I keep getting an error on the lines: private static String pad (String str, int n) { if (str.length() > n) return str.substring (0, n); while (str.length() < n) str += " "; return str; } The program says that there is an exception but I cannot figure out where it is occuring within these lines. Can you please help me with this? Thanks so much.
I have provided the full code for my issue; I am getting an exception error that I do not understand.
import java.util.Scanner;
class Part {
private int number;
private String name;
private int quantity;
///////////////////
public Part(int number, String name, int quantity) {
this.number = number;
this.name = name;
this.quantity = quantity;
}
//////////////////////
public Part(int number, String name){}
public Part() {
}
public void decreaseQuantity (int amount) {
quantity -= amount;
}
/////////////////////////
public String getName(){
return name;
}
////////////////////
public int getNumber () {
return number;
}
/////////////////////
public int getQuantity () {
return quantity;
}
/////////////////////
public void increaseQuantity(int amount) {
quantity += amount;
}
/////////////////////////
public String toString() {
return pad (number + " ", 5) + " " + pad(name, 20) + " " +
quantity;
}
///////////////////////////
private static String pad (String str, int n) {
if (str.length() > n)
return str.substring (0, n);
while (str.length() < n)
str += " ";
return str;
}
// Provided for testing purposes only:
public static void main (String [] args) {
Scanner scan = new Scanner (System.in);
// Prompt user to enter a part:
System.out.println("Enter part number: ");
int number = scan.nextInt();
System.out.println("Enter part name: ");
String name = scan.next();
System.out.println("Enter quantity: ");
int quantity = scan.nextInt();
// Test constructors:
Part part = new Part (number, name, quantity);
System.out.println("\nPart using first constructor: " + part);
System.out.println("Part using second constructor: " + new Part(number, name));
System.out.println("Part using no-arg constructor: " + new Part());
// Test getters:
System.out.println("\nNumber: " + part.getNumber());
System.out.println("Name: " + part.getName());
System.out.println("Quantity: " + part.getQuantity());
System.out.println("\nEnter amount of increase: ");
int amount = scan.nextInt();
part.increaseQuantity(amount);
System.out.println("Part is now: " + part);
System.out.println("\nEnter amount of increase: ");
amount = scan.nextInt();
part.decreaseQuantity(amount);
System.out.println("Part is now: " + part);
} // End of main
} // End of class
I keep getting an error on the lines:
private static String pad (String str, int n) {
if (str.length() > n)
return str.substring (0, n);
while (str.length() < n)
str += " ";
return str;
}
The program says that there is an exception but I cannot figure out where it is occuring within these lines. Can you please help me with this? Thanks so much.

Step by step
Solved in 2 steps

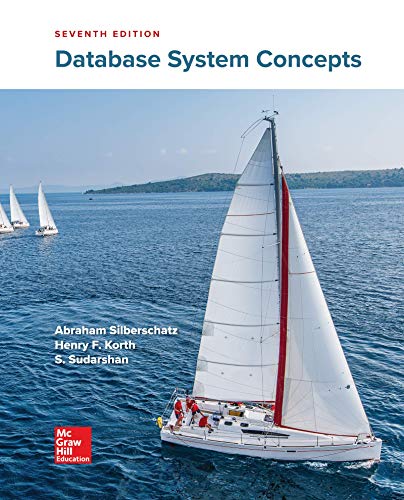
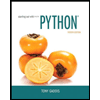
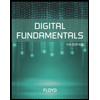
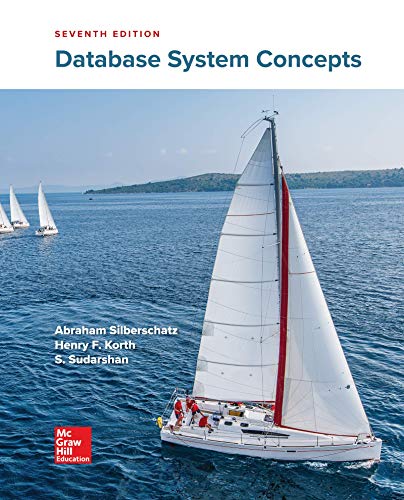
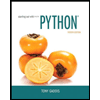
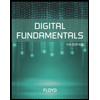
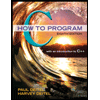
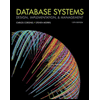
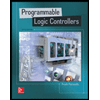