I just need an explanation as to how this program works, particularly at
I just need an explanation as to how this program works, particularly at
"string* lastnames = new string[students];
char* grades = new char[students]; " or the sort function for example.
, nothing needs to be added in it since it's complete and working.
The program is supposed to work as an average calculator for student's exams in which it takes student's last names, re-organizes them alphabetically, takes three of their scores finds the average and assigns a letter grade according to the average.
Program
#include<iostream>
#include<string>
using namespace std;
char getGrade(double average){
if(average>=90)return 'A';
else if(average>=80)return 'B';
else if(average>=70)return 'C';
else if(average>=60)return 'D';
else return 'F';
}
void sort(string lastnames[] , char grades[], int n){
for(int i=0;i<n;i++){
for(int j=0; j<n-i-1;j++){
if(lastnames[j].compare(lastnames[j+1])>0){
string temp = lastnames[j];lastnames[j]=lastnames[j+1];lastnames[j+1]=temp;
char t = grades[j];grades[j]=grades[j+1];grades[j+1]=t;
}
}
}
}
int main(){
int students; int s1,s2,s3;
double average;
cout<<"This is an automatic grade calculator. Please enter the number of students in the class:\n";
cin >> students;
string* lastnames = new string[students];
char* grades = new char[students];
cout<<"Enter each student’s last name, followed by their 3 test scores:\n";
for(int i=0; i<students;i++){
cin >> *(lastnames+i);
cin >> s1 >> s2 >> s3;
average = (s1+s2+s3)/3;
*(grades+i) = getGrade(average);
}
sort(lastnames,grades,students);
cout<<"Thanks. Here is the final grade sheet:\n";
for(int i=0; i<students;i++){
cout<<*(lastnames+i) <<" " << *(grades+i) << endl;
}
return 0;
}


Step by step
Solved in 2 steps

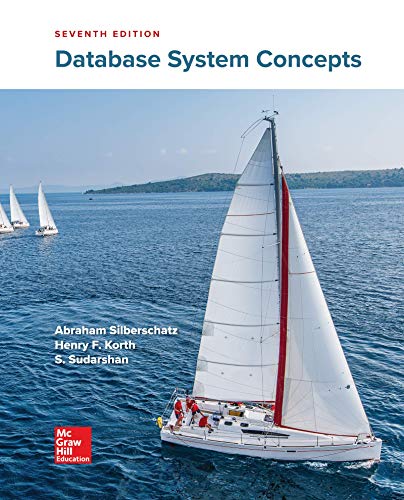
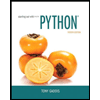
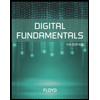
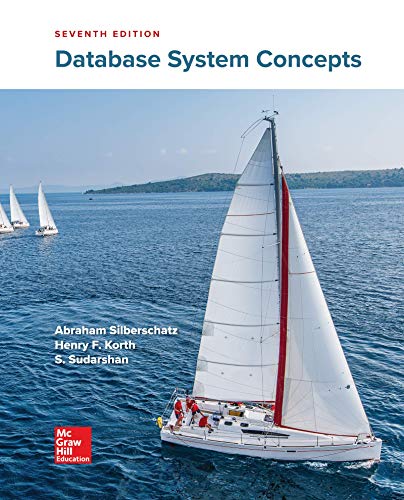
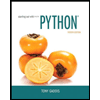
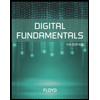
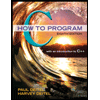
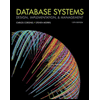
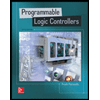