.(5 spacebar 6): ").split()) x = Complex(*C) y = Complex(*D) print('\n'.join(map(str, [x+y, x-y, x*y, x/y, x.mod(), y.mod()]))) Thank you
I need a line by line explination as to how the below Python
Python program source code:
import math
class Complex(object):
def __init__(self, real, imaginary):
self.real = real
self.imaginary = imaginary
def __add__(self, no):
# (a + ib) + (c + id) = (a + c) + i(b + d)
real = self.real + no.real
imaginary = self.imaginary + no.imaginary
return Complex(real, imaginary)
def __sub__(self, no):
# (a + ib) - (c + id) = (a - c) + i(b - d)
real = self.real - no.real
imaginary = self.imaginary - no.imaginary
return Complex(real, imaginary)
def __mul__(self, no):
# (a + ib) * (c + id) = (ac - bd) + i(ad + bc)
real = self.real * no.real - self.imaginary * no.imaginary
imaginary = self.real * no.imaginary + self.imaginary * no.real
return Complex(real, imaginary)
def __truediv__(self, no):
# (a+ib)/(c+id) = ((ac + bd) + i(bc - ad)) / (c^2 + d^2)
real = (self.real * no.real + self.imaginary * no.imaginary) / (no.real**2 + no.imaginary**2)
imaginary = (self.imaginary * no.real - self.real * no.imaginary) / (no.real**2 + no.imaginary**2)
return Complex(real, imaginary)
def mod(self):
# a + ib = sqrt(a^2 + b^2), sqrt(c^2 + d^2)
real = math.sqrt((self.real ** 2 + self.imaginary ** 2))
return Complex(real, 0)
def __str__(self):
#Real and imaginary numbers should be correct up to two decimal places.
if self.imaginary >= 0:
result = '{0:.2f} + {1:.2f}i'.format(self.real, self.imaginary)
else:
result = '{0:.2f} - {1:.2f}i'.format(self.real, abs(self.imaginary))
return result
# Put this code into the main method
C = map(float, input("Input a complex number e.g.(2 spacebar 1): ").split())
D = map(float, input("Input a complex number e.g.(5 spacebar 6): ").split())
x = Complex(*C)
y = Complex(*D)
print('\n'.join(map(str, [x+y, x-y, x*y, x/y, x.mod(), y.mod()])))
Thank you
Unlock instant AI solutions
Tap the button
to generate a solution
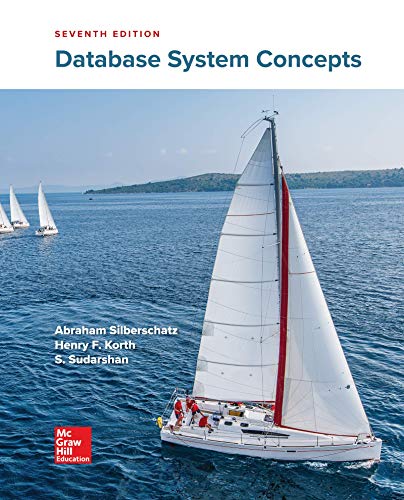
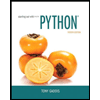
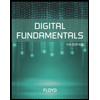
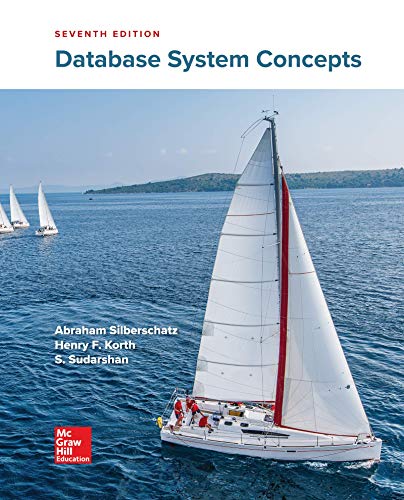
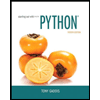
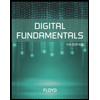
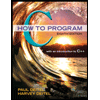
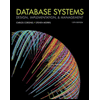
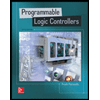