I have to modify my payroll program, written in C++, in order to accept interactive input for salary and the number of dependents. My code for the original payroll program is below. // This program calculates an employee's take-home pay. #include using namespace std; int main() { // first three lines are to declare constants for state tax, federal tax, and dependent deduction rate const double STATE_TAX = 6.5; const double FEDERAL_TAX = 28.0; const double DEPENDENTS_DEDUCTION = 2.5; double salary = 1250.00; double stateTax; double federalTax; double numDependents = 2; double dependentDeduction; double totalWithholding; double takeHomePay; // Calculate state tax here stateTax = salary * (STATE_TAX / 100); cout << "State Tax: $" << stateTax << endl; // Calculate federal tax here federalTax = salary * (FEDERAL_TAX / 100); cout << "Federal Tax: $" << federalTax << endl; // Calculate dependent deduction here dependentDeduction = salary * (DEPENDENTS_DEDUCTION / 100) * numDependents; cout << "Dependents: $" << dependentDeduction << endl; // Calculate total withholding here totalWithholding = stateTax + federalTax; // Calculate take-home pay here takeHomePay = salary - totalWithholding + dependentDeduction; cout << "Salary: $" << salary << endl; cout << "Take-Home Pay: $" << takeHomePay << endl; return 0; }
I have to modify my payroll
// This program calculates an employee's take-home pay.
#include <iostream>
using namespace std;
int main()
{
// first three lines are to declare constants for state tax, federal tax, and dependent deduction rate
const double STATE_TAX = 6.5;
const double FEDERAL_TAX = 28.0;
const double DEPENDENTS_DEDUCTION = 2.5;
double salary = 1250.00;
double stateTax;
double federalTax;
double numDependents = 2;
double dependentDeduction;
double totalWithholding;
double takeHomePay;
// Calculate state tax here
stateTax = salary * (STATE_TAX / 100);
cout << "State Tax: $" << stateTax << endl;
// Calculate federal tax here
federalTax = salary * (FEDERAL_TAX / 100);
cout << "Federal Tax: $" << federalTax << endl;
// Calculate dependent deduction here
dependentDeduction = salary * (DEPENDENTS_DEDUCTION / 100) * numDependents;
cout << "Dependents: $" << dependentDeduction << endl;
// Calculate total withholding here
totalWithholding = stateTax + federalTax;
// Calculate take-home pay here
takeHomePay = salary - totalWithholding + dependentDeduction;
cout << "Salary: $" << salary << endl;
cout << "Take-Home Pay: $" << takeHomePay << endl;
return 0;
}

Step by step
Solved in 4 steps with 6 images

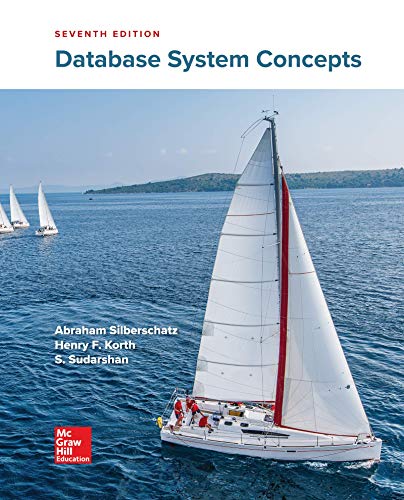
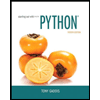
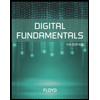
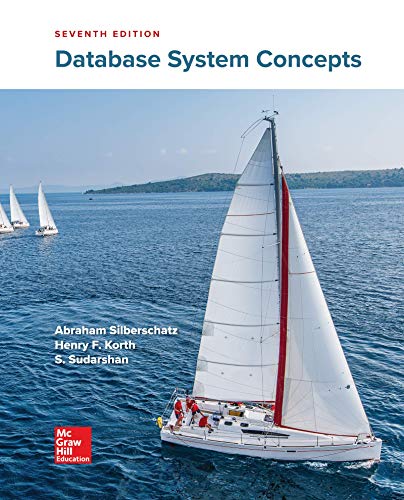
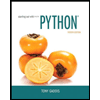
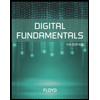
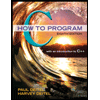
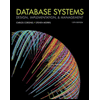
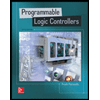