C++ Visual Studio 2019 Complete #13. Dependent #1 Employee and ProductionWorker classes showing below. Modify the Employee and ProductionWorker classes so they throw exceptions when the following errors occur: The Employee class should throw an exception named InvalidEmployeeNumber when it receives an employee number that is less than 0 or greater than 9999. The ProductionWorker class should throw an exception named InvalidShift when it receives an invalid shift. The ProductionWorker class should throw an exception named InvalidPayRate when it receives a negative number for the hourly pay rate. Write a driver program that demonstrates how each of these exception conditions works. #1 Employee and ProductionWorker classes #include #include #include using namespace std; class Employee { private: string name; // Employee name string number; // Employee number string hireDate; // Hire date public: // Default constructor Employee() { name = ""; number = ""; hireDate = ""; } // Constructor Employee(string aName, string aNumber, string aDate) { name = aName; number = aNumber; hireDate = aDate; } // Mutators void setName(string n) { name = n; } void setNumber(string num) { number = num; } void setHireDate(string date) { hireDate = date; } // Accessors string getName() const { return name; } string getNumber() const { return number; } string getHireDate() const { return hireDate; } }; // Specification for the ProductionWorker Class #include using namespace std; class ProductionWorker : public Employee { private: int shift; // The worker's shift double payRate; // The worker's hourly pay rate public: // Default constructor ProductionWorker() : Employee() { shift = 0; payRate = 0.0; } // Constructor ProductionWorker(string aName, string aNumber, string aDate, int aShift, double aPayRate) : Employee(aName, aNumber, aDate) { shift = aShift; payRate = aPayRate; } // Mutators void setShift(int s) { shift = s; } void setPayRate(double r) { payRate = r; } // Accessors int getShiftNumber() const { return shift; } string getShiftName() const { if (shift == 1) return "Day"; else if (shift == 2) return "Night"; else return "Invalid"; } double getPayRate() const { return payRate; } }; // Employee and ProductionWorker classes // Function prototype void displayInfo(ProductionWorker); int main() { ProductionWorker pw("John Jones", "123", "10/12/2010", 2, 18.00); displayInfo(pw); return 0; } //****************************************************** // The displayInfo function displays a production * // worker's employment information. * //****************************************************** void displayInfo(ProductionWorker e) { cout << setprecision(2) << fixed << showpoint; cout << "Name: " << e.getName() << endl; cout << "Employee number: " << e.getNumber() << endl; cout << "Hire date: " << e.getHireDate() << endl; cout << "Shift: " << e.getShiftName() << endl; cout << "Shift number: " << e.getShiftNumber() << endl; cout << "Pay rate: " << e.getPayRate() << endl; }
C++ Visual Studio 2019
Complete #13. Dependent #1 Employee and ProductionWorker classes showing below. Modify the Employee and ProductionWorker classes so they throw exceptions when the following errors occur:
- The Employee class should throw an exception named InvalidEmployeeNumber when it receives an employee number that is less than 0 or greater than 9999.
- The ProductionWorker class should throw an exception named InvalidShift when it receives an invalid shift.
- The ProductionWorker class should throw an exception named InvalidPayRate when it receives a negative number for the hourly pay rate.
Write a driver program that demonstrates how each of these exception conditions works.
#1 Employee and ProductionWorker classes
#include <string>
#include <iostream>
#include <iomanip>
using namespace std;
class Employee
{
private:
string name; // Employee name
string number; // Employee number
string hireDate; // Hire date
public:
// Default constructor
Employee()
{
name = ""; number = ""; hireDate = "";
}
// Constructor
Employee(string aName, string aNumber, string aDate)
{
name = aName; number = aNumber; hireDate = aDate;
}
// Mutators
void setName(string n)
{
name = n;
}
void setNumber(string num)
{
number = num;
}
void setHireDate(string date)
{
hireDate = date;
}
// Accessors
string getName() const
{
return name;
}
string getNumber() const
{
return number;
}
string getHireDate() const
{
return hireDate;
}
};
// Specification for the ProductionWorker Class
#include <string>
using namespace std;
class ProductionWorker : public Employee
{
private:
int shift; // The worker's shift
double payRate; // The worker's hourly pay rate
public:
// Default constructor
ProductionWorker() : Employee()
{
shift = 0; payRate = 0.0;
}
// Constructor
ProductionWorker(string aName, string aNumber, string aDate,
int aShift, double aPayRate) : Employee(aName, aNumber, aDate)
{
shift = aShift; payRate = aPayRate;
}
// Mutators
void setShift(int s)
{
shift = s;
}
void setPayRate(double r)
{
payRate = r;
}
// Accessors
int getShiftNumber() const
{
return shift;
}
string getShiftName() const
{
if (shift == 1)
return "Day";
else if (shift == 2)
return "Night";
else
return "Invalid";
}
double getPayRate() const
{
return payRate;
}
};
// Employee and ProductionWorker classes
// Function prototype
void displayInfo(ProductionWorker);
int main()
{
ProductionWorker pw("John Jones", "123", "10/12/2010", 2, 18.00);
displayInfo(pw);
return 0;
}
//******************************************************
// The displayInfo function displays a production *
// worker's employment information. *
//******************************************************
void displayInfo(ProductionWorker e)
{
cout << setprecision(2) << fixed << showpoint;
cout << "Name: "
<< e.getName() << endl;
cout << "Employee number: "
<< e.getNumber() << endl;
cout << "Hire date: "
<< e.getHireDate() << endl;
cout << "Shift: "
<< e.getShiftName() << endl;
cout << "Shift number: "
<< e.getShiftNumber() << endl;
cout << "Pay rate: "
<< e.getPayRate() << endl;
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 9 images

I m getting this memory error message?
Unhandled exception at 0x776979D2 in Chapter16ExceptionProject.exe: Microsoft C++ exception: InvalidEmployeeNumber at memory location 0x010FF8FC.
![Chapter 16Exception Project.cpp X
Chapter 16Exception Project
40
41
42
43
44
45
46
17
47
100 %
48
10
19
49
co
50
51
51
52
53
54
55
56
57
58
59
60
Autos
Search (Ctrl+E)
Name
▷ num
>
this
void setName(string n)
{
}
void setNumber(string num)
name = n;
→→ Employee
Exception Unhandled
Unhandled exception at Ox776979D2 in Chapter 16ExceptionProject.exe:
Microsoft C++ exception: InvalidEmployeeNumber at memory location
0x010FF8FC.
Copy Details | Start Live Share session...
▷ Exception Settings
hireDate= date;
// Accessors
No issues found
Value
"-3"
P.
Autos Locals Watch 1
Loading symbols for ucrtbased.dll
←
- setNumber(string num)
Search Depth: 3
+ X
Ln: 50 Ch: 1
▾x Exception Settings
Tab
Type
Q std::string
0x010ffe0c {name="John Jones" number... Employee *
* Diagnostic Tools
▷
▷
▷
SPC CRLF
Diagnostics session: 0 seconds
✔ Events
Process Memory ▼S... Priva...
100
▼ + © PE Search (Ctrl+E)
Break When Thrown
0
4 CPU (% of all processors)
Summary Events Memory Usage CPU Usage
Events
Memory Usage
[]Take Snapshot
Show Events (1 of 1)
▷
▷
JavaScript Exceptions
▷
JavaScrint Runtime Exceptions
Call Stack Breakpoints Exception...
10s
C++ Exceptions
Common Language Runtime Except
GPU Memory Access Exceptions
Java Exceptions
Conditions
100
Enable heap profiling (affects performance)
0
д х Live Share
- д х
Command... Immediate... Output Error List
|Q=*P|O•|
Recent contacts (0)
Suggested contacts (0)
4 x
Solution Explorer Git Changes Notifications.
↑ Add to Source Control --2](https://content.bartleby.com/qna-images/question/9588d75b-96d3-4568-8e6a-ba35399bd189/706e1a4a-6f98-473e-8e7a-fe0e679a1fe3/i3asmch_thumbnail.png)
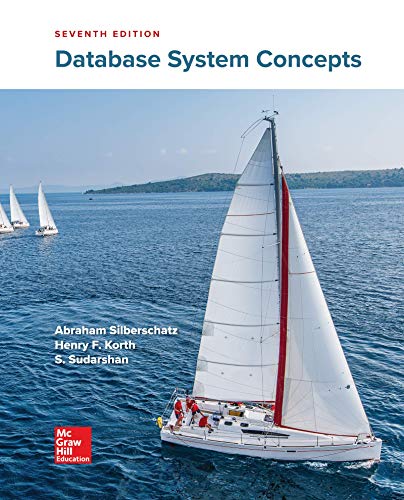
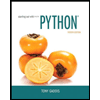
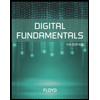
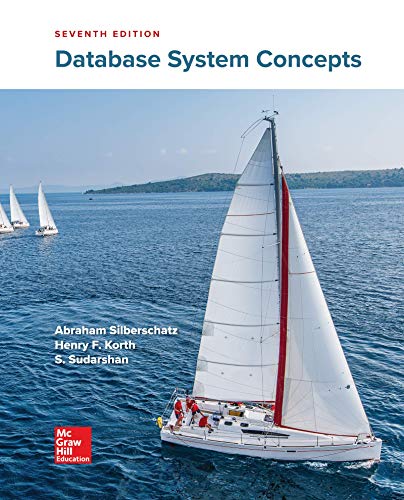
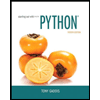
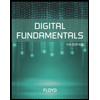
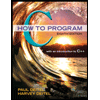
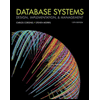
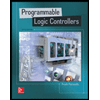